用c语言修改这段代码,让这段代码能够输出字符串中数字的个数#include <stdio.h> #include <stdlib.h> #include<string.h> int number(char str[]) {int n,i,k=0; n=strlen(str); for(i=0;i<n;i++){ if(str[i]>=0&&str[i]<=9) k++; }return k; } int main() {char str[20]; int a; gets(str); a=number(str); printf("%d",a); return 0; }
时间: 2023-06-09 16:07:48 浏览: 215
可以使用以下代码实现:
```c
#include <stdio.h>
int main() {
char str[100];
int num_count = 0;
printf("请输入一个字符串:\n");
scanf("%s", str);
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] >= '0' && str[i] <= '9') { // 判断是否为数字字符
num_count++;
}
}
printf("字符串中数字的个数为: %d\n", num_count);
return 0;
}
```
首先定义一个字符数组 `str` 和一个整型变量 `num_count`,分别用来存储输入的字符串和数字的个数。
使用 `scanf` 函数从控制台输入一个字符串。
使用 `for` 循环遍历字符串,判断每一个字符是否为数字字符,如果是则将数字的个数加一。
最后输出数字的个数。
需要注意的是,判断字符是否为数字字符的条件是该字符的 ASCII 码值在 `'0'` 和 `'9'` 之间。
相关问题
#include <stdio.h> #include <stdlib.h> void PutRes(void); int fun(char *); int fun (char *p) 接着这个编写C语言:通过函数统计字符串中数字字符的个数,返回结果。在主函数中输入一串字符
代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
int countDigits(char*);
int main() {
char str[100];
printf("Enter a string: ");
gets(str);
int count = countDigits(str);
printf("The number of digits in the string is: %d\n", count);
return 0;
}
int countDigits(char *str) {
int count = 0;
while (*str != '\0') {
if (*str >= '0' && *str <= '9') {
count++;
}
str++;
}
return count;
}
```
注意:使用 `gets()` 函数时要小心,因为它没有限制输入的长度,可能会导致缓冲区溢出。更好的选择是使用 `fgets()` 函数。
#include<stdlib.h> #include <stdio.h> #include <math.h> #define PI 3.1415926 double f(double x) { return exp(x)cos(4x); // 定义被积函数 } double simpson(double a, double b) { int n = 1000; // 分割区间的个数 double h = (b - a) / n; double sum = 0; for (int i = 0; i < n; i++) { double x1 = a + i * h; double x2 = a + (i + 1) * h; sum += f(x1) + 4 * f((x1 + x2) / 2) + f(x2); } return sum * h / 6; } int main() { double a = 0, b = PI; // 积分区间 [a, b] double result = simpson(a, b); printf("The result is: %lf\n", result); system("pause"); return 0; }写出计算这个代码的函数调用次数的C语言代码
可以在原代码基础上加入计数器,用于统计函数调用的次数,代码如下:
```
#include<stdlib.h>
#include<stdio.h>
#include<math.h>
#define PI 3.1415926
int f_call_count = 0; // 函数f(x)的调用次数计数器
double f(double x) {
f_call_count++; // 每次调用函数f(x)时计数器加1
return exp(x)cos(4x); // 定义被积函数
}
double simpson(double a, double b) {
int n = 1000; // 分割区间的个数
double h = (b - a) / n;
double sum = 0;
for (int i = 0; i < n; i++) {
double x1 = a + i * h;
double x2 = a + (i + 1) * h;
sum += f(x1) + 4 * f((x1 + x2) / 2) + f(x2);
}
return sum * h / 6;
}
int main() {
double a = 0, b = PI; // 积分区间 [a, b]
double result = simpson(a, b);
printf("The result is: %lf\n", result);
printf("The function f(x) is called %d times.\n", f_call_count);
system("pause");
return 0;
}
```
运行程序后,会输出计算结果和函数 `f(x)` 的调用次数。
阅读全文
相关推荐
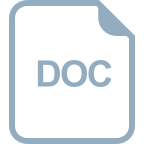
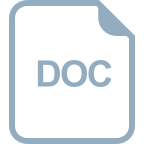
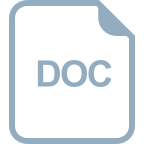







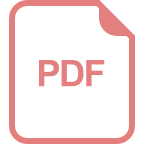





