S800板用I2C0中断读入SW1~SW8的值
时间: 2023-07-05 20:29:49 浏览: 204
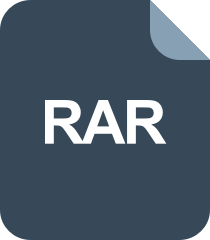
嵌入式原理S800实验板相关资料
S800板使用I2C0进行读取SW1~SW8的值,可以使用以下代码实现:
```c
#include "inc/hw_memmap.h"
#include "inc/hw_types.h"
#include "driverlib/gpio.h"
#include "driverlib/i2c.h"
#include "driverlib/pin_map.h"
#include "driverlib/sysctl.h"
#define I2C_SLAVE_ADDR 0x20
void initI2C0(void)
{
// 使能I2C0和GPIOB模块的时钟
SysCtlPeripheralEnable(SYSCTL_PERIPH_I2C0);
SysCtlPeripheralEnable(SYSCTL_PERIPH_GPIOB);
// 配置PB2和PB3引脚作为I2C0的SDA和SCL信号线
GPIOPinConfigure(GPIO_PB2_I2C0SCL);
GPIOPinConfigure(GPIO_PB3_I2C0SDA);
GPIOPinTypeI2C(GPIO_PORTB_BASE, GPIO_PIN_2 | GPIO_PIN_3);
// 配置I2C0的工作模式
I2CMasterInitExpClk(I2C0_BASE, SysCtlClockGet(), true);
// 配置I2C0的中断
I2CMasterIntEnable(I2C0_BASE);
IntEnable(INT_I2C0);
// 设置I2C0的从设备地址
I2CMasterSlaveAddrSet(I2C0_BASE, I2C_SLAVE_ADDR, false);
}
void readSwitches(void)
{
uint8_t data[1];
// 发送读取SW1~SW8的命令
data[0] = 0x00;
I2CMasterDataPut(I2C0_BASE, data[0]);
I2CMasterControl(I2C0_BASE, I2C_MASTER_CMD_SINGLE_SEND);
while(I2CMasterBusy(I2C0_BASE));
// 读取SW1~SW8的值
I2CMasterSlaveAddrSet(I2C0_BASE, I2C_SLAVE_ADDR, true);
I2CMasterControl(I2C0_BASE, I2C_MASTER_CMD_BURST_RECEIVE_START);
while(I2CMasterBusy(I2C0_BASE));
data[0] = I2CMasterDataGet(I2C0_BASE);
for(int i = 0; i < 7; i++)
{
I2CMasterControl(I2C0_BASE, I2C_MASTER_CMD_BURST_RECEIVE_CONT);
while(I2CMasterBusy(I2C0_BASE));
data[0] = I2CMasterDataGet(I2C0_BASE);
}
I2CMasterControl(I2C0_BASE, I2C_MASTER_CMD_BURST_RECEIVE_FINISH);
while(I2CMasterBusy(I2C0_BASE));
data[0] = I2CMasterDataGet(I2C0_BASE);
// 输出SW1~SW8的值
for(int i = 7; i >= 0; i--)
{
if(data[0] & (1 << i))
{
printf("SW%d is ON\n", i + 1);
}
else
{
printf("SW%d is OFF\n", i + 1);
}
}
}
void I2C0IntHandler(void)
{
uint32_t intStatus = I2CMasterIntStatus(I2C0_BASE);
I2CMasterIntClear(I2C0_BASE, intStatus);
}
int main(void)
{
// 初始化系统时钟和UART
SysCtlClockSet(SYSCTL_SYSDIV_4 | SYSCTL_USE_PLL | SYSCTL_XTAL_16MHZ | SYSCTL_OSC_MAIN);
initUART();
// 初始化I2C0
initI2C0();
while(1)
{
// 读取SW1~SW8的值
readSwitches();
// 延时一段时间
SysCtlDelay(SysCtlClockGet() / 3);
}
}
```
在该代码中,我们首先定义了I2C从设备的地址为0x20,然后在initI2C0()函数中配置了I2C0的工作模式和中断。在readSwitches()函数中,我们首先向I2C从设备发送了一个命令,以读取SW1~SW8的值,然后使用I2C_MASTER_CMD_BURST_RECEIVE_START命令开始读取数据,并输出SW1~SW8的值。在I2C0IntHandler()函数中,我们处理了I2C0的中断。最后,在main()函数中,我们循环读取SW1~SW8的值,并延时一段时间。
阅读全文
相关推荐
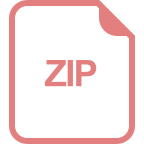
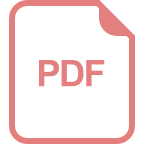
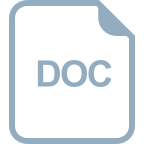
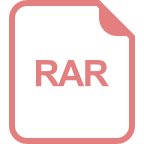
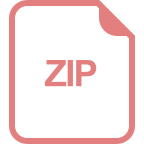
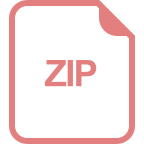
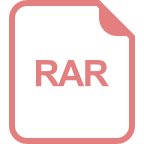
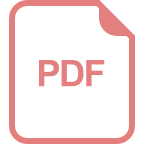
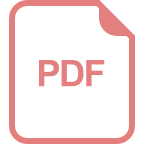
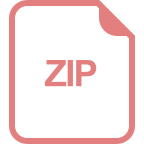
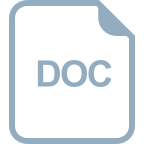
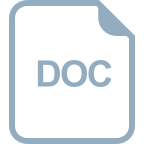
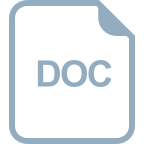
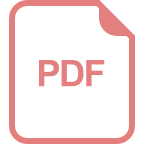