c++用ffmpeg创建rtsp服务器
时间: 2023-10-05 16:11:48 浏览: 56
要使用 C++ 和 FFmpeg 创建 RTSP 服务器,你需要了解以下几个步骤:
1. 安装 FFmpeg 库:你需要从 FFmpeg 官网下载源代码,并按照说明进行编译和安装。
2. 创建 RTSP 服务器:你需要编写一个 C++ 程序来创建 RTSP 服务器。可以使用 Live555 或者 FFmpeg 提供的库来完成这个任务。如果你选择使用 FFmpeg,你需要使用下面的代码来创建 RTSP 服务器:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <errno.h>
#include <signal.h>
#include <pthread.h>
#include <arpa/inet.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <netinet/tcp.h>
#include <sys/ioctl.h>
#include <net/if.h>
#include <fcntl.h>
#include <stdbool.h>
#include <libavutil/avutil.h>
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
#include <libavutil/opt.h>
#include <libavutil/error.h>
#include <libavutil/time.h>
#include <libavutil/mathematics.h>
#include <libswscale/swscale.h>
#include <libswresample/swresample.h>
#include <libavutil/imgutils.h>
#define RTSP_PORT 554
#define MAX_CLIENTS 10
typedef struct RTSPClient {
int state;
int socket;
struct sockaddr_in address;
AVFormatContext *pFormatCtx;
AVCodecContext *pCodecCtx;
AVCodec *pCodec;
AVFrame *pFrame;
AVPacket packet;
struct SwsContext *img_convert_ctx;
int video_stream_index;
pthread_t thread_id;
bool is_running;
} RTSPClient;
RTSPClient clients[MAX_CLIENTS];
void* client_thread(void *arg) {
RTSPClient *client = (RTSPClient*)arg;
int ret;
AVPacket packet;
AVFrame *frame;
uint8_t *buffer;
int buffer_size;
struct timeval tv;
int64_t start_time, end_time, pts;
int64_t duration, sleep_time;
int64_t last_frame_pts = -1;
client->is_running = true;
while (client->is_running) {
ret = av_read_frame(client->pFormatCtx, &packet);
if (ret < 0) {
if (ret == AVERROR_EOF) {
printf("End of stream\n");
break;
} else if (ret == AVERROR(EAGAIN)) {
usleep(1000);
continue;
} else {
char errbuf[AV_ERROR_MAX_STRING_SIZE];
av_make_error_string(errbuf, AV_ERROR_MAX_STRING_SIZE, ret);
printf("Error while reading frame: %s\n", errbuf);
break;
}
}
if (packet.stream_index == client->video_stream_index) {
frame = av_frame_alloc();
if (!frame) {
printf("Error allocating frame\n");
av_packet_unref(&packet);
break;
}
ret = avcodec_send_packet(client->pCodecCtx, &packet);
if (ret < 0) {
av_frame_free(&frame);
av_packet_unref(&packet);
char errbuf[AV_ERROR_MAX_STRING_SIZE];
av_make_error_string(errbuf, AV_ERROR_MAX_STRING_SIZE, ret);
printf("Error sending packet to decoder: %s\n", errbuf);
break;
}
while (ret >= 0) {
ret = avcodec_receive_frame(client->pCodecCtx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
av_frame_free(&frame);
break;
} else if (ret < 0) {
av_frame_free(&frame);
char errbuf[AV_ERROR_MAX_STRING_SIZE];
av_make_error_string(errbuf, AV_ERROR_MAX_STRING_SIZE, ret);
printf("Error receiving frame from decoder: %s\n", errbuf);
break;
}
if (frame->pts != AV_NOPTS_VALUE) {
pts = av_rescale_q(frame->pts, client->pFormatCtx->streams[client->video_stream_index]->time_base, AV_TIME_BASE_Q);
} else {
duration = av_frame_get_pkt_duration(frame);
pts = last_frame_pts + duration;
}
last_frame_pts = pts;
buffer_size = av_image_get_buffer_size(client->pCodecCtx->pix_fmt, client->pCodecCtx->width, client->pCodecCtx->height, 1);
buffer = (uint8_t*)av_malloc(buffer_size);
av_image_fill_arrays(frame->data, frame->linesize, buffer, client->pCodecCtx->pix_fmt, client->pCodecCtx->width, client->pCodecCtx->height, 1);
av_init_packet(&packet);
packet.data = buffer;
packet.size = buffer_size;
packet.pts = pts;
