opencv调整图像对比度的函数
时间: 2023-09-24 14:14:00 浏览: 58
OpenCV中可以使用cv::Mat::convertTo()函数来调整图像的对比度。具体的实现方式是将图像中的每个像素值乘以一个比例因子并加上一个偏移值。
以下是一个示例代码:
```
cv::Mat img = cv::imread("example.jpg");
double alpha = 1.5; // 对比度比例因子
int beta = 50; // 亮度偏移值
cv::Mat new_img;
img.convertTo(new_img, -1, alpha, beta);
cv::imshow("Original Image", img);
cv::imshow("New Image", new_img);
```
在上述代码中,我们首先读取了一张名为 "example.jpg" 的图像。然后,我们指定了一个对比度比例因子 alpha 和一个亮度偏移值 beta。接着,我们使用convertTo()函数将原始图像转换为新的图像,并将其存储在new_img中。最后,我们使用imshow()函数显示原始图像和新的图像。
希望这可以帮助到你。
相关问题
opencv 提高图像对比度
要提高图像对比度,可以使用 OpenCV 的 equalizeHist 函数。该函数将直方图均衡化应用于灰度图像,从而增加图像的对比度。以下是一个示例代码:
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg', 0)
# 应用直方图均衡化
img_eq = cv2.equalizeHist(img)
# 显示原始图像和增强后的图像
cv2.imshow('Original Image', img)
cv2.imshow('Enhanced Image', img_eq)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,cv2.imread 函数用于读取图像,第二个参数为 0 表示将图像读取为灰度图像。然后,将 img 传递给 equalizeHist 函数,生成一个增强后的图像 img_eq。最后,使用 cv2.imshow 函数显示原始图像和增强后的图像。
opencv调图像对比度
如何调整?
可以使用 cv2.convertScaleAbs() 函数来调整图像的对比度,具体操作如下:
1. 读入图像并将其转为灰度图像。
2. 计算图像的平均灰度值。
3. 使用公式:new_pixel_value = (pixel_value - mean_gray_value) * contrast_factor + mean_gray_value 来计算每个像素点的新值。
4. 将新的像素值转为无符号8位整数类型,并将其存储在新的图像矩阵中。
其中,contrast_factor 是对比度调整参数。值越大,对比度越高,值越小,对比度越低。
示例代码如下:
import cv2
import numpy as np
# 读入图像并将其转为灰度图像
img = cv2.imread('test.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 计算图像的平均灰度值
mean_gray = np.mean(gray)
# 设置对比度调整参数
contrast_factor = 1.5
# 计算每个像素点的新值
new_gray = cv2.convertScaleAbs(gray, alpha=contrast_factor, beta=-contrast_factor*mean_gray+255)
# 显示原图和调整后的图像
cv2.imshow('Original', gray)
cv2.imshow('Adjusted', new_gray)
cv2.waitKey(0)
cv2.destroyAllWindows()
相关推荐
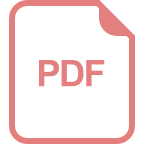
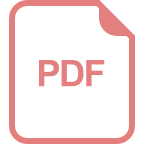
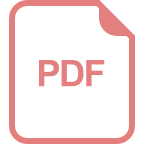
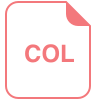
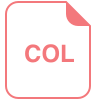
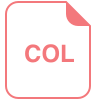
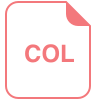
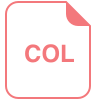







