【数据结构与算法可视化】
发布时间: 2024-09-01 05:25:58 阅读量: 182 订阅数: 135 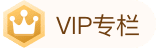
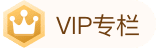
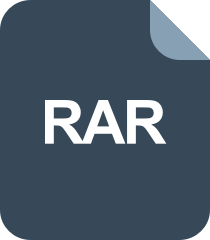
数据结构课程设计,算法可视化

# 1. 数据结构与算法可视化概述
数据结构与算法是计算机科学的基石,它们在软件开发、算法设计以及系统优化等方面发挥着至关重要的作用。然而,这些抽象概念对于初学者来说往往难以快速掌握,而可视化技术则提供了一种直观易懂的理解方式。在本章中,我们将介绍数据结构与算法可视化的基本概念,探讨其重要性和应用范围,并概述如何利用可视化工具和方法来提高理解和学习效率。
数据结构是数据的组织方式,决定了数据的存储和操作效率。算法则是解决问题的一系列步骤。当这两者结合可视化技术后,复杂的概念和过程就变得易于观察和理解。例如,算法执行过程中的变量变化、数据结构内部节点的连接关系等,都可以通过图形界面直观展现。
可视化不仅可以帮助开发者和学习者更好地理解数据结构和算法,而且对于优化和改进现有算法也具有重要意义。通过动态展示,我们可以更清楚地看到算法在不同情况下的表现,识别性能瓶颈,从而对算法进行针对性优化。
在后续章节中,我们将进一步探讨各种数据结构的可视化方法,介绍常见的算法可视化技术,并通过案例分析展示如何在实际工作中应用这些可视化工具和技术。
# 2. 基础数据结构可视化
数据结构是计算机存储、组织数据的方式,使得数据的操作更加高效。通过可视化手段来展示这些数据结构,可以让学习者更直观地理解其操作过程和内部逻辑。本章将详细介绍基础数据结构的可视化表现,涵盖线性结构、树形结构和图结构的可视化,以及它们的具体操作和表现方法。
## 2.1 线性结构的可视化
线性结构是最基础的数据结构之一,包括数组、链表、栈和队列等,其元素在逻辑上是一条线,具有一个首元素和一个尾元素。
### 2.1.1 数组和链表的表示与操作
数组和链表是两种常见的线性数据结构。数组是用连续的内存空间存储相同类型数据的线性结构,而链表是通过指针连接一系列不连续的内存空间。
#### 表格:数组和链表特性比较
| 特性 | 数组 | 链表 |
| --- | --- | --- |
| 存储方式 | 连续的内存空间 | 不连续的内存空间 |
| 空间分配 | 静态分配或动态分配 | 动态分配 |
| 索引访问 | 支持随机访问,时间复杂度为O(1) | 不支持随机访问,时间复杂度为O(n) |
| 插入和删除操作 | 时间复杂度为O(n),移动元素效率低 | 时间复杂度为O(1),通过指针调整效率高 |
#### 代码块示例:数组和链表的插入操作
```python
# 数组插入示例
def array_insert(array, index, element):
array.insert(index, element)
return array
# 链表插入示例
class ListNode:
def __init__(self, value=0, next_node=None):
self.value = value
self.next = next_node
def linked_list_insert(head, index, element):
new_node = ListNode(element)
if index == 0:
new_node.next = head
return new_node
current = head
position = 0
while current.next and position < index - 1:
current = current.next
position += 1
new_node.next = current.next
current.next = new_node
return head
# 示例
arr = [1, 2, 3]
linked_list = ListNode(1, ListNode(2, ListNode(3)))
arr = array_insert(arr, 1, 4)
linked_list = linked_list_insert(linked_list, 1, 4)
```
在这个示例中,`array_insert`函数用于在数组中插入一个元素,而`linked_list_insert`函数用于在链表中插入一个元素。数组的插入需要移动后续的元素,因此效率较低,特别是在数组较大时。链表的插入只需要调整相关节点的指针,因此效率较高。
### 2.1.2 栈和队列的入栈出栈行为
栈和队列是两种特殊的线性数据结构,它们的操作有一定的限制:栈是一种后进先出(LIFO)的数据结构,支持元素的压入(push)和弹出(pop)操作;队列是一种先进先出(FIFO)的数据结构,支持元素的入队(enqueue)和出队(dequeue)操作。
