写一个基于Tensorflow的fashion mnist数据集识别算法(不用卷积神经网络)
时间: 2023-05-31 09:03:29 浏览: 70
以下是一个基于Tensorflow的fashion mnist数据集识别算法的示例,使用的是全连接神经网络:
```python
import tensorflow as tf
from tensorflow import keras
import numpy as np
# 加载数据集
fashion_mnist = keras.datasets.fashion_mnist
(train_images, train_labels), (test_images, test_labels) = fashion_mnist.load_data()
# 数据预处理
train_images = train_images / 255.0
test_images = test_images / 255.0
# 定义模型
model = keras.Sequential([
keras.layers.Flatten(input_shape=(28, 28)),
keras.layers.Dense(128, activation='relu'),
keras.layers.Dense(10)
])
# 编译模型
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# 训练模型
model.fit(train_images, train_labels, epochs=10)
# 评估模型
test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=2)
print('\nTest accuracy:', test_acc)
# 预测
predictions = model.predict(test_images)
predicted_labels = np.argmax(predictions, axis=1)
```
该模型使用一个Flatten层来将二维的图像数据展平成一维的向量,然后通过一个128个神经元的全连接层和一个输出10个类别的全连接层进行预测。在训练过程中,使用Adam优化器和交叉熵损失函数进行模型训练。最终通过在测试集上的表现来评估模型的准确性,并使用预测结果来预测测试集中的标签。
相关推荐
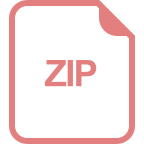
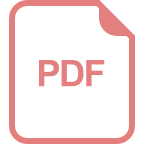
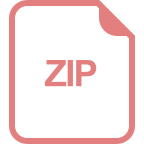














