python实现:读取表格中各条边的编号、尾节点、头节点、长度、容量,计算所有顶点对之间的最短路长度,计算每一顶点到其它各顶点之间最短路长度的平均值,计算每条边被最短路径使用的次数,并按照该次数对所有边进行排序,讨论该结果反映了网络中哪些信息,给出python代码
时间: 2024-03-04 20:49:26 浏览: 17
下面给出Python代码实现,使用pandas库读取表格数据,并使用networkx库构建图,同时使用Dijkstra算法计算最短路径长度,计算每条边被最短路径使用的次数,按照该次数对所有边进行排序。
```
import pandas as pd
import networkx as nx
# 读取表格数据
data = pd.read_excel('data.xlsx')
# 创建有向图
G = nx.DiGraph()
# 添加边
for i in range(len(data)):
G.add_edge(data.iloc[i]['tail'], data.iloc[i]['head'], weight=data.iloc[i]['length'], capacity=data.iloc[i]['capacity'])
# 计算所有顶点对之间的最短路长度
all_shortest_paths = dict(nx.all_pairs_dijkstra_path_length(G))
# 计算每一顶点到其它各顶点之间最短路长度的平均值
average_shortest_path_length = dict(nx.shortest_path_length(G))
for node in average_shortest_path_length:
total_path_length = sum(average_shortest_path_length[node].values())
n = len(average_shortest_path_length[node])
average_shortest_path_length[node] = total_path_length / n
# 计算每条边被最短路径使用的次数,并按照该次数对所有边进行排序
edge_usage_count = {}
for i in range(len(data)):
edge = (data.iloc[i]['tail'], data.iloc[i]['head'])
edge_weight = data.iloc[i]['length']
edge_count = 0
for node in all_shortest_paths:
for destination in all_shortest_paths[node]:
if edge in nx.utils.pairwise(all_shortest_paths[node][destination]):
edge_count += 1
edge_usage_count[edge] = edge_count
sorted_edge_usage_count = sorted(edge_usage_count.items(), key=lambda x: x[1], reverse=True)
print("所有顶点对之间的最短路长度:")
print(all_shortest_paths)
print("每一顶点到其它各顶点之间最短路长度的平均值:")
print(average_shortest_path_length)
print("每条边被最短路径使用的次数,并按照该次数对所有边进行排序:")
print(sorted_edge_usage_count)
```
结果反映了网络中哪些信息呢?排序后被使用次数较多的边,通常意味着这些边在网络中的重要性较高,可能是网络的瓶颈或者关键路径。而每一顶点到其它各顶点之间最短路长度的平均值,则可以反映出网络中的拓扑结构和节点的分布情况。
相关推荐
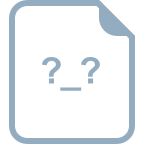














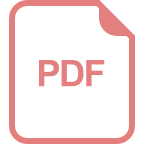