arduino数据传输到mysql
时间: 2023-10-17 11:12:21 浏览: 76
要将Arduino数据传输到MySQL,可以使用以下步骤:
1. 首先,需要编写一个Arduino程序来收集传感器数据并将其发送到计算机上运行的PHP脚本。可以使用Arduino的串行通信库来与计算机进行通信。可以使用以下代码示例从Arduino发送数据:
```
// Setup the serial port at 9600 baud
void setup() {
Serial.begin(9600);
}
// Read the sensor data and send it over serial
void loop() {
int sensorValue = analogRead(A0);
Serial.println(sensorValue);
delay(1000);
}
```
2. 在计算机上,需要编写一个PHP脚本来接收从Arduino发送的数据并将其插入到MySQL数据库中。可以使用以下代码示例来获取从Arduino发送的数据并将其插入到MySQL数据库中:
```
<?php
// Connect to the MySQL database
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "database";
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Get the data sent from the Arduino
$data = $_GET['data'];
// Insert the data into the MySQL database
$sql = "INSERT INTO sensor_data (value) VALUES ('$data')";
if ($conn->query($sql) === TRUE) {
echo "New record created successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
// Close the MySQL connection
$conn->close();
?>
```
3. 最后,需要在Arduino程序中使用HTTP GET请求将传感器数据发送到PHP脚本。可以使用Arduino的Ethernet或WiFi库来进行HTTP请求。可以使用以下代码示例从Arduino发送HTTP GET请求:
```
#include <Ethernet.h>
// Set the MAC address and IP address of the Arduino
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
IPAddress ip(192, 168, 1, 177);
// Set the IP address of the server running the PHP script
IPAddress server(192, 168, 1, 100);
// Set the path to the PHP script
char path[] = "/insert_data.php";
// Setup the Ethernet client
EthernetClient client;
void setup() {
// Start the Ethernet connection
Ethernet.begin(mac, ip);
// Wait for the Ethernet connection to be established
while (!Ethernet.ready()) {
delay(1000);
}
}
void loop() {
// Read the sensor data
int sensorValue = analogRead(A0);
// Send an HTTP GET request to the PHP script with the sensor data
if (client.connect(server, 80)) {
client.print("GET ");
client.print(path);
client.print("?data=");
client.print(sensorValue);
client.println(" HTTP/1.1");
client.println("Host: 192.168.1.100");
client.println("Connection: close");
client.println();
}
// Wait for a response from the server
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.print(c);
}
}
// Wait for 1 second before sending the next request
delay(1000);
}
```
使用以上步骤,就可以将Arduino数据传输到MySQL了。
相关推荐
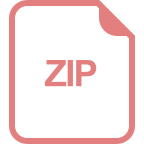
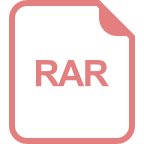







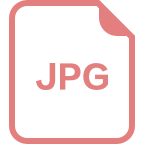
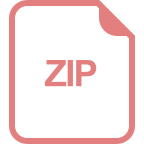