#### mermaid流程图:栈的入栈出栈过程
```mermaid
flowchart LR
A[Start] --> B[Push element 1]
B --> C[Push element 2]
C --> D[Push element 3]
D --> E[Pop]
E --> F[End]
```
在上述流程图中,展示了栈中元素入栈(push)和出栈(pop)的顺序。首先,三个元素依次入栈,然后依次出栈。
#### 代码块示例:栈的实现和操作
```python
# 栈的实现和操作
class Stack:
def __init__(self):
self.items = []
def is_empty(self):
return self.items == []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
return self.items[-1] if self.items else None
# 使用栈进行操作
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # 输出: 3
print(stack.peek()) # 输出: 2
```
这段代码展示了如何使用Python实现一个简单的栈数据结构,以及如何进行入栈(push)和出栈(pop)操作。`Stack`类使用Python内置列表`items`来存储栈内的元素。`push`方法将元素添加到栈顶,`pop`方法移除栈顶元素并返回它。
## 2.2 树形结构的可视化
树形结构是一种分层的数据结构,它模拟了自然界中树的结构。树形结构在数据库系统、文件系统和网络路由等计算机领域应用广泛。
### 2.2.1 二叉树的遍历过程
二叉树是树形结构中的一种特殊形式,每个节点最多有两个子节点,分别是左子节点和右子节点。二叉树的遍历分为前序、中序和后序三种基本方式。
#### 表格:二叉树遍历方式比较
| 遍历方式 | 访问顺序 | 应用场景 |
| --- | --- | --- |
| 前序遍历 | 根 -> 左 -> 右 | 复制二叉树、打印表达式树的值 |
| 中序遍历 | 左 -> 根 -> 右 | 二叉搜索树的中序遍历输出有序数据 |
| 后序遍历 | 左 -> 右 -> 根 | 在删除二叉树时释放内存 |
#### 代码块示例:二叉树的前序遍历
```python
class TreeNode:
def __init__(self, x):
self.val = x
self.left = None
self.right = None
def preorder_traversal(root):
if root is None:
return []
return [root.val] + preorder_traversal(root.left) + preorder_traversal(root.right)
# 构建一个简单的二叉树
# 1
# / \
# 2 3
# / \
# 4 5
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
# 执行前序遍历
print(preorder_traversal(root)) # 输出: [1, 2, 4, 5, 3]
```
在这段代码中,定义了一个二叉树节点`TreeNode`,以及一个递归函数`preorder_traversal`来执行前序遍历。遍历结果表明,在访问任何节点之前,都会首先访问其根节点,然后是左子节点,最后是右子节点。
### 2.2.2 堆结构及其调整
堆是一种特殊的完全二叉树,其中每个父节点的值都大于或等于其子节点的值。堆常用于实现优先队列。
#### mermaid流程图:堆结构调整过程
```mermaid
flowchart TD
A[Start] --> B[Adjust Heap]
B --> C[Swap parent with larger child]
C --> D[Is Heap Condition Satisfied?]
D -- Yes --> E[
```
0
0
相关推荐
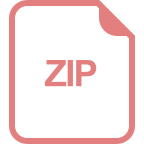
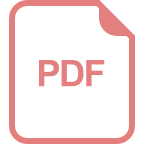
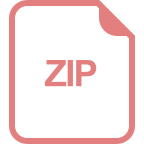
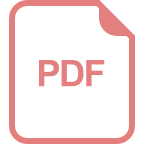
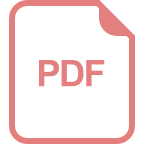
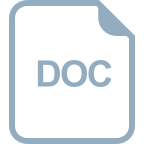
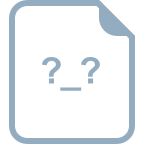