C语言实现二叉树广义表形二叉树式链表结构
时间: 2023-10-09 12:15:26 浏览: 120
二叉树广义表表示法是一种用字符串来表示二叉树的方法,在广义表中,每个节点都可以是一个数据元素,也可以是一个子树的广义表。广义表表示法可以用来表示二叉树,也可以用来表示一般的树。
二叉树广义表表示法是一种递归定义,它可以用以下的方式来表示一个二叉树:
1. 如果该二叉树为空,那么它的广义表表示为()。
2. 如果该二叉树不为空,那么它的广义表表示为“(数据元素,左子树广义表,右子树广义表)”。
例如,对于以下的二叉树:
```
A
/ \
B C
/ / \
D E F
```
它的广义表表示为:(A,B(D,(),()),C(E,(),F))
下面是C语言实现二叉树广义表形式的链表结构的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 二叉树结点
typedef struct node {
char data; // 数据域
struct node *left; // 左子树指针
struct node *right; // 右子树指针
} Node;
// 创建新结点
Node *new_node(char data) {
Node *node = (Node *)malloc(sizeof(Node));
node->data = data;
node->left = NULL;
node->right = NULL;
return node;
}
// 构建二叉树
Node *build_tree(char *str, int *index) {
Node *root = NULL;
if (str[*index] != '(') { // 如果当前字符不是'(',则返回空指针
return NULL;
}
*index += 1; // index指向下一个字符
if (str[*index] != ')') { // 如果当前字符不是')',则创建新结点
root = new_node(str[*index]);
*index += 1; // index指向下一个字符
root->left = build_tree(str, index); // 递归构建左子树
root->right = build_tree(str, index); // 递归构建右子树
}
*index += 1; // index指向下一个字符
return root;
}
// 中序遍历二叉树
void inorder(Node *root) {
if (root == NULL) {
return;
}
inorder(root->left);
printf("%c ", root->data);
inorder(root->right);
}
int main() {
char *str = "(A,B(D,(),()),C(E,(),F))";
int index = 0;
Node *root = build_tree(str, &index);
inorder(root);
return 0;
}
```
该程序将输出:D B A E C F
相关推荐
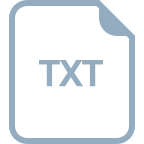
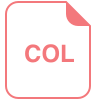
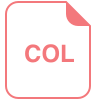
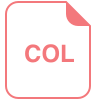
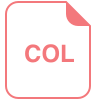
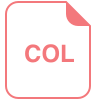









