用C语言利用稀疏矩阵的链式存储实现稀疏矩阵的加,减,乘,转置等简单运算
时间: 2024-03-17 16:47:46 浏览: 16
以下是用C语言实现稀疏矩阵的加、减、乘、转置等简单运算的示例代码,利用稀疏矩阵的链式存储方式实现:
```
#include <stdio.h>
#include <stdlib.h>
// 定义稀疏矩阵结构体
typedef struct sparse_matrix {
int row; // 矩阵行数
int col; // 矩阵列数
int num; // 矩阵非零元素个数
struct node *row_head; // 行链表头指针
struct node *col_head; // 列链表头指针
} SparseMatrix;
// 定义稀疏矩阵节点结构体
typedef struct node {
int row; // 节点所在行
int col; // 节点所在列
int val; // 节点元素值
struct node *row_next; // 行链表下一节点指针
struct node *col_next; // 列链表下一节点指针
} Node;
// 创建稀疏矩阵
SparseMatrix* create_sparse_matrix(int row, int col) {
SparseMatrix *matrix = (SparseMatrix*)malloc(sizeof(SparseMatrix));
matrix->row = row;
matrix->col = col;
matrix->num = 0;
matrix->row_head = NULL;
matrix->col_head = NULL;
return matrix;
}
// 添加节点到矩阵中
void add_node_to_matrix(SparseMatrix *matrix, int row, int col, int val) {
Node *node = (Node*)malloc(sizeof(Node));
node->row = row;
node->col = col;
node->val = val;
node->row_next = NULL;
node->col_next = NULL;
// 将节点插入到行链表中
Node *row_head = matrix->row_head;
if (!row_head || row_head->row > row) {
matrix->row_head = node;
node->row_next = row_head;
} else {
Node *prev = row_head;
while (prev->row_next && prev->row_next->row <= row) {
prev = prev->row_next;
}
node->row_next = prev->row_next;
prev->row_next = node;
}
// 将节点插入到列链表中
Node *col_head = matrix->col_head;
if (!col_head || col_head->col > col) {
matrix->col_head = node;
node->col_next = col_head;
} else {
Node *prev = col_head;
while (prev->col_next && prev->col_next->col <= col) {
prev = prev->col_next;
}
node->col_next = prev->col_next;
prev->col_next = node;
}
matrix->num++;
}
// 打印稀疏矩阵
void print_sparse_matrix(SparseMatrix *matrix) {
printf("Sparse Matrix:\n");
printf("Row: %d, Col: %d, Num: %d\n", matrix->row, matrix->col, matrix->num);
Node *row_head = matrix->row_head;
for (int i = 0; i < matrix->row; i++) {
Node *cur = row_head;
for (int j = 0; j < matrix->col; j++) {
if (cur && cur->col == j) {
printf("%d ", cur->val);
cur = cur->row_next;
} else {
printf("0 ");
}
}
printf("\n");
if (row_head) {
row_head = row_head->row_next;
}
}
}
// 稀疏矩阵加法
SparseMatrix* sparse_matrix_add(SparseMatrix *matrix1, SparseMatrix *matrix2) {
if (matrix1->row != matrix2->row || matrix1->col != matrix2->col) {
return NULL;
}
SparseMatrix *result = create_sparse_matrix(matrix1->row, matrix1->col);
Node *node1 = matrix1->row_head;
Node *node2 = matrix2->row_head;
while (node1 || node2) {
if (!node2 || (node1 && node1->col < node2->col)) {
add_node_to_matrix(result, node1->row, node1->col, node1->val);
node1 = node1->row_next;
} else if (!node1 || (node2 && node2->col < node1->col)) {
add_node_to_matrix(result, node2->row, node2->col, node2->val);
node2 = node2->row_next;
} else {
add_node_to_matrix(result, node1->row, node1->col, node1->val + node2->val);
node1 = node1->row_next;
node2 = node2->row_next;
}
}
return result;
}
// 稀疏矩阵减法
SparseMatrix* sparse_matrix_subtract(SparseMatrix *matrix1, SparseMatrix *matrix2) {
