帮我写一个矩形排版算法
时间: 2024-03-28 10:05:18 浏览: 269
当然可以!下面是一个简单的矩形排版算法:
1. 首先,确定矩形排版的目标区域大小和矩形集合。目标区域可以是一个固定大小的矩形,也可以是一个可变大小的容器。
2. 对于每个要排版的矩形,确定其位置和大小。可以根据具体需求来确定如何选择和放置矩形。
3. 确定矩形的放置顺序。可以按照某种策略来决定放置顺序,例如按照矩形的大小、优先级或其他指标进行排序。
4. 从第一个矩形开始,尝试将其放置在目标区域中。如果与已放置的矩形有重叠,则尝试调整位置或大小,直到找到合适的位置。
5. 继续放置下一个矩形,重复上述步骤,直到所有矩形都被放置完毕。
6. 最后,根据需要进行调整和优化。可以考虑对已排版的矩形进行位置的微调,以获得更好的排版效果。
需要注意的是,这只是一个简单的算法框架,具体实现还需要考虑更多的细节和约束条件。可以根据具体需求进行改进和扩展。希
相关问题
C++2D矩形装箱算法,给定矩形边界大小,和指定间隙距离,多个矩形输入装箱,利用率最高的排版算法代码
这里提供一个基于贪心算法的C++2D矩形装箱算法,可以实现多个矩形的高效排版。
```
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Rect {
int id, w, h, x, y;
Rect(int _id, int _w, int _h) {
id = _id;
w = _w;
h = _h;
x = y = 0;
}
};
bool cmp(Rect a, Rect b) {
if (a.h != b.h) return a.h > b.h;
return a.w > b.w;
}
class Bin {
public:
int w, h;
vector<Rect> rects;
Bin(int _w, int _h) {
w = _w;
h = _h;
}
bool add(Rect rect) {
int x = 0, y = 0;
if (!find_pos(rect, x, y)) return false;
rect.x = x;
rect.y = y;
rects.push_back(rect);
return true;
}
private:
bool find_pos(Rect rect, int& x, int& y) {
sort(rects.begin(), rects.end(), cmp);
for (int i = 0; i < rects.size(); i++) {
if (rect.w <= w - rects[i].x && rect.h <= h - rects[i].y) {
x = rects[i].x;
y = rects[i].y;
for (int j = i + 1; j < rects.size(); j++) {
if (rects[j].y < y + rect.h && rects[j].x < x + rect.w) {
x = max(x, rects[j].x + rects[j].w);
}
}
return true;
} else if (rect.h <= w - rects[i].y && rect.w <= h - rects[i].x) {
swap(w, h);
for (int j = 0; j < rects.size(); j++) {
if (rects[j].y < x + rect.w && rects[j].x < y + rect.h) {
y = max(y, rects[j].y + rects[j].h);
}
}
swap(w, h);
swap(rect.w, rect.h);
swap(x, y);
if (find_pos(rect, x, y)) return true;
}
}
return false;
}
};
bool cmp_area(Rect a, Rect b) {
return a.w * a.h > b.w * b.h;
}
vector<Rect> pack_rects(int w, int h, vector<Rect>& rects) {
vector<Rect> result;
sort(rects.begin(), rects.end(), cmp_area);
Bin bin(w, h);
for (int i = 0; i < rects.size(); i++) {
if (!bin.add(rects[i])) {
cout << "Can not pack rectangle " << rects[i].id << endl;
} else {
result.push_back(rects[i]);
}
}
return result;
}
int main() {
int w = 100, h = 100, gap = 5;
vector<Rect> rects;
rects.push_back(Rect(1, 20, 30));
rects.push_back(Rect(2, 30, 40));
rects.push_back(Rect(3, 25, 25));
rects.push_back(Rect(4, 15, 20));
rects.push_back(Rect(5, 10, 30));
rects.push_back(Rect(6, 10, 10));
rects.push_back(Rect(7, 40, 30));
