linux C多线程实现回调函数访问共享资源和数据同步的程序
时间: 2024-02-27 22:58:41 浏览: 96
以下是一个简单的示例程序,展示了如何在多线程中实现回调函数访问共享资源和数据同步:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
typedef struct {
int value;
pthread_mutex_t mutex;
pthread_cond_t cond;
} shared_data_t;
void* thread_func(void* arg);
void callback_func(void* arg) {
shared_data_t* shared_data = (shared_data_t*) arg;
pthread_mutex_lock(&shared_data->mutex);
shared_data->value++;
pthread_cond_signal(&shared_data->cond);
pthread_mutex_unlock(&shared_data->mutex);
}
int main() {
shared_data_t shared_data = {0, PTHREAD_MUTEX_INITIALIZER, PTHREAD_COND_INITIALIZER};
pthread_t thread;
if (pthread_create(&thread, NULL, thread_func, (void*) &shared_data) != 0) {
fprintf(stderr, "Error creating thread.\n");
exit(1);
}
pthread_mutex_lock(&shared_data.mutex);
while (shared_data.value == 0) {
pthread_cond_wait(&shared_data.cond, &shared_data.mutex);
}
printf("Value updated by callback: %d\n", shared_data.value);
pthread_mutex_unlock(&shared_data.mutex);
pthread_join(thread, NULL);
return 0;
}
void* thread_func(void* arg) {
shared_data_t* shared_data = (shared_data_t*) arg;
// Do some work...
callback_func(shared_data);
return NULL;
}
```
在这个程序中,我们定义了一个 `shared_data_t` 结构体,其中包含一个整数值 `value`,一个互斥锁 `mutex` 和一个条件变量 `cond`。我们使用互斥锁来保护共享资源 `value` 的访问,使用条件变量来实现线程之间的同步。
在 `thread_func` 函数中,我们调用了回调函数 `callback_func`,并将共享数据结构体的指针作为参数传递给它。在 `callback_func` 中,我们先使用互斥锁锁定共享数据结构体,然后对 `value` 进行更新,并使用条件变量通知其他等待线程。最后,我们释放互斥锁。
在 `main` 函数中,我们启动一个新的线程,并等待回调函数更新共享数据。我们使用条件变量来等待线程通知,如果 `value` 的值仍然为 0,则线程将等待条件变量的信号。一旦条件变量被通知,我们再次锁定互斥锁来读取更新后的值,并释放互斥锁。
这个示例程序展示了如何在多线程中实现回调函数访问共享资源和数据同步的基本原理。当然,实际情况可能更加复杂,需要更多的同步机制来确保线程安全。
阅读全文
相关推荐
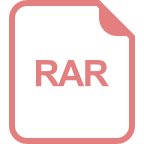
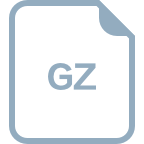
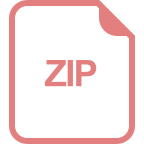
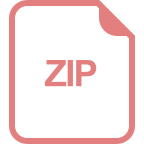
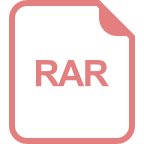
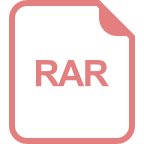
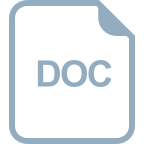
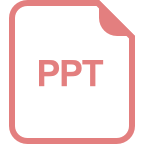
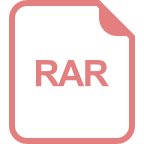
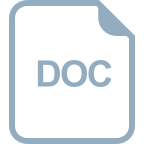
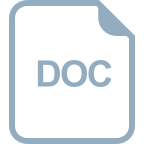
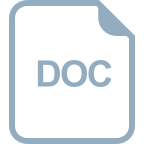
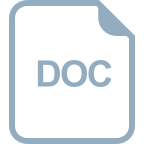
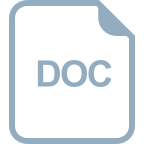
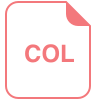
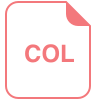