if (matrix1->row != matrix2->row || matrix1->col != matrix2->col) {
return NULL;
}
SparseMatrix *result = create_sparse_matrix(matrix1->row, matrix1->col);
Node *node1 = matrix1->row_head;
Node *node2 = matrix2->row_head;
while (node1 || node2) {
if (!node2 || (node1 && node1->col < node2->col)) {
add_node_to_matrix(result, node1->row, node1->col, node1->val);
node1 = node1->row_next;
} else if (!node1 || (node2 && node2->col < node1->col)) {
add_node_to_matrix(result, node2->row, node2->col, -node2->val);
node2 = node2->row_next;
} else {
add_node_to_matrix(result, node1->row, node1->col, node1->val - node2->val);
node1 = node1->row_next;
node2 = node2->row_next;
}
}
return result;
}
// 稀疏矩阵乘法
SparseMatrix* sparse_matrix_multiply(SparseMatrix *matrix1, SparseMatrix *matrix2) {
if (matrix1->col != matrix2->row) {
return NULL;
}
SparseMatrix *result = create_sparse_matrix(matrix1->row, matrix2->col);
Node *node1 = matrix1->row_head;
while (node1) {
Node *node2 = matrix2->col_head;
while (node2) {
if (node1->col == node2->row) {
int sum = 0;
Node *cur1 = node1;
Node *cur2 = node2;
while (cur1 && cur2 && cur1->col == node1->col && cur2->row == node2->row) {
if (cur1->row < cur2->col) {
cur1 = cur1->row_next;
} else if (cur1->row > cur2->col) {
cur2 = cur2->col_next;
} else {
sum += cur1->val * cur2->val;
cur1 = cur1->row_next;
cur2 = cur2->col_next;
}
}
add_node_to_matrix(result, node1->row, node2->col, sum);
}
node2 = node2->col_next;
}
node1 = node1->row_next;
}
return result;
}
// 稀疏矩阵转置
SparseMatrix* sparse_matrix_transpose(SparseMatrix *matrix) {
SparseMatrix *result = create_sparse_matrix(matrix->col, matrix->row);
Node *node = matrix->row_head;
while (node) {
add_node_to_matrix(result, node->col, node->row, node->val);
node = node->row_next;
}
return result;
}
// 测试稀疏矩阵加、减、乘、转置
int main() {
SparseMatrix *matrix1 = create_sparse_matrix(3, 3);
add_node_to_matrix(matrix1, 0, 0, 1);
add_node_to_matrix(matrix1, 0, 1, 2);
add_node_to_matrix(matrix1, 1, 0, 3);
add_node_to_matrix(matrix1, 1, 1, 4);
add_node_to_matrix(matrix1, 2, 2, 5);
print_sparse_matrix(matrix1);
SparseMatrix *matrix2 = create_sparse_matrix(3, 3);
add_node_to_matrix(matrix2, 0, 0, 6);
add_node_to_matrix(matrix2, 0, 1, 7);
add_node_to_matrix(matrix2, 1, 0, 8);
add_node_to_matrix(matrix2, 1, 1, 9);
add_node_to_matrix(matrix2, 2, 2, 10);
print_sparse_matrix(matrix2);
SparseMatrix *result = sparse_matrix_add(matrix1, matrix2);
print_sparse_matrix(result);
result = sparse_matrix_subtract(matrix1, matrix2);
print_sparse_matrix(result);
result = sparse_matrix_multiply(matrix1, matrix2);
print_sparse_matrix(result);
result = sparse_matrix_transpose(matrix1);
print_sparse_matrix(result);
return 0;
}
```
注意:以上示例代码仅供参考,具体实现可能还需要根据实际情况进行调整。
相关推荐
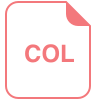
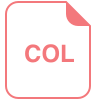
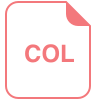

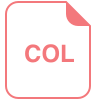









