f=open("f.txt","w+") lst=["苹果","香蕉"] f.writelines(lst) for line in f: print(line) f.close() 输出结果是
时间: 2023-07-19 08:24:05 浏览: 69
由于文件指针已经指向文件末尾,因此在读取文件内容之前需要将文件指针置于文件开头。可以使用`f.seek(0)`函数将文件指针移动到文件开头。
另外,`f.writelines(lst)`函数会将列表`lst`中的元素按行写入文件,但是每行后面并没有加上换行符`\n`,因此在读取文件内容时需要手动加上换行符。
因此,正确的代码应该如下:
```python
f = open("f.txt", "w+")
lst = ["苹果", "香蕉"]
f.writelines(lst)
f.seek(0) # 将文件指针移动到文件开头
for line in f:
print(line.strip()) # 手动加上换行符
f.close()
```
输出结果为:
```
苹果
香蕉
```
相关问题
优化代码import os import re import pandas as pd from pandas import DataFrame lst1=[] lst2=[] path1 = r'D:\C-cn' path2 = r'D:\C-en' files1 = os.listdir(path1) files2 = os.listdir(path2) lst1=[] lst2=[] reg1=re.compile(r"[^。?!…]*[。?!……]") reg2=re.compile(r'.*\.[\n ]') df1 = [] df2 = [] for i in range(0,39): domain=os.path.abspath(r'D:\C-cn') file1=os.path.join(domain,files1[i]) fn = open(str(file1),encoding='gbk') f1 = fn.readlines() #print(f1) for line in f1: line=line.rstrip('\n') if "。" not in line: lst1.append(line) else: lis=reg1.findall(line) for j in lis: lst1.append(j) data1=DataFrame({"Chinese":lst1}) df1.append(data1) df1 = pd.concat(df1) df1.to_excel("Chinese.xlsx",index="false") for i in range(0,39): domains=os.path.abspath(r'D:\C-en') file2=os.path.join(domains,files2[i]) ft = open(str(file2),encoding='gbk') f2 = ft.readlines() print(f2) for line in f2: if "." not in line: line=line.rstrip("\n") lst2.append(line) else: liss=line.split(". ") for j in liss: j=j+"." lst2.append(j) data2=DataFrame({"English":lst2}) df2.append(data2) df2 = pd.concat(df2)# 合并所有数据 df2.to_excel("English.xlsx",index="false")
这段代码可以进行一些优化,包括:
1. 将重复的代码封装成函数,避免重复代码。
2. 不需要在每次迭代时都重新初始化 `lst1` 和 `lst2` 列表,可以将其初始化放在循环外面。
3. 在使用 `pandas` 库时,最好使用 `read_csv()` 函数和 `to_csv()` 函数,而不是 `DataFrame()` 和 `to_excel()` 函数。
下面是优化后的代码:
```python
import os
import re
import pandas as pd
def get_sentences(file_path, regex):
with open(file_path, encoding='gbk') as f:
lines = f.readlines()
sentences = []
for line in lines:
line = line.rstrip('\n')
if "。" not in line:
sentences.append(line)
else:
lis = regex.findall(line)
for j in lis:
sentences.append(j)
return sentences
def get_english_sentences(file_path):
with open(file_path, encoding='gbk') as f:
lines = f.readlines()
sentences = []
for line in lines:
if "." not in line:
line = line.rstrip("\n")
sentences.append(line)
else:
liss = line.split(". ")
for j in liss:
j = j + "."
sentences.append(j)
return sentences
path1 = r'D:\C-cn'
path2 = r'D:\C-en'
files1 = os.listdir(path1)
files2 = os.listdir(path2)
reg1 = re.compile(r"[^。?!…]*[。?!……]")
reg2 = re.compile(r'.*\.[\n ]')
lst1 = []
for i in range(0, 39):
file1 = os.path.join(path1, files1[i])
lst1 += get_sentences(file1, reg1)
lst2 = []
for i in range(0, 39):
file2 = os.path.join(path2, files2[i])
lst2 += get_english_sentences(file2)
df1 = pd.DataFrame({"Chinese": lst1})
df1.to_csv("Chinese.csv", index=False)
df2 = pd.DataFrame({"English": lst2})
df2.to_csv("English.csv", index=False)
```
这里将中文和英文的句子分别存储到了 `Chinese.csv` 和 `English.csv` 文件中,以便后续的处理和分析。
def Freq(line): lst=[] count1=0 for i in line: if i not in lst: lst.append(i) count1+=1 print(count1) lst.sort() for j in lst: count2=0 for i in line: if j==i: count2+=1 print('%s = %d'%(j,count2))正确编写格式是什么
正确的格式应该是:
```python
def Freq(line):
lst=[]
count1=0
for i in line:
if i not in lst:
lst.append(i)
count1+=1
print(count1)
lst.sort()
for j in lst:
count2=0
for i in line:
if j==i:
count2+=1
print('%s = %d'%(j,count2))
```
注意代码缩进和函数定义后面的冒号。
阅读全文
相关推荐
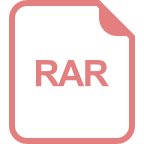
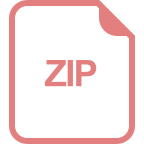
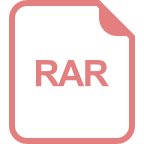


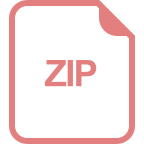
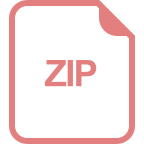
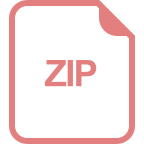
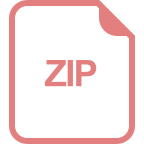
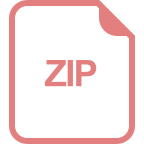
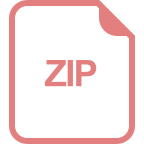
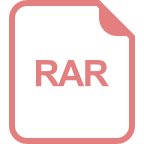