用python写一个考勤管理系统,并且连接到mysql
时间: 2023-11-28 19:48:05 浏览: 47
好的,首先需要安装好MySQL数据库和Python的mysql-connector包。
接下来,我们可以按照以下步骤来实现一个简单的考勤管理系统:
1. 创建一个名为attendance的数据库,并在其中创建一个名为students的表,用于存储学生信息和考勤记录。
```python
import mysql.connector
# 连接MySQL数据库
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="attendance"
)
# 创建一个students表
mycursor = mydb.cursor()
mycursor.execute("CREATE TABLE students (id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), attendance INT)")
```
2. 编写一个函数,用于添加新的学生记录。
```python
def add_student(name):
# 向students表中插入一条新记录
sql = "INSERT INTO students (name, attendance) VALUES (%s, 0)"
val = (name,)
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record inserted.")
```
3. 编写一个函数,用于进行考勤操作。
```python
def check_attendance(name):
# 更新学生的attendance字段
sql = "UPDATE students SET attendance = 1 WHERE name = %s"
val = (name,)
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "record(s) affected.")
```
4. 编写一个函数,用于显示当前所有学生的考勤记录。
```python
def show_attendance():
# 查询students表中所有记录
mycursor.execute("SELECT * FROM students")
myresult = mycursor.fetchall()
# 打印每个学生的考勤记录
for x in myresult:
print(x[1], "has", ("attended." if x[2] == 1 else "not attended."))
```
5. 最后,我们可以编写一个简单的命令行界面,使用户可以通过输入命令来进行操作。
```python
while True:
# 显示命令提示
print("\nAvailable commands:")
print("add [name] - add a new student")
print("check [name] - check attendance for a student")
print("show - show attendance for all students")
print("exit - exit the program")
# 获取用户输入的命令
cmd = input("> ")
# 处理用户输入的命令
if cmd.startswith("add "):
name = cmd.split(" ")[1]
add_student(name)
elif cmd.startswith("check "):
name = cmd.split(" ")[1]
check_attendance(name)
elif cmd == "show":
show_attendance()
elif cmd == "exit":
break
else:
print("Invalid command.")
```
这样,一个简单的考勤管理系统就完成了。用户可以通过add命令添加新学生,通过check命令对学生进行考勤操作,通过show命令查看所有学生的考勤记录。所有数据都存储在MySQL数据库中。
相关推荐
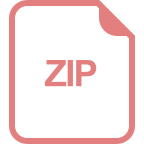
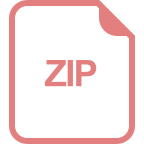














