请用python写一段canny边缘检测代码
时间: 2023-07-22 17:00:22 浏览: 117
当然,下面是一个使用Python和OpenCV库实现Canny边缘检测的示例代码:
```python
import cv2
def canny_edge_detection(image_path):
# 读取图像
image = cv2.imread(image_path, 0)
# 进行Canny边缘检测
edges = cv2.Canny(image, 50, 150)
# 显示原始图像和边缘检测结果
cv2.imshow("Original Image", image)
cv2.imshow("Canny Edge Detection", edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 测试代码
image_path = "image.jpg"
canny_edge_detection(image_path)
```
这段代码首先使用`cv2.imread`函数读取指定路径的图像,将其转换为灰度图像。然后,使用`cv2.Canny`函数进行Canny边缘检测,传入图像和阈值参数。阈值1和阈值2用于控制边缘的强度,具体取值可以根据需求进行调整。
最后,使用`cv2.imshow`函数显示原始图像和Canny边缘检测结果,并使用`cv2.waitKey`等待用户按下任意键关闭窗口。
请确保您已经安装了OpenCV库,并将图像文件命名为"image.jpg"并与代码放在同一目录下。
相关问题
用python写一段Canny算子的边缘检测程序
好的,以下是用Python实现Canny算子的边缘检测程序:
```python
import cv2
# 读取图像
img = cv2.imread('test.jpg', cv2.IMREAD_GRAYSCALE)
# Canny算子边缘检测
edges = cv2.Canny(img, 100, 200)
# 显示结果
cv2.imshow('output', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`cv2.imread()`函数用来读取图像,`cv2.IMREAD_GRAYSCALE`表示以灰度模式读取。
`cv2.Canny()`函数用来进行Canny算子边缘检测,其中第二个和第三个参数分别为低阈值和高阈值,可以根据具体需求进行调整。
最后,使用`cv2.imshow()`函数显示结果,`cv2.waitKey()`等待用户按键关闭窗口,`cv2.destroyAllWindows()`释放窗口。
用python代码写一个canny边缘检测算法
以下是用 Python 代码实现 Canny 边缘检测算法的示例:
```python
import cv2
import numpy as np
def canny_edge_detection(image, sigma=0.33):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Compute the median of the single channel pixel intensities
median = np.median(gray)
# Calculate the lower and upper thresholds using the median
lower = int(max(0, (1.0 - sigma) * median))
upper = int(min(255, (1.0 + sigma) * median))
# Apply Canny edge detection using the computed thresholds
edges = cv2.Canny(gray, lower, upper)
return edges
# Load an image
image = cv2.imread('image.jpg')
# Apply Canny edge detection
edges = canny_edge_detection(image)
# Display the original image and the detected edges
cv2.imshow('Original Image', image)
cv2.imshow('Canny Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码使用 OpenCV 库实现了 Canny 边缘检测算法,可以对一张图片进行边缘检测并显示结果。
阅读全文
相关推荐
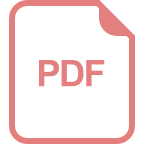
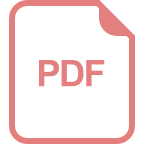
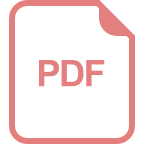
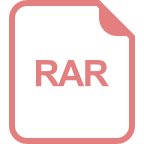
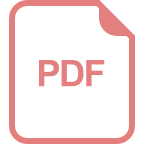
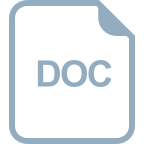
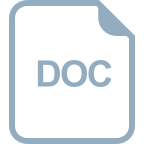
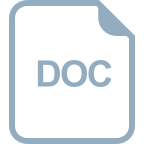
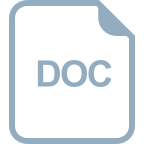
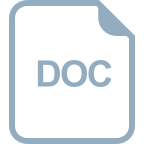





