format {} 格式化选项详解
时间: 2024-03-08 09:24:02 浏览: 21
在Python中,{}是一个占位符,可以在字符串中用来表示需要填充的值。在使用{}时,可以使用格式化选项来指定填充的方式和格式。以下是常见的格式化选项及其含义:
1. 填充和对齐
- {:} 不进行任何填充和对齐操作,默认为左对齐
- {:<n} 左对齐,用空格填充至n位
- {:>n} 右对齐,用空格填充至n位
- {:^n} 居中对齐,用空格填充至n位
- {:0n} 不考虑对齐,用0填充至n位
示例:
```python
print("{:10}".format("hello")) # 左对齐,用空格填充至10位
print("{:>10}".format("hello")) # 右对齐,用空格填充至10位
print("{:^10}".format("hello")) # 居中对齐,用空格填充至10位
print("{:0>10}".format("hello")) # 不考虑对齐,用0填充至10位
```
输出:
```
hello
hello
hello
00000hello
```
2. 数字格式化
- {:d} 默认整数格式,不含小数点
- {:b} 二进制整数格式
- {:o} 八进制整数格式
- {:x} 十六进制整数格式(小写字母)
- {:X} 十六进制整数格式(大写字母)
- {:n.mf} 浮点数格式,n表示总位数,m表示小数位数
示例:
```python
print("{:d}".format(123)) # 默认整数格式
print("{:b}".format(123)) # 二进制整数格式
print("{:o}".format(123)) # 八进制整数格式
print("{:x}".format(123)) # 十六进制整数格式(小写字母)
print("{:X}".format(123)) # 十六进制整数格式(大写字母)
print("{:.2f}".format(123.456)) # 浮点数格式,保留2位小数
```
输出:
```
123
1111011
173
7b
7B
123.46
```
3. 字符串格式化
- {:s} 默认字符串格式
- {:n.m}s 字符串格式,n表示总位数,m表示截取的字符数
示例:
```python
print("{:s}".format("hello")) # 默认字符串格式
print("{:7.3s}".format("hello")) # 字符串格式,总位数为7,截取3个字符
```
输出:
```
hello
hel
```
4. 变量替换
- {var} 变量替换,var表示变量名
示例:
```python
name = "Tom"
age = 18
print("My name is {name}, and I'm {age} years old.".format(name=name, age=age))
```
输出:
```
My name is Tom, and I'm 18 years old.
```
5. 混合使用
示例:
```python
name = "Tom"
age = 18
print("{0:^10} | {1:<10} | {2:>10}".format("Index", "Name", "Age"))
print("{0:^10} | {1:<10.3s} | {2:>10d}".format(1, name, age))
```
输出:
```
Index | Name | Age
1 | Tom | 18
```
以上就是{}格式化选项的详细解释。在实际应用中,可以根据需要灵活使用不同的格式化选项,使输出更加美观和易读。
相关推荐
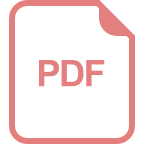
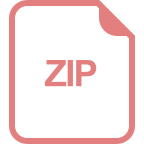
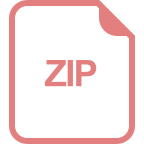














