机器学习绘制误差随迭代次数的变化曲线的代码
时间: 2024-05-14 21:12:44 浏览: 141
以下是使用Python和matplotlib库绘制误差随迭代次数的变化曲线的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成随机数据
x = np.arange(1, 101)
y = np.random.normal(0, 1, size=(100,))
# 初始化权重和偏置
w = 0
b = 0
lr = 0.01 # 学习率
epochs = 100 # 迭代次数
errors = [] # 记录每次迭代的误差
# 线性回归模型
def linear_regression(x, w, b):
return w * x + b
# 均方误差损失函数
def mean_squared_error(y_true, y_pred):
return np.mean(np.square(y_true - y_pred))
# 梯度下降算法
def gradient_descent(x, y, w, b, lr, epochs):
n = len(x)
for i in range(epochs):
y_pred = linear_regression(x, w, b)
error = mean_squared_error(y, y_pred)
errors.append(error)
dw = (-2/n) * np.sum(x * (y - y_pred))
db = (-2/n) * np.sum(y - y_pred)
w = w - lr * dw
b = b - lr * db
return w, b
# 运行梯度下降算法并绘制误差随迭代次数的变化曲线
w, b = gradient_descent(x, y, w, b, lr, epochs)
plt.plot(np.arange(epochs), errors)
plt.title("Error vs. Iterations")
plt.xlabel("Iterations")
plt.ylabel("Error")
plt.show()
```
这段代码生成了一个随机数据集,然后使用梯度下降算法对线性回归模型进行训练,并记录每次迭代的误差。最后,使用matplotlib库绘制误差随迭代次数的变化曲线。请注意,这只是一个示例代码,实际应用中的模型和数据可能会有所不同。
阅读全文
相关推荐
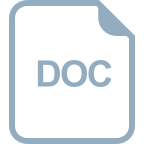
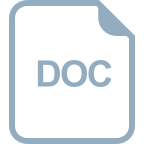
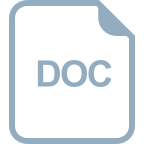
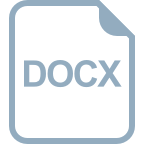
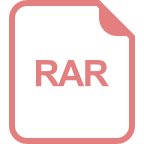
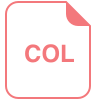
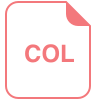
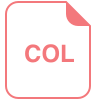
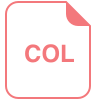
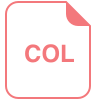
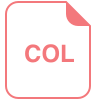
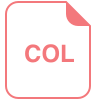
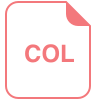
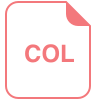
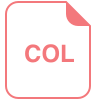
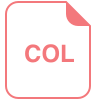


