使用C语言实现创建广义表的递归算法。
时间: 2024-01-24 21:19:11 浏览: 91
广义表可以看作是一个树状结构,其中每个节点可能是一个原子元素或者是一个子广义表。对于一个广义表,它可以被表示为一个由左括号、右括号和逗号组成的字符串。例如,广义表 (1,2,(3,4)) 可以表示为字符串 "(1,2,(3,4))"。
下面是使用递归算法创建广义表的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct node {
char data;
struct node *next;
struct node *sublist;
} Node;
// 创建子表
Node *create_sublist(char *str, int *index) {
Node *cur = NULL;
Node *head = NULL;
while (str[*index] != ')' && str[*index] != '\0') {
if (str[*index] == '(') { // 递归创建子表
(*index)++;
Node *sublist = create_sublist(str, index);
if (cur) {
cur->next = NULL;
cur->sublist = sublist;
} else {
head = sublist;
}
cur = sublist;
} else if (str[*index] == ',') { // 创建新节点
(*index)++;
Node *node = (Node *) malloc(sizeof(Node));
node->data = '\0';
node->next = NULL;
node->sublist = NULL;
if (cur) {
cur->next = node;
} else {
head = node;
}
cur = node;
} else { // 创建新节点
Node *node = (Node *) malloc(sizeof(Node));
node->data = str[*index];
node->next = NULL;
node->sublist = NULL;
if (cur) {
cur->next = node;
} else {
head = node;
}
cur = node;
(*index)++;
}
}
(*index)++;
return head;
}
// 创建广义表
Node *create_generalized_list(char *str) {
int index = 0;
if (str[index] == '(') {
index++;
return create_sublist(str, &index);
} else {
return NULL;
}
}
// 打印广义表
void print_generalized_list(Node *head) {
printf("(");
while (head) {
if (head->sublist) { // 打印子表
print_generalized_list(head->sublist);
head = head->next;
} else if (head->data == '\0') { // 打印分隔符
printf(",");
head = head->next;
} else { // 打印原子元素
printf("%c", head->data);
head = head->next;
}
}
printf(")");
}
int main() {
char *str = "(1,2,(3,4))";
Node *head = create_generalized_list(str);
print_generalized_list(head);
return 0;
}
```
在这个实现中,我们使用了一个结构体 `Node` 来表示广义表中的节点。其中,`data` 字段表示节点的数据,`next` 字段表示指向下一个节点的指针,`sublist` 字段表示指向子表的指针。在创建广义表时,我们首先判断当前字符是左括号、右括号还是逗号,然后根据不同的情况进行处理。如果当前字符是左括号,那么我们递归调用 `create_sublist` 函数来创建子表;如果当前字符是逗号,那么我们创建一个新节点作为分隔符;如果当前字符是原子元素,那么我们创建一个新节点来存储它。在创建完成节点后,我们将它添加到广义表中,并且更新指向最后一个节点的指针 `cur`。最后,我们返回广义表的头结点 `head`。
在程序的主函数中,我们先定义了一个字符串 `str` 来表示广义表的字符串表示。然后,我们调用 `create_generalized_list` 函数来创建广义表,并且调用 `print_generalized_list` 函数来打印广义表。在打印广义表时,我们首先打印左括号,然后遍历广义表中的所有节点。对于每个节点,如果它是一个子表,那么我们递归调用 `print_generalized_list` 函数来打印它;如果它是一个分隔符,那么我们打印逗号;如果它是一个原子元素,那么我们打印它。最后,我们打印右括号来结束广义表的打印。
注意:在实际编写代码时,我们应该为 `Node` 结构体添加一些边界检查代码,以避免访问非法内存。此外,我们也应该在程序运行结束后释放动态分配的内存。
阅读全文
相关推荐


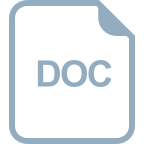
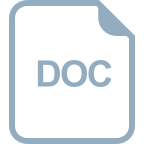
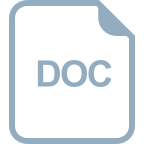
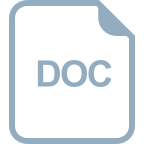
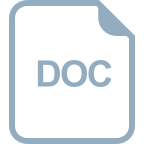
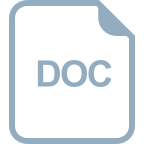
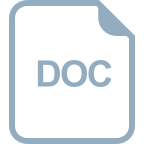



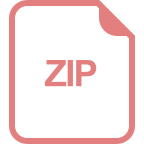
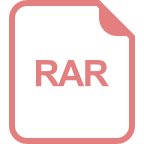
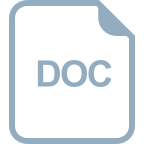