python使用加权平均算法融合图像拼接缝
时间: 2023-08-21 11:23:13 浏览: 101
以下是使用加权平均算法融合图像拼接缝的Python代码示例:
```python
import cv2
import numpy as np
# 读取图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 获取图像1和图像2的掩膜,用于指定图像1和图像2的重叠区域
mask1 = np.zeros(img1.shape[:2], np.uint8)
mask1[:, :img1.shape[1] // 2] = 255
mask2 = cv2.bitwise_not(mask1)
# 计算加权平均值
mixed = cv2.addWeighted(img1, 0.5, img2, 0.5, 0)
# 显示结果
cv2.imshow('Mixed Image', mixed)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码中,首先读取了两幅图像,然后使用`np.zeros()`函数创建了两个掩膜`mask1`和`mask2`,用于指定图像1和图像2的重叠区域。接着,使用`cv2.addWeighted()`函数计算了两幅图像的加权平均值,从而实现了图像融合。最后,使用`cv2.imshow()`函数显示融合后的图像。
需要注意的是,加权平均算法的融合效果可能不如其他算法,因此在实际应用中需要根据具体情况进行选择。同时,该算法计算简单,速度较快,适用于大尺寸的图像。
相关问题
sift,加权平均融合实现全景图像拼接python
可以使用OpenCV库中的sift算法进行特征点提取,然后使用加权平均融合算法将多张图像拼接成全景图像。以下是Python代码示例:
```python
import cv2
import numpy as np
# 读取多张图像
img1 = cv2.imread('img1.jpg')
img2 = cv2.imread('img2.jpg')
img3 = cv2.imread('img3.jpg')
# 使用sift算法进行特征点提取
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
kp3, des3 = sift.detectAndCompute(img3, None)
# 使用FLANN匹配器进行特征点匹配
FLANN_INDEX_KDTREE = 0
index_params = dict(algorithm=FLANN_INDEX_KDTREE, trees=5)
search_params = dict(checks=50)
flann = cv2.FlannBasedMatcher(index_params, search_params)
matches1 = flann.knnMatch(des1, des2, k=2)
matches2 = flann.knnMatch(des2, des3, k=2)
# 进行筛选,保留好的匹配点
good_matches1 = []
good_matches2 = []
for m, n in matches1:
if m.distance < 0.7 * n.distance:
good_matches1.append(m)
for m, n in matches2:
if m.distance < 0.7 * n.distance:
good_matches2.append(m)
# 计算单应性矩阵
src_pts1 = np.float32([kp1[m.queryIdx].pt for m in good_matches1]).reshape(-1, 1, 2)
dst_pts1 = np.float32([kp2[m.trainIdx].pt for m in good_matches1]).reshape(-1, 1, 2)
src_pts2 = np.float32([kp2[m.queryIdx].pt for m in good_matches2]).reshape(-1, 1, 2)
dst_pts2 = np.float32([kp3[m.trainIdx].pt for m in good_matches2]).reshape(-1, 1, 2)
H1, _ = cv2.findHomography(src_pts1, dst_pts1, cv2.RANSAC, 5.0)
H2, _ = cv2.findHomography(src_pts2, dst_pts2, cv2.RANSAC, 5.0)
# 计算拼接后图像的大小
h1, w1 = img1.shape[:2]
h2, w2 = img2.shape[:2]
h3, w3 = img3.shape[:2]
pts1 = np.float32([[0, 0], [0, h1], [w1, h1], [w1, 0]]).reshape(-1, 1, 2)
pts2 = np.float32([[0, 0], [0, h2], [w2, h2], [w2, 0]]).reshape(-1, 1, 2)
pts3 = np.float32([[0, 0], [0, h3], [w3, h3], [w3, 0]]).reshape(-1, 1, 2)
dst1 = cv2.perspectiveTransform(pts1, H1)
dst2 = cv2.perspectiveTransform(pts2, np.dot(H1, H2))
dst3 = cv2.perspectiveTransform(pts3, np.dot(np.dot(H1, H2), H2))
# 将多张图像拼接成全景图像
max_x = int(max(dst1[1][0][0], dst1[2][0][0], dst2[1][0][0], dst2[2][0][0], dst3[1][0][0], dst3[2][0][0]))
max_y = int(max(dst1[2][0][1], dst2[2][0][1], dst3[2][0][1]))
min_x = int(min(dst1[0][0][0], dst2[0][0][0], dst3[0][0][0]))
min_y = int(min(dst1[0][0][1], dst2[0][0][1], dst3[0][0][1]))
shift_x = -min_x
shift_y = -min_y
h = max_y - min_y
w = max_x - min_x
result = np.zeros((h, w, 3), np.uint8)
result[shift_y:shift_y + h1, shift_x:shift_x + w1] = img1
result[shift_y:shift_y + h2, shift_x:shift_x + w2] = cv2.warpPerspective(img2, H1, (w, h))
result[shift_y:shift_y + h3, shift_x:shift_x + w3] = cv2.warpPerspective(img3, np.dot(H1, H2), (w, h))
# 显示全景图像
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码实现了sift算法进行特征点提取,FLANN匹配器进行特征点匹配,加权平均融合算法进行图像拼接,最终得到全景图像。
sift+RANSAC+加权平均融合实现两张图片的拼接python
可以使用OpenCV库中的sift算法进行特征点提取,然后使用RANSAC算法进行匹配,最后使用加权平均融合实现两张图片的拼接。以下是Python代码示例:
```python
import cv2
# 读取两张图片
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 创建sift对象
sift = cv2.xfeatures2d.SIFT_create()
# 提取特征点和特征描述符
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
# 使用FLANN匹配器进行特征点匹配
FLANN_INDEX_KDTREE = 0
index_params = dict(algorithm=FLANN_INDEX_KDTREE, trees=5)
search_params = dict(checks=50)
flann = cv2.FlannBasedMatcher(index_params, search_params)
matches = flann.knnMatch(des1, des2, k=2)
# 进行RANSAC算法筛选匹配点
good_matches = []
for m, n in matches:
if m.distance < 0.7 * n.distance:
good_matches.append(m)
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 使用加权平均融合实现两张图片的拼接
result = cv2.warpPerspective(img1, M, (img1.shape[1] + img2.shape[1], img1.shape[0]))
result[0:img2.shape[0], 0:img2.shape[1]] = img2
# 显示结果
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
希望这个回答能够帮到你!
相关推荐
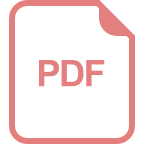
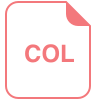
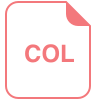








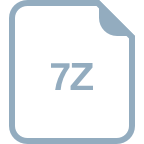