根据如下代码,选项中描述错误的是( )。 a=3 while a>0: a-=1 print(a,end="") A. a -= 1 可由 a = a-1 实现 B。条件 a>0 如果修改为 a<0 程序执行会进入死循环 C. 这段代码的输出内容为 210 D. 使用 while 保留字可创建无限循
时间: 2023-03-04 15:21:07 浏览: 334
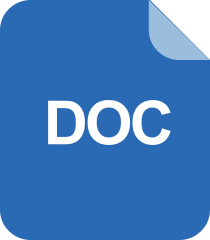
2019_2020学年高中数学第一章算法初步1.2.3循环语句限时规范训练新人教A版必修3
选项 B 描述错误。
原代码中,while 循环的条件是 a>0,表示只要 a 大于 0 就会继续执行循环体内的代码。如果将条件修改为 a<0,那么循环条件永远不会满足,循环体内的代码永远不会执行,程序也就不会进入死循环。
选项 A 描述正确,a -= 1 可以由 a = a-1 实现,它表示将 a 的值减 1。
选项 C 描述正确,代码的输出内容为 210。循环体内的代码会在每次循环中将 a 的值减 1,并输出 a 的值,因此输出的内容为 2、1、0。
选项 D 描述正确,使用 while 保留字可以创建无限循环,例如 while True:。
阅读全文
相关推荐
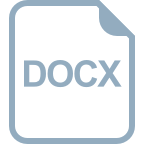
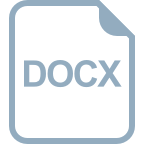
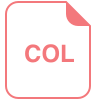
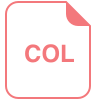
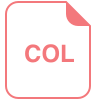
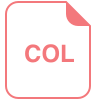
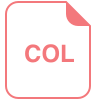
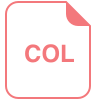
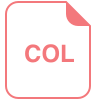
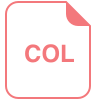
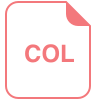
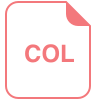
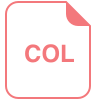




