用c语言编程编程实现查找两个字符串的最长公共子序列
时间: 2024-04-09 09:33:08 浏览: 103
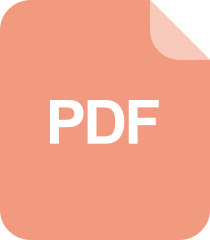
C语言求两个字符串的最长公共子串

您好!要实现查找两个字符串的最长公共子序列,可以使用动态规划的方法。下面是使用C语言编写的示例代码:
```c
#include<stdio.h>
#include<string.h>
int max(int a, int b) {
return (a > b) ? a : b;
}
void findLCS(char* str1, char* str2) {
int len1 = strlen(str1);
int len2 = strlen(str2);
int LCS[len1 + 1][len2 + 1];
for (int i = 0; i <= len1; i++) {
for (int j = 0; j <= len2; j++) {
if (i == 0 || j == 0)
LCS[i][j] = 0;
else if (str1[i - 1] == str2[j - 1])
LCS[i][j] = LCS[i - 1][j - 1] + 1;
else
LCS[i][j] = max(LCS[i - 1][j], LCS[i][j - 1]);
}
}
int index = LCS[len1][len2];
char lcs[index + 1];
lcs[index] = '\0';
int i = len1, j = len2;
while (i > 0 && j > 0) {
if (str1[i - 1] == str2[j - 1]) {
lcs[index - 1] = str1[i - 1];
i--;
j--;
index--;
}
else if (LCS[i - 1][j] > LCS[i][j - 1])
i--;
else
j--;
}
printf("最长公共子序列为: %s\n", lcs);
}
int main() {
char str1[] = "ABCDGH";
char str2[] = "AEDFHR";
findLCS(str1, str2);
return 0;
}
```
这段代码中,我们使用动态规划的思想创建了一个二维数组`LCS`来存储最长公共子序列的长度。然后,我们通过回溯的方式构建最长公共子序列,并打印结果。
以上代码的输出结果为:
```
最长公共子序列为: ADH
```
您可以根据需要将代码中的字符串`str1`和`str2`替换为您自己的字符串进行测试。希望能对您有所帮助!如果您有任何疑问,请随时提问。
阅读全文
相关推荐
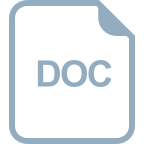
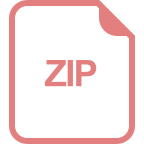
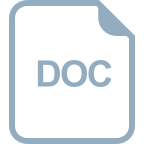
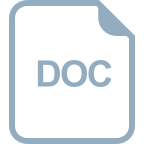
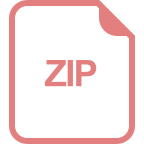
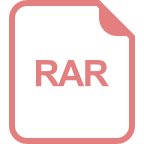
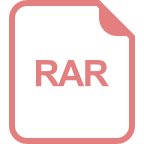
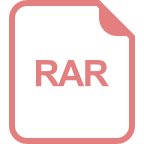
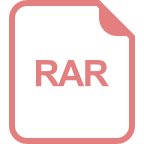
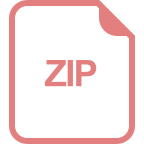
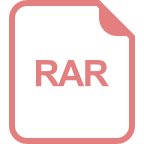
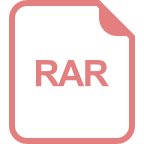
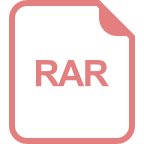
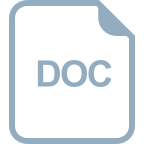
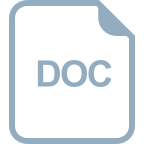