signal.h使用例程
时间: 2023-08-06 20:01:56 浏览: 44
以下是一个使用 signal.h 的简单例程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
#include <unistd.h>
void signal_handler(int signal_num) {
printf("Received signal %d\n", signal_num);
}
int main() {
// 注册 SIGINT 信号的处理函数
signal(SIGINT, signal_handler);
printf("My PID is %d\n", getpid());
while(1) {
// 等待信号
sleep(1);
}
return 0;
}
```
这个例程中,我们定义了一个 signal_handler() 函数,当接收到 SIGINT 信号时,会输出 "Received signal n" 的信息。在 main() 函数中通过 `signal(SIGINT, signal_handler)` 注册了对 SIGINT 信号的处理函数,然后进入一个无限循环等待信号的到来。当我们在终端中按下 Ctrl+C 时,就会触发 SIGINT 信号,程序会调用 signal_handler() 函数并输出相应的信息。
相关问题
ubuntu系统下,使用qt creater,使图片输出成灰度图、降低分辨率的图片的main.cpp和mainwindow.cpp的例程,以及对应的.pro文件
以下是一个简单的例程,可以将图像转换为灰度图,并降低分辨率。需要在.pro文件中添加QT += widgets和QT += gui。
main.cpp
```c++
#include <QApplication>
#include "mainwindow.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
```
mainwindow.h
```c++
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QLabel>
#include <QImage>
#include <QSpinBox>
#include <QVBoxLayout>
#include <QHBoxLayout>
#include <QPushButton>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void openImage();
void convertToGray();
void reduceResolution();
private:
QLabel *imageLabel;
QImage image;
QSpinBox *spinBox;
};
#endif // MAINWINDOW_H
```
mainwindow.cpp
```c++
#include "mainwindow.h"
#include <QFileDialog>
#include <QMessageBox>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
imageLabel = new QLabel;
imageLabel->setBackgroundRole(QPalette::Base);
imageLabel->setSizePolicy(QSizePolicy::Ignored, QSizePolicy::Ignored);
imageLabel->setScaledContents(true);
QWidget *centralWidget = new QWidget(this);
setCentralWidget(centralWidget);
QVBoxLayout *mainLayout = new QVBoxLayout(centralWidget);
QHBoxLayout *buttonLayout = new QHBoxLayout;
QPushButton *openButton = new QPushButton(tr("Open"), this);
QPushButton *grayButton = new QPushButton(tr("Convert to Gray"), this);
QPushButton *reduceButton = new QPushButton(tr("Reduce Resolution"), this);
spinBox = new QSpinBox(this);
spinBox->setRange(1, 10);
spinBox->setValue(1);
buttonLayout->addWidget(openButton);
buttonLayout->addWidget(grayButton);
buttonLayout->addWidget(reduceButton);
buttonLayout->addWidget(spinBox);
mainLayout->addWidget(imageLabel);
mainLayout->addLayout(buttonLayout);
connect(openButton, SIGNAL(clicked()), this, SLOT(openImage()));
connect(grayButton, SIGNAL(clicked()), this, SLOT(convertToGray()));
connect(reduceButton, SIGNAL(clicked()), this, SLOT(reduceResolution()));
}
MainWindow::~MainWindow()
{
}
void MainWindow::openImage()
{
QString fileName = QFileDialog::getOpenFileName(this, tr("Open Image"), ".", tr("Image Files (*.png *.jpg *.bmp)"));
if (fileName.isEmpty())
return;
image.load(fileName);
if (image.isNull())
{
QMessageBox::information(this, tr("Error"), tr("Cannot load %1.").arg(fileName));
return;
}
imageLabel->setPixmap(QPixmap::fromImage(image));
}
void MainWindow::convertToGray()
{
if (image.isNull())
return;
image = image.convertToFormat(QImage::Format_Grayscale8);
imageLabel->setPixmap(QPixmap::fromImage(image));
}
void MainWindow::reduceResolution()
{
if (image.isNull())
return;
int factor = spinBox->value();
image = image.scaled(image.width() / factor, image.height() / factor);
imageLabel->setPixmap(QPixmap::fromImage(image));
}
```
.pro文件
```c++
QT += widgets gui
TARGET = imageConverter
TEMPLATE = app
SOURCES += main.cpp \
mainwindow.cpp
HEADERS += mainwindow.h
```
这个例程中,使用了Qt的几个基本控件,如QLabel、QSpinBox、QPushButton等,并且利用QImage类进行图像处理。openImage()函数用于打开图片,convertToGray()函数将图片转换为灰度图,reduceResolution()函数将图片降低分辨率。
linux下用户态定时器 例程
下面是一个Linux下用户态定时器的简单例程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
#include <time.h>
#include <unistd.h>
#define TIMER_SIG SIGRTMIN
void timer_handler(int sig, siginfo_t *si, void *uc)
{
printf("Timer expired.\n");
}
int main(int argc, char *argv[])
{
timer_t timerid;
struct sigevent sev;
struct itimerspec its;
struct sigaction sa;
// 设置定时器信号处理函数
sa.sa_flags = SA_SIGINFO;
sa.sa_sigaction = timer_handler;
sigemptyset(&sa.sa_mask);
if (sigaction(TIMER_SIG, &sa, NULL) == -1) {
perror("sigaction");
exit(EXIT_FAILURE);
}
// 创建定时器
sev.sigev_notify = SIGEV_SIGNAL;
sev.sigev_signo = TIMER_SIG;
sev.sigev_value.sival_ptr = &timerid;
if (timer_create(CLOCK_REALTIME, &sev, &timerid) == -1) {
perror("timer_create");
exit(EXIT_FAILURE);
}
// 设置定时器
its.it_value.tv_sec = 5; // 初始定时器时间为5秒
its.it_value.tv_nsec = 0;
its.it_interval.tv_sec = 1; // 定时器到期后每1秒重新启动一次
its.it_interval.tv_nsec = 0;
if (timer_settime(timerid, 0, &its, NULL) == -1) {
perror("timer_settime");
exit(EXIT_FAILURE);
}
// 循环等待定时器信号
while (1) {
sleep(1);
}
return 0;
}
```
该程序使用 `timer_create()` 创建一个定时器,并使用 `timer_settime()` 设置定时器的初始值和周期值。在定时器到期时,会触发一个 `SIGRTMIN` 信号,该信号将被注册的信号处理函数 `timer_handler()` 捕获并执行。在该例程中,我们简单地打印了一条消息来表示定时器已经到期。
要编译上述程序,请使用以下命令:
```
gcc -o timer timer.c
```
运行程序,它将等待5秒钟,然后输出一条消息,之后每隔1秒钟再输出一条消息。你可以使用 `Ctrl+C` 组合键来中断程序。
相关推荐
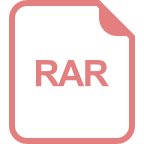
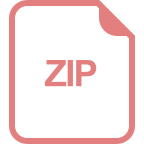
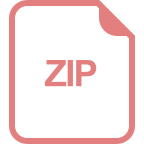










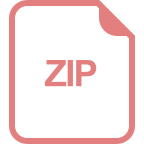
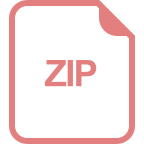
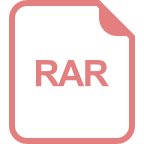