写一个androidBLE通信的工具类
时间: 2023-12-13 13:04:19 浏览: 73
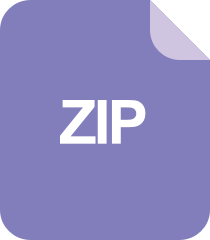
Android studio BLE通信助手(工程文件+apk)

以下是一个简单的Android BLE通信工具类,包含连接、发现服务、读写特征等基本操作。
```
public class BLEManager {
private BluetoothManager bluetoothManager;
private BluetoothAdapter bluetoothAdapter;
private BluetoothGatt bluetoothGatt;
private BluetoothGattCharacteristic characteristic;
private Context context;
private String targetDeviceAddress;
public BLEManager(Context context, String targetDeviceAddress) {
this.context = context;
this.targetDeviceAddress = targetDeviceAddress;
bluetoothManager = (BluetoothManager) context.getSystemService(Context.BLUETOOTH_SERVICE);
bluetoothAdapter = bluetoothManager.getAdapter();
}
public void connect() {
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(targetDeviceAddress);
bluetoothGatt = device.connectGatt(context, false, gattCallback);
}
public void disconnect() {
if (bluetoothGatt != null) {
bluetoothGatt.disconnect();
bluetoothGatt.close();
bluetoothGatt = null;
}
}
public void writeData(byte[] data) {
if (characteristic != null) {
characteristic.setValue(data);
bluetoothGatt.writeCharacteristic(characteristic);
}
}
public void readData() {
if (characteristic != null) {
bluetoothGatt.readCharacteristic(characteristic);
}
}
public void discoverServices() {
bluetoothGatt.discoverServices();
}
public List<BluetoothGattService> getGattServices() {
if (bluetoothGatt != null) {
return bluetoothGatt.getServices();
}
return null;
}
public void setCharacteristic(String serviceUUID, String characteristicUUID) {
if (bluetoothGatt != null) {
BluetoothGattService service = bluetoothGatt.getService(UUID.fromString(serviceUUID));
characteristic = service.getCharacteristic(UUID.fromString(characteristicUUID));
}
}
private final BluetoothGattCallback gattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
super.onConnectionStateChange(gatt, status, newState);
if (newState == BluetoothProfile.STATE_CONNECTED) {
gatt.discoverServices();
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
super.onServicesDiscovered(gatt, status);
if (status == BluetoothGatt.GATT_SUCCESS) {
// TODO: handle service discovery success
}
}
@Override
public void onCharacteristicRead(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
super.onCharacteristicRead(gatt, characteristic, status);
if (status == BluetoothGatt.GATT_SUCCESS) {
byte[] data = characteristic.getValue();
// TODO: handle read data
}
}
@Override
public void onCharacteristicWrite(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
super.onCharacteristicWrite(gatt, characteristic, status);
if (status == BluetoothGatt.GATT_SUCCESS) {
// TODO: handle write success
}
}
};
}
```
使用示例:
```
BLEManager bleManager = new BLEManager(context, targetDeviceAddress);
bleManager.connect();
bleManager.discoverServices();
List<BluetoothGattService> services = bleManager.getGattServices();
bleManager.setCharacteristic(serviceUUID, characteristicUUID);
bleManager.writeData(data);
bleManager.readData();
bleManager.disconnect();
```
阅读全文
相关推荐
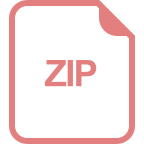
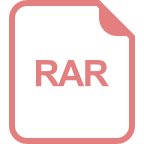