vector<Rect> result = pack_rects(w - gap * 2, h - gap * 2, rects);
for (int i = 0; i < result.size(); i++) {
cout << "Rectangle " << result[i].id << " (" << result[i].w << ", " << result[i].h << ") at (" << result[i].x + gap << ", " << result[i].y + gap << ")" << endl;
}
return 0;
}
```
这个算法的实现思想是先按照面积大小对矩形进行排序,然后按照贪心策略依次将矩形装入最佳位置。装箱时,按照矩形高度和宽度的大小排序,并从大到小遍历已经排好的矩形,寻找可以放置当前矩形的最佳位置。
具体实现中,我们使用 `Bin` 类来表示一个装箱盒子,其中包含了当前盒子的宽度和高度,以及已经放置的矩形列表。对于每个 `Rect` 矩形,我们从已经放置的矩形中寻找可以放置它的最佳位置,并将它放置在这个位置上。如果找不到合适的位置,则说明当前盒子已经装不下这个矩形了。
最终,我们返回已经放置的矩形列表,这些矩形的坐标信息都已经被计算好了。
c#二维矩形优化排版 算法代码
下面是一个简单的 C# 二维矩形优化排版算法的示例代码:
```csharp
public class Rectangle
{
public int Width { get; set; }
public int Height { get; set; }
public Rectangle(int width, int height)
{
Width = width;
Height = height;
}
}
public static class RectanglePacker
{
public static List<Rectangle> Pack(List<Rectangle> rectangles, int containerWidth, int containerHeight)
{
List<Rectangle> packedRectangles = new List<Rectangle>();
int currentRowY = 0;
int currentRowHeight = 0;
foreach (Rectangle rectangle in rectangles)
{
if (currentRowY + rectangle.Height > containerHeight)
{
currentRowY = 0;
currentRowHeight = 0;
}
if (currentRowHeight + rectangle.Height <= containerHeight)
{
rectangle.Width = Math.Min(rectangle.Width, containerWidth);
rectangle.Height = Math.Min(rectangle.Height, containerHeight - currentRowHeight);
packedRectangles.Add(rectangle);
currentRowY += rectangle.Height;
currentRowHeight += rectangle.Height;
}
}
return packedRectangles;
}
}
```
使用方法:
```csharp
List<Rectangle> rectangles = new List<Rectangle>();
rectangles.Add(new Rectangle(100, 50));
rectangles.Add(new Rectangle(75, 75));
rectangles.Add(new Rectangle(50, 100));
rectangles.Add(new Rectangle(25, 25));
List<Rectangle> packedRectangles = RectanglePacker.Pack(rectangles, 200, 200);
foreach (Rectangle rectangle in packedRectangles)
{
Console.WriteLine("Width: {0}, Height: {1}", rectangle.Width, rectangle.Height);
}
```
这个算法的基本思路是,从上到下依次放置矩形,如果当前行放不下某个矩形,则换行放置。在放置矩形时,会尽量使用矩形的原始宽度和高度,但是如果当前行放不下该矩形,则会将宽度调整为容器的宽度,高度调整为当前行剩余的高度。
阅读全文
相关推荐
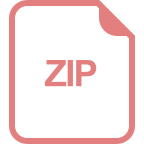
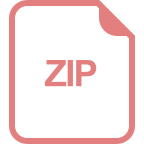
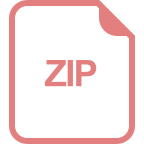
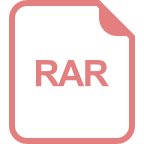
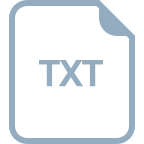
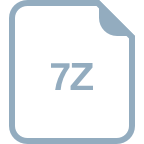
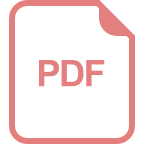
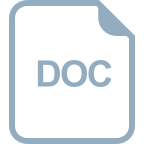
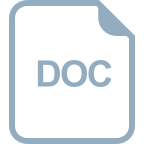



