航天器轨迹优化的Python代码
时间: 2023-07-15 08:09:52 浏览: 241
航天器轨迹优化是一个复杂的问题,需要考虑多个因素,如引力、推力、空气阻力等。下面给出一个基于Python的简单示例代码,帮助你了解航天器轨迹优化的大致思路。
首先,我们需要导入必要的库:
```python
import numpy as np
from scipy.integrate import solve_ivp
import matplotlib.pyplot as plt
```
接下来,定义一些常量和初始条件:
```python
# gravitational constant
G = 6.674e-11
# mass of earth
M = 5.972e24
# radius of earth
R = 6.371e6
# initial position and velocity of spacecraft
X0 = np.array([R+500e3, 0, 0])
V0 = np.array([0, 7.9e3, 0])
# thrust acceleration
a_thrust = 1
# time span and step size
t_span = (0, 10000)
dt = 10
```
然后,我们定义一个函数来计算航天器在引力和推力作用下的加速度:
```python
def acceleration(t, state):
x, y, z, vx, vy, vz = state
r = np.sqrt(x**2 + y**2 + z**2)
a_gravity = -G*M/r**2 * np.array([x, y, z])/r
a = a_gravity
if t < 5000:
a += a_thrust * np.array([1, 0, 0])
return a
```
该函数使用了 solve_ivp 函数来数值求解航天器的轨迹:
```python
sol = solve_ivp(acceleration, t_span, np.concatenate([X0, V0]), t_eval=np.arange(0, t_span[1]+dt, dt))
```
最后,我们可以使用 Matplotlib 来绘制航天器的轨迹:
```python
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot(sol.y[0], sol.y[1], sol.y[2])
ax.set_xlabel('X (m)')
ax.set_ylabel('Y (m)')
ax.set_zlabel('Z (m)')
plt.show()
```
完整代码如下:
```python
import numpy as np
from scipy.integrate import solve_ivp
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# gravitational constant
G = 6.674e-11
# mass of earth
M = 5.972e24
# radius of earth
R = 6.371e6
# initial position and velocity of spacecraft
X0 = np.array([R+500e3, 0, 0])
V0 = np.array([0, 7.9e3, 0])
# thrust acceleration
a_thrust = 1
# time span and step size
t_span = (0, 10000)
dt = 10
def acceleration(t, state):
x, y, z, vx, vy, vz = state
r = np.sqrt(x**2 + y**2 + z**2)
a_gravity = -G*M/r**2 * np.array([x, y, z])/r
a = a_gravity
if t < 5000:
a += a_thrust * np.array([1, 0, 0])
return a
sol = solve_ivp(acceleration, t_span, np.concatenate([X0, V0]), t_eval=np.arange(0, t_span[1]+dt, dt))
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot(sol.y[0], sol.y[1], sol.y[2])
ax.set_xlabel('X (m)')
ax.set_ylabel('Y (m)')
ax.set_zlabel('Z (m)')
plt.show()
```
当然,这只是一个简单的示例代码,实际的航天器轨迹优化问题远比这个复杂。如果你需要解决实际问题,建议参考相关文献或咨询专业人士。
阅读全文
相关推荐
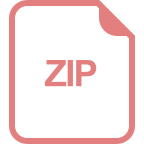
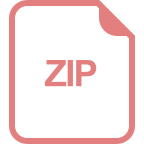
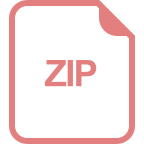
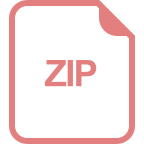
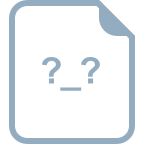
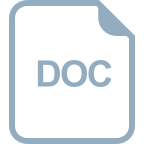
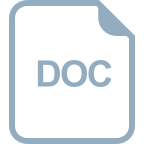
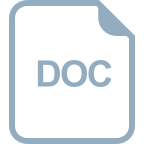
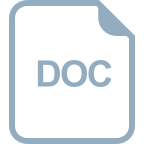
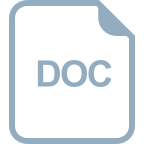
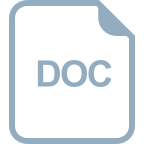
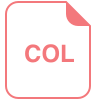
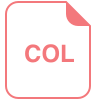
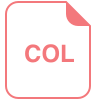
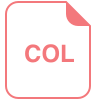
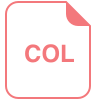