设计一个算法,求两个单链表表示的集合的交集,冰机那个结果用一个新的单链表保存并返回,用c语言代码表示
时间: 2023-03-31 18:03:02 浏览: 136
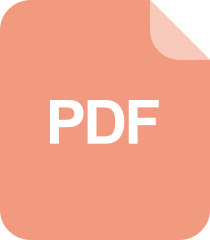
海淡水两用制冰机的总体方案和热力循环设计.pdf
可以使用哈希表来实现求两个单链表的交集,具体步骤如下:
1. 定义一个哈希表,用于存储链表1中的元素。
2. 遍历链表1,将每个元素插入到哈希表中。
3. 遍历链表2,对于每个元素,判断其是否在哈希表中出现过,如果出现过,则将其加入到结果链表中。
4. 返回结果链表。
以下是C语言代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#define HASH_SIZE 100
// 定义链表节点
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
// 定义哈希表节点
typedef struct HashNode {
int key;
struct HashNode *next;
} HashNode;
// 哈希函数
int hash(int key) {
return key % HASH_SIZE;
}
// 插入哈希表
void insert(HashNode **hashTable, int key) {
int index = hash(key);
HashNode *node = (HashNode *)malloc(sizeof(HashNode));
node->key = key;
node->next = hashTable[index];
hashTable[index] = node;
}
// 查找哈希表
int find(HashNode **hashTable, int key) {
int index = hash(key);
HashNode *node = hashTable[index];
while (node != NULL) {
if (node->key == key) {
return 1;
}
node = node->next;
}
return ;
}
// 求两个单链表的交集
ListNode* getIntersectionNode(ListNode* headA, ListNode* headB) {
HashNode *hashTable[HASH_SIZE] = {NULL};
ListNode *p = headA;
while (p != NULL) {
insert(hashTable, p->val);
p = p->next;
}
ListNode *q = headB;
ListNode *result = NULL;
while (q != NULL) {
if (find(hashTable, q->val)) {
ListNode *node = (ListNode *)malloc(sizeof(ListNode));
node->val = q->val;
node->next = result;
result = node;
}
q = q->next;
}
return result;
}
// 测试
int main() {
ListNode *headA = (ListNode *)malloc(sizeof(ListNode));
headA->val = 1;
headA->next = (ListNode *)malloc(sizeof(ListNode));
headA->next->val = 2;
headA->next->next = (ListNode *)malloc(sizeof(ListNode));
headA->next->next->val = 3;
headA->next->next->next = NULL;
ListNode *headB = (ListNode *)malloc(sizeof(ListNode));
headB->val = 2;
headB->next = (ListNode *)malloc(sizeof(ListNode));
headB->next->val = 3;
headB->next->next = (ListNode *)malloc(sizeof(ListNode));
headB->next->next->val = 4;
headB->next->next->next = NULL;
ListNode *result = getIntersectionNode(headA, headB);
while (result != NULL) {
printf("%d ", result->val);
result = result->next;
}
printf("\n");
return ;
}
```
阅读全文
相关推荐
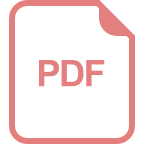
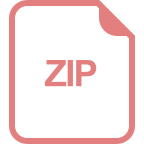







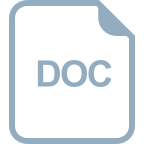
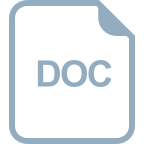
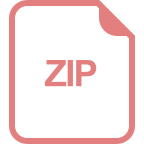
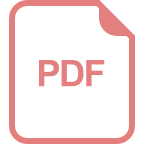
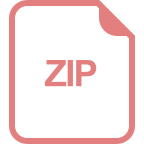
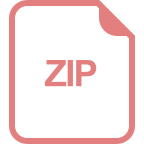
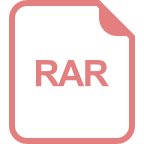
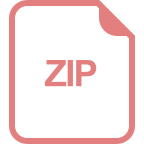