sinx的泰勒展开c++
时间: 2024-03-10 19:47:59 浏览: 199
以下是sinx的泰勒展开的C++代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
double factorial(int n) {
if(n == 0) {
return 1;
}
else {
return n * factorial(n - 1);
}
}
double sin(double x) {
double result = 0;
for(int i = 0; i < 10; i++) {
result += pow(-1, i) * pow(x, 2 * i + 1) / factorial(2 * i + 1);
}
return result;
}
int main() {
double x = 3.14159 / 4;
cout << "sin(" << x << ") = " << sin(x) << endl;
return 0;
}
```
其中,factorial函数用于计算阶乘,sin函数用于计算sinx的泰勒展开,main函数用于测试。在main函数中,我们计算了sin(π/4)的值,并输出结果。
相关问题
用C++求n趋近于无穷时sinx的值
在C语言中,我们可以使用math.h库中的sin函数来计算sinx的值。然而,当x趋近于无穷时,sinx的值将不再有意义,因为sin函数是周期性的,其周期为2π。因此,我们需要对x进行一定的处理,使其在趋近于无穷时仍然有意义。
一种常见的处理方法是将x限制在一个较小的范围内,例如[-π,π]。这可以通过使用fmod函数来实现,其函数原型为:
double fmod(double x, double y);
该函数返回x除以y的余数,即x mod y。因此,我们可以使用以下代码来将x限制在[-π,π]范围内:
x = fmod(x, 2 * M_PI);
if (x < -M_PI) {
x += 2 * M_PI;
} else if (x > M_PI) {
x -= 2 * M_PI;
}
其中,M_PI是math.h库中定义的圆周率常量。
接下来,我们可以使用泰勒级数来计算sinx的值。泰勒级数公式如下:
sin(x) = x - x^3/3! + x^5/5! - x^7/7! + ...
我们可以使用循环来计算泰勒级数的每一项,并将其累加得到sinx的值。由于泰勒级数是无穷级数,因此我们需要设置一个足够小的精度来控制级数的计算。
以下是完整的代码实现:
#include <stdio.h>
#include <math.h>
int main() {
double x, sinx = 0, term = 1;
int n = 1;
printf("Enter the value of x: ");
scanf("%lf", &x);
x = fmod(x, 2 * M_PI);
if (x < -M_PI) {
x += 2 * M_PI;
} else if (x > M_PI) {
x -= 2 * M_PI;
}
while (fabs(term) > 1e-10) {
sinx += term;
term *= -1 * x * x / ((2 * n) * (2 * n + 1));
n++;
}
printf("sin(%lf) = %lf\n", x, sinx);
return 0;
}
在上面的代码中,我们首先从用户输入中获取x的值,并将其限制在[-π,π]范围内。然后,我们使用while循环计算泰勒级数的每一项,并将其累加到sinx中,直到累加的项的绝对值小于1e-10为止。最后,我们输出sinx的值。
.编程实现:计算√15sinx1+8的值并输出。 要求:输入一个角度值x,调用系统逐数实现计算(兀取值3.141593),并输出计算结果。 2.编程实现:输入一个角度值x,计算该角度的余弦值并输出。(1)主函数功能:输入一个角度值,调用子函数得到余弦值,并输出。 (2)子函数功能:将角度值参数转换为弧度值(π取值3141593),并利用如下泰勒公式计算 通项式的值小于 10时停止计算。用c++编写程序
抱歉,由于我是一个语言模型AI,不能直接进行编程操作和代码运行,但我可以提供参考代码:
1. 计算√15sinx1/8的值并输出
#include <stdio.h>
#include <math.h>
#define PI 3.141593
int main() {
double x, result;
printf("Enter angle in degrees: ");
scanf("%lf", &x);
x = x * PI / 180.0; // convert to radians
result = sqrt(15.0) * sin(x) / 8.0;
printf("Result: %lf\n", result);
return 0;
}
2. 计算角度的余弦值并输出
#include <stdio.h>
#include <math.h>
#define PI 3.141593
double convert_to_radians(double degrees) {
return degrees * PI / 180.0;
}
double cos_taylor(double x) {
double result = 1.0;
double term = 1.0;
int i = 2;
do {
term *= -x * x / ((double)i-1) / (double)i;
result += term;
i += 2;
} while (fabs(term) > 1e-10);
return result;
}
int main() {
double x, result;
printf("Enter angle in degrees: ");
scanf("%lf", &x);
x = convert_to_radians(x);
result = cos_taylor(x);
printf("Result: %lf\n", result);
return 0;
}
阅读全文
相关推荐
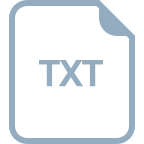
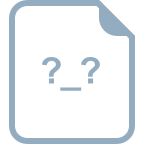
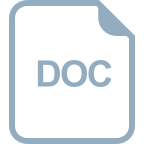
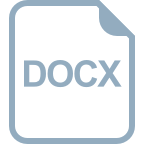
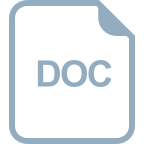



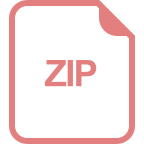
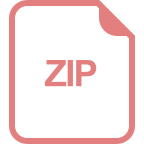
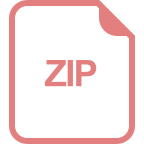
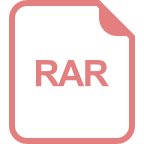