packet.dts = pts;
packet.duration = duration;
packet.stream_index = 0;
gettimeofday(&tv, NULL);
start_time = tv.tv_sec * 1000000 + tv.tv_usec;
av_packet_rescale_ts(&packet, client->pFormatCtx->streams[client->video_stream_index]->time_base, client->pFormatCtx->streams[0]->time_base);
ret = av_interleaved_write_frame(client->pFormatCtx, &packet);
if (ret < 0) {
char errbuf[AV_ERROR_MAX_STRING_SIZE];
av_make_error_string(errbuf, AV_ERROR_MAX_STRING_SIZE, ret);
printf("Error writing frame: %s\n", errbuf);
}
gettimeofday(&tv, NULL);
end_time = tv.tv_sec * 1000000 + tv.tv_usec;
sleep_time = (duration * 1000000 - (end_time - start_time)) / 1000;
if (sleep_time > 0) {
usleep(sleep_time);
}
av_packet_unref(&packet);
av_free(buffer);
av_frame_free(&frame);
}
} else {
av_packet_unref(&packet);
}
}
avformat_close_input(&client->pFormatCtx);
avcodec_free_context(&client->pCodecCtx);
av_frame_free(&client->pFrame);
sws_freeContext(client->img_convert_ctx);
close(client->socket);
client->state = 0;
return NULL;
}
int main(int argc, char *argv[]) {
int ret;
int server_socket;
int client_socket;
struct sockaddr_in server_address;
struct sockaddr_in client_address;
socklen_t client_address_len;
char buf[1024];
int n;
int i;
int optval;
RTSPClient *client;
av_register_all();
avformat_network_init();
server_socket = socket(AF_INET, SOCK_STREAM, 0);
if (server_socket < 0) {
printf("Error creating socket: %s\n", strerror(errno));
return 1;
}
optval = 1;
setsockopt(server_socket, SOL_SOCKET, SO_REUSEADDR, &optval, sizeof(optval));
memset(&server_address, 0, sizeof(server_address));
server_address.sin_family = AF_INET;
server_address.sin_addr.s_addr = htonl(INADDR_ANY);
server_address.sin_port = htons(RTSP_PORT);
ret = bind(server_socket, (struct sockaddr*)&server_address, sizeof(server_address));
if (ret < 0) {
printf("Error binding socket: %s\n", strerror(errno));
close(server_socket);
return 1;
}
ret = listen(server_socket, 5);
if (ret < 0) {
printf("Error listening on socket: %s\n", strerror(errno));
close(server_socket);
return 1;
}
for (i = 0; i < MAX_CLIENTS; i++) {
clients[i].state = 0;
}
while (1) {
client_address_len = sizeof(client_address);
client_socket = accept(server_socket, (struct sockaddr*)&client_address, &client_address_len);
if (client_socket < 0) {
printf("Error accepting connection: %s\n", strerror(errno));
continue;
}
for (i = 0; i < MAX_CLIENTS; i++) {
if (clients[i].state == 0) {
client = &clients[i];
break;
}
}
if (i == MAX_CLIENTS) {
printf("Too many clients\n");
close(client_socket);
continue;
}
client->state = 1;
client->socket = client_socket;
client->address = client_address;
client->pFormatCtx = avformat_alloc_context();
client->pCodecCtx = NULL;
client->pCodec = NULL;
client->pFrame = NULL;
client->img_convert_ctx = NULL;
client->video_stream_index = -1;
ret = avformat_open_input(&client->pFormatCtx, "video.mp4", NULL, NULL);
if (ret < 0) {
char errbuf[AV_ERROR_MAX_STRING_SIZE];
av_make_error_string(errbuf, AV_ERROR_MAX_STRING_SIZE, ret);
printf("Error opening input file: %s\n", errbuf);
close(client_socket);
client->state = 0;
continue;
}
ret = avformat_find_stream_info(client->pFormatCtx, NULL);
if (ret < 0) {
char errbuf[AV_ERROR_MAX_STRING_SIZE];
av_make_error_string(errbuf, AV_ERROR_MAX_STRING_SIZE, ret);
printf("Error finding stream info: %s\n", errbuf);
avformat_close_input(&client->pFormatCtx);
close(client_socket);
