图像分割指标计算recall代码
时间: 2023-12-09 18:05:38 浏览: 57
以下是Python实现图像分割中Recall指标的示例代码:
```python
def recall(true_mask, pred_mask):
true_positive = np.logical_and(true_mask, pred_mask)
false_negative = np.logical_and(true_mask, np.logical_not(pred_mask))
return true_positive.sum() / (true_positive.sum() + false_negative.sum())
```
该函数接受两个二值化的mask作为输入,并返回Recall指标值。其中,true_positive是真正例的掩膜,即预测为正例且真实为正例的像素掩膜;false_negative是假负例的掩膜,即预测为负例但真实为正例的像素掩膜。Recall指标定义为真正例的数量除以真正例和假负例的数量之和,计算方法与准确率略有不同。
相关问题
图像分割指标计算python
图像分割的指标有很多种,其中常用的有Jaccard Index、Dice Coefficient、Precision、Recall、F1 Score等。以下是Python实现这些指标的示例代码:
Jaccard Index:
```python
def jaccard_index(true_mask, pred_mask):
intersection = np.logical_and(true_mask, pred_mask)
union = np.logical_or(true_mask, pred_mask)
return intersection.sum() / union.sum()
```
Dice Coefficient:
```python
def dice_coefficient(true_mask, pred_mask):
intersection = np.logical_and(true_mask, pred_mask)
return 2. * intersection.sum() / (true_mask.sum() + pred_mask.sum())
```
Precision:
```python
def precision(true_mask, pred_mask):
true_positive = np.logical_and(true_mask, pred_mask)
false_positive = np.logical_and(np.logical_not(true_mask), pred_mask)
return true_positive.sum() / (true_positive.sum() + false_positive.sum())
```
Recall:
```python
def recall(true_mask, pred_mask):
true_positive = np.logical_and(true_mask, pred_mask)
false_negative = np.logical_and(true_mask, np.logical_not(pred_mask))
return true_positive.sum() / (true_positive.sum() + false_negative.sum())
```
F1 Score:
```python
def f1_score(true_mask, pred_mask):
p = precision(true_mask, pred_mask)
r = recall(true_mask, pred_mask)
return 2. * p * r / (p + r)
```
这些函数都接受两个二值化的mask作为输入,并返回相应的指标值。可以根据自己的需要选择合适的指标函数。
图像分割评估指标代码
图像分割评估指标是用来衡量图像分割算法性能的重要指标。以下是一个示例代码,用于计算图像分割的常见评估指标,包括精确度(Accuracy)、召回率(Recall)、特异度(Specificity)、F1分数(F1 Score)和IoU(Intersection over Union)。
```python
import numpy as np
def evaluate_segmentation(true_mask, pred_mask):
true_mask = true_mask.astype(bool)
pred_mask = pred_mask.astype(bool)
# 计算真阳性、假阳性和假阴性
true_positive = np.logical_and(true_mask, pred_mask).sum()
false_positive = np.logical_and(np.logical_not(true_mask), pred_mask).sum()
false_negative = np.logical_and(true_mask, np.logical_not(pred_mask)).sum()
# 计算精确度、召回率和特异度
accuracy = (true_positive + true_negative) / (true_positive + true_negative + false_positive + false_negative)
recall = true_positive / (true_positive + false_negative)
specificity = true_negative / (true_negative + false_positive)
# 计算F1分数
precision = true_positive / (true_positive + false_positive)
f1_score = 2 * (precision * recall) / (precision + recall)
# 计算IoU
intersection = np.logical_and(true_mask, pred_mask).sum()
union = np.logical_or(true_mask, pred_mask).sum()
iou = intersection / union
return accuracy, recall, specificity, f1_score, iou
# 示例用法
true_mask = np.array([[0, 1, 0],
[1, 1, 1],
[0, 1, 0]])
pred_mask = np.array([[0, 1, 0],
[0, 1, 0],
[0, 1, 0]])
accuracy, recall, specificity, f1_score, iou = evaluate_segmentation(true_mask, pred_mask)
print("Accuracy:", accuracy)
print("Recall:", recall)
print("Specificity:", specificity)
print("F1 Score:", f1_score)
print("IoU:", iou)
```
这段代码定义了一个`evaluate_segmentation`函数,该函数接受两个二值化的掩膜(true_mask和pred_mask),并计算出精确度、召回率、特异度、F1分数和IoU。在示例中,我们使用了一个3x3的真实掩膜和预测掩膜进行了计算,并打印了结果。
相关推荐
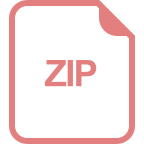
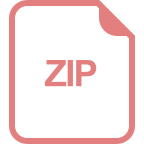












