使用ehcache实现购物车
时间: 2024-06-08 22:09:48 浏览: 175
购物车是一个经典的缓存场景,可以使用 Ehcache 实现。以下是一个简单的购物车实现:
1. 首先,定义一个 CartItem 类,表示购物车中的商品项,包含商品 ID、商品名称、商品数量、商品单价等信息。
```java
public class CartItem {
private Long itemId;
private String itemName;
private Integer quantity;
private BigDecimal price;
// 省略 getter 和 setter 方法
}
```
2. 定义一个 Cart 类,表示购物车。在 Cart 类中,使用 Ehcache 缓存购物车中的商品项,以便在多个请求之间共享购物车信息。
```java
public class Cart {
private CacheManager cacheManager;
private Cache<Long, CartItem> cartCache;
public Cart() {
cacheManager = CacheManagerBuilder.newCacheManagerBuilder().build();
cacheManager.init();
cartCache = cacheManager.createCache("cartCache",
CacheConfigurationBuilder.newCacheConfigurationBuilder(Long.class, CartItem.class,
ResourcePoolsBuilder.heap(100)));
}
public void addItem(CartItem item) {
Long itemId = item.getItemId();
CartItem existingItem = cartCache.get(itemId);
if (existingItem != null) {
existingItem.setQuantity(existingItem.getQuantity() + item.getQuantity());
} else {
cartCache.put(itemId, item);
}
}
public void removeItem(Long itemId) {
cartCache.remove(itemId);
}
public List<CartItem> getItems() {
return new ArrayList<>(cartCache.values());
}
public void clear() {
cartCache.clear();
}
public void close() {
cacheManager.close();
}
}
```
在 Cart 类中,使用 Ehcache 缓存管理器创建一个名为 "cartCache" 的缓存,缓存的键为商品 ID,值为 CartItem 对象。addItem() 方法向购物车中添加商品项,如果商品项已存在,则增加商品数量;否则,将商品项添加到购物车中。removeItem() 方法从购物车中删除指定商品项。getItems() 方法返回购物车中的所有商品项。clear() 方法清空购物车。close() 方法关闭 Ehcache 缓存管理器。
3. 在 Web 应用程序中使用购物车。在 Web 应用程序中,通常将购物车保存在 HttpSession 中,以便在多个请求之间共享购物车信息。以下是一个简单的 Servlet 示例代码:
```java
public class ShoppingCartServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String itemIdStr = request.getParameter("itemId");
String itemName = request.getParameter("itemName");
String quantityStr = request.getParameter("quantity");
String priceStr = request.getParameter("price");
Long itemId = Long.parseLong(itemIdStr);
Integer quantity = Integer.parseInt(quantityStr);
BigDecimal price = new BigDecimal(priceStr);
CartItem item = new CartItem(itemId, itemName, quantity, price);
HttpSession session = request.getSession(true);
Cart cart = (Cart) session.getAttribute("cart");
if (cart == null) {
cart = new Cart();
session.setAttribute("cart", cart);
}
cart.addItem(item);
response.sendRedirect("cart.jsp");
}
}
```
在这个示例中,从请求参数中获取商品 ID、商品名称、商品数量和商品单价,创建一个 CartItem 对象。然后,获取 HttpSession 对象,从中获取购物车对象。如果购物车对象不存在,则创建一个新的购物车对象,并将其保存到 HttpSession 中。然后,将商品项添加到购物车中,并重定向到购物车页面。
在购物车页面(cart.jsp)中,可以通过调用 Cart 类的 getItems() 方法获取购物车中的所有商品项,并显示在页面上。
```jsp
<%@ page contentType="text/html;charset=UTF-8" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>购物车</title>
</head>
<body>
<table>
<tr>
<th>商品ID</th>
<th>商品名称</th>
<th>商品数量</th>
<th>商品单价</th>
<th>小计</th>
</tr>
<c:forEach var="item" items="${cart.items}">
<tr>
<td>${item.itemId}</td>
<td>${item.itemName}</td>
<td>${item.quantity}</td>
<td>${item.price}</td>
<td>${item.quantity * item.price}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
```
这个示例代码演示了如何使用 Ehcache 实现购物车功能。在实际应用中,购物车的功能可能更加复杂,例如支持优惠券、促销活动等。但是,使用 Ehcache 缓存购物车信息是一个常见的做法,可以提高应用程序的性能和可扩展性。
阅读全文
相关推荐


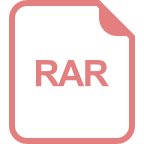



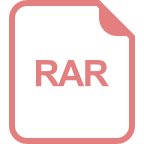


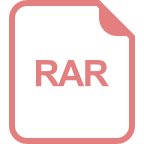
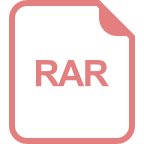
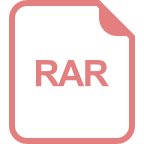
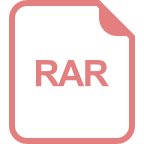


