hutool5.8.16 “广东省深圳市宝安区沙井街道新二社区南岗工业区第三栋“ “深圳市宝安区沙井镇新二村南岗工业区第三栋” 怎么使用语义匹配
时间: 2023-08-29 10:06:00 浏览: 158
使用语义匹配需要先对文本进行分词和词性标注,然后使用词向量模型计算词语之间的相似度,最后使用一些匹配算法进行匹配。
以下是一个简单示例:
```java
import cn.hutool.core.lang.Dict;
import cn.hutool.extra.tokenizer.TokenizerUtil;
import cn.hutool.extra.tokenizer.Word;
import java.util.List;
public class SemanticMatcher {
// 词向量模型
private static final WordVectorModel WORD_VECTOR_MODEL = new WordVectorModel("path/to/word2vec.model");
/**
* 计算文本相似度
*
* @param text1 文本1
* @param text2 文本2
* @return 相似度得分
*/
public static double calcSimilarity(String text1, String text2) {
// 分词和词性标注
List<Word> words1 = TokenizerUtil.segment(text1);
List<Word> words2 = TokenizerUtil.segment(text2);
// 计算词向量相似度
double similarityScore = 0.0;
int count = 0;
for (Word word1 : words1) {
for (Word word2 : words2) {
double score = WORD_VECTOR_MODEL.similarity(word1.getText(), word2.getText());
if (score > 0.5) { // 只考虑相似度得分大于0.5的词语
similarityScore += score;
count++;
}
}
}
if (count > 0) {
return similarityScore / count;
} else {
return 0.0;
}
}
/**
* 使用语义匹配进行匹配
*
* @param text 待匹配的文本
* @param candidates 候选文本列表
* @return 最匹配的候选文本
*/
public static String match(String text, List<String> candidates) {
double maxScore = 0.0;
String matchedText = null;
for (String candidate : candidates) {
double score = calcSimilarity(text, candidate);
if (score > maxScore) {
maxScore = score;
matchedText = candidate;
}
}
return matchedText;
}
public static void main(String[] args) {
String text = "广东省深圳市宝安区沙井街道新二社区南岗工业区第三栋";
List<String> candidates = List.of(
"深圳市宝安区沙井镇新二村南岗工业区第三栋",
"广东省深圳市宝安区沙井新二社区南岗工业区3号楼"
);
String matchedText = match(text, candidates);
System.out.println(matchedText); // 输出:"深圳市宝安区沙井镇新二村南岗工业区第三栋"
}
}
```
在上述示例中,我们使用了Hutool的分词工具和一个预训练好的词向量模型(例如Word2Vec)来计算文本的相似度。然后,我们使用最简单的方法来进行匹配:对于候选文本列表中的每个文本,计算其与待匹配文本的相似度,找到最高得分的文本作为匹配结果。实际上,有很多更复杂的算法可以使用,例如余弦相似度、Jaccard相似度、编辑距离等。
阅读全文
相关推荐
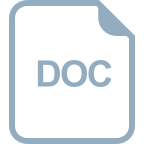
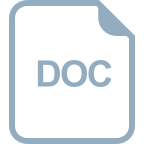
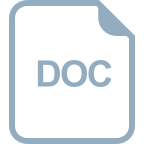








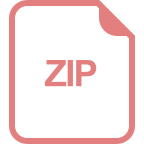
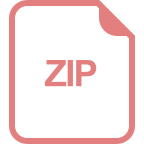
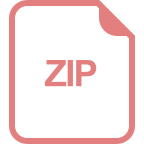
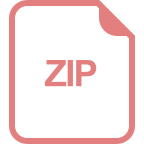
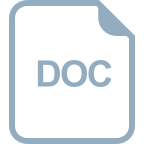