client->state = 0;
continue;
}
for (i = 0; i < client->pFormatCtx->nb_streams; i++) {
if (client->pFormatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
client->video_stream_index = i;
break;
}
}
if (client->video_stream_index == -1) {
printf("No video stream found\n");
avformat_close_input(&client->pFormatCtx);
close(client_socket);
client->state = 0;
continue;
}
client->pCodec = avcodec_find_decoder(client->pFormatCtx->streams[client->video_stream_index]->codecpar->codec_id);
if (!client->pCodec) {
printf("Codec not found\n");
avformat_close_input(&client->pFormatCtx);
close(client_socket);
client->state = 0;
continue;
}
client->pCodecCtx = avcodec_alloc_context3(client->pCodec);
if (!client->pCodecCtx) {
printf("Error allocating codec context\n");
avformat_close_input(&client->pFormatCtx);
close(client_socket);
client->state = 0;
continue;
}
ret = avcodec_parameters_to_context(client->pCodecCtx, client->pFormatCtx->streams[client->video_stream_index]->codecpar);
if (ret < 0) {
char errbuf[AV_ERROR_MAX_STRING_SIZE];
av_make_error_string(errbuf, AV_ERROR_MAX_STRING_SIZE, ret);
printf("Error setting codec parameters: %s\n", errbuf);
avcodec_free_context(&client->pCodecCtx);
avformat_close_input(&client->pFormatCtx);
close(client_socket);
client->state = 0;
continue;
}
ret = avcodec_open2(client->pCodecCtx, client->pCodec, NULL);
if (ret < 0) {
char errbuf[AV_ERROR_MAX_STRING_SIZE];
av_make_error_string(errbuf, AV_ERROR_MAX_STRING_SIZE, ret);
printf("Error opening codec: %s\n", errbuf);
avcodec_free_context(&client->pCodecCtx);
avformat_close_input(&client->pFormatCtx);
close(client_socket);
client->state = 0;
continue;
}
client->pFrame = av_frame_alloc();
if (!client->pFrame) {
printf("Error allocating frame\n");
avcodec_free_context(&client->pCodecCtx);
avformat_close_input(&client->pFormatCtx);
close(client_socket);
client->state = 0;
continue;
}
client->img_convert_ctx = sws_getContext(client->pCodecCtx->width, client->pCodecCtx->height, client->pCodecCtx->pix_fmt, client->pCodecCtx->width, client->pCodecCtx->height, AV_PIX_FMT_YUV420P, SWS_BICUBIC, NULL, NULL, NULL);
if (!client->img_convert_ctx) {
printf("Error creating img_convert_ctx\n");
av_frame_free(&client->pFrame);
avcodec_free_context(&client->pCodecCtx);
avformat_close_input(&client->pFormatCtx);
close(client_socket);
client->state = 0;
continue;
}
ret = pthread_create(&client->thread_id, NULL, client_thread, client);
if (ret != 0) {
printf("Error creating thread\n");
av_frame_free(&client->pFrame);
sws_freeContext(client->img_convert_ctx);
avcodec_free_context(&client->pCodecCtx);
avformat_close_input(&client->pFormatCtx);
close(client_socket);
client->state = 0;
continue;
}
}
for (i = 0; i < MAX_CLIENTS; i++) {
if (clients[i].state == 1) {
clients[i].is_running = false;
pthread_join(clients[i].thread_id, NULL);
av_frame_free(&clients[i].pFrame);
sws_freeContext(clients[i].img_convert_ctx);
avcodec_free_context(&clients[i].pCodecCtx);
avformat_close_input(&clients[i].pFormatCtx);
close(clients[i].socket);
}
}
avformat_network_deinit();
av_free_all();
return 0;
}
```
3. 启动 RTSP 服务器:你需要在终端上运行上面编写好的 C++ 程序,然后根据需要设置你的客户端连接地址和端口号,并开始发送数据。
以上就是使用 C++ 和 FFmpeg 创建 RTSP 服务器的基本步骤。如果你不想从头开始编写代码,也可以使用现成的 RTSP 服务器实现,如 Live555 或者 FFmpeg 提供的 RTSP 服务器。
相关推荐
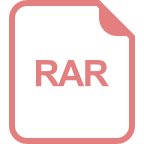
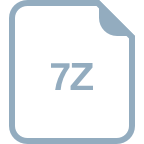













