广义表的创建和打印
时间: 2023-07-25 08:18:59 浏览: 131
广义表是一种数据结构,类似于树形结构。它可以用来表示各种复杂的数据,如树、图、多项式等。广义表由一个表头和一个表尾组成,其中表头可以是任意类型的数据,表尾是一个指向另一个广义表的指针。
以下是一个简单的广义表的创建和打印的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义广义表节点的结构体
typedef struct GListNode {
int isAtom; // 是否为原子节点,0表示不是,1表示是
union {
char atom; // 原子节点的值
struct GListNode *sublist; // 子表节点的指针
} data;
struct GListNode *next;
} GListNode;
// 函数声明
GListNode *createGList(char *str);
void printGList(GListNode *list);
// 创建广义表的函数
GListNode *createGList(char *str) {
GListNode *head = NULL, *tail = NULL, *node = NULL;
int isAtom = 0;
char atom;
while (*str != '\0') {
switch (*str) {
case '(':
isAtom = 0;
node = (GListNode *) malloc(sizeof(GListNode));
node->next = NULL;
if (head == NULL) {
head = node;
} else {
tail->next = node;
}
tail = node;
break;
case ')':
node = tail;
tail = tail->next;
free(node);
break;
case ',':
isAtom = 0;
break;
default:
atom = *str;
if (*(str + 1) == ',' || *(str + 1) == ')') {
isAtom = 1;
}
node = (GListNode *) malloc(sizeof(GListNode));
node->isAtom = isAtom;
if (isAtom) {
node->data.atom = atom;
node->data.sublist = NULL;
} else {
node->data.atom = 0;
node->data.sublist = createGList(str + 1);
}
node->next = NULL;
if (head == NULL) {
head = node;
} else {
tail->next = node;
}
tail = node;
break;
}
str++;
}
return head;
}
// 打印广义表的函数
void printGList(GListNode *list) {
printf("(");
while (list != NULL) {
if (list->isAtom) {
printf("%c", list->data.atom);
} else {
printGList(list->data.sublist);
}
if (list->next != NULL) {
printf(",");
}
list = list->next;
}
printf(")");
}
// 测试
int main() {
char str[] = "(a,(b,c),d)";
GListNode *list = createGList(str);
printGList(list);
return 0;
}
```
代码中的createGList函数通过递归的方式来创建广义表,它可以接受一个字符串作为参数,返回一个指向广义表头节点的指针。printGList函数用来打印广义表。在测试代码中,我们创建了一个广义表,并用printGList函数来打印它。
输出结果为:
```
(a,(b,c),d)
```
注意,这里我们只是实现了一个简单的广义表创建和打印的功能,实际上广义表还有很多复杂的操作,如求深度、求长度、插入、删除等,需要根据需求进行实现。
阅读全文
相关推荐
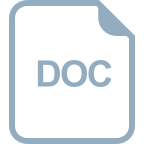
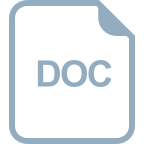
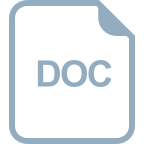



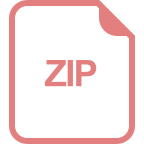
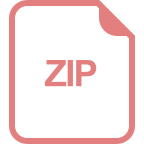
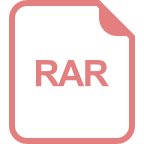
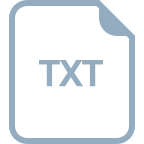
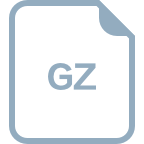
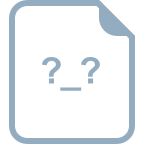
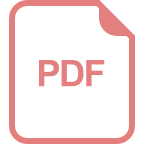
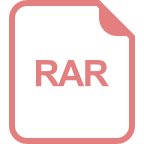
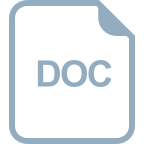
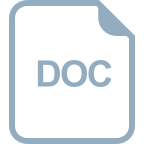
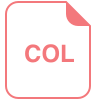

