用c++编写一个打飞机小游戏
时间: 2023-08-20 08:07:09 浏览: 44
好的,以下是一个简单的打飞机小游戏的C++代码:
```c++
#include<iostream>
#include<Windows.h>
#include<conio.h>
#include<time.h>
using namespace std;
const int width = 40; //游戏边框宽度
const int height = 20; //游戏边框高度
int x, y; //飞机位置坐标
int ex, ey; //敌机位置坐标
int bulletx, bullety; //子弹位置坐标
int score; //得分
void gotoxy(int x, int y) //光标定位函数
{
COORD pos;
pos.X = x;
pos.Y = y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void drawBorder() //画游戏边框
{
for (int i = 0; i < height; i++) {
gotoxy(0, i);
cout << "|";
gotoxy(width, i);
cout << "|";
}
for (int i = 0; i < width; i++) {
gotoxy(i, 0);
cout << "-";
gotoxy(i, height);
cout << "-";
}
}
void drawPlane() //画飞机
{
gotoxy(x, y);
cout << " /\\ ";
gotoxy(x, y + 1);
cout << " / \\ ";
gotoxy(x, y + 2);
cout << "/____\\";
}
void drawEnemy() //画敌机
{
gotoxy(ex, ey);
cout << " /\\ ";
gotoxy(ex, ey + 1);
cout << " / \\ ";
gotoxy(ex, ey + 2);
cout << "/____\\";
}
void drawBullet() //画子弹
{
gotoxy(bulletx, bullety);
cout << "|";
}
void movePlane() //移动飞机
{
if (_kbhit()) { //判断是否有按键
char key = _getch(); //获取按键
switch (key) {
case 'a': //向左移动
if (x > 1) {
x--;
}
break;
case 'd': //向右移动
if (x < width - 7) {
x++;
}
break;
case 'w': //向上移动
if (y > 1) {
y--;
}
break;
case 's': //向下移动
if (y < height - 3) {
y++;
}
break;
case ' ': //发射子弹
bulletx = x + 2;
bullety = y - 1;
break;
}
}
}
void moveEnemy() //移动敌机
{
ey++;
if (ey >= height - 2) { //敌机飞出屏幕
ey = 1;
ex = rand() % (width - 5) + 1; //重新生成敌机位置
}
}
void moveBullet() //移动子弹
{
if (bullety > 0) {
bullety--;
}
else { //子弹飞出屏幕
bulletx = 0;
bullety = 0;
}
}
void checkHit() //检测碰撞
{
if (bulletx >= ex && bulletx <= ex + 4 && bullety == ey + 2) { //子弹击中敌机
score += 10; //得分加10分
bulletx = 0;
bullety = 0;
ex = rand() % (width - 5) + 1; //重新生成敌机位置
ey = 1;
}
if ((x >= ex && x <= ex + 4 && y >= ey && y <= ey + 2) || (x + 4 >= ex && x + 4 <= ex + 4 && y >= ey && y <= ey + 2)) { //飞机撞上敌机
gotoxy(width / 2 - 4, height / 2);
cout << "Game Over!";
gotoxy(width / 2 - 6, height / 2 + 1);
cout << "Your Score: " << score;
Sleep(3000);
exit(0);
}
}
void showScore() //显示得分
{
gotoxy(width - 10, height + 1);
cout << "Score: " << score;
}
int main()
{
srand((unsigned)time(NULL)); //初始化随机数生成器
x = width / 2 - 3;
y = height - 4;
ex = rand() % (width - 5) + 1;
ey = 1;
score = 0;
while (true) {
system("cls"); //清屏
drawBorder();
drawPlane();
drawEnemy();
drawBullet();
movePlane();
moveEnemy();
moveBullet();
checkHit();
showScore();
Sleep(50); //延时50毫秒
}
return 0;
}
```
该游戏使用了Windows.h库和conio.h库,需要在Windows系统下运行。运行时,玩家可以使用键盘上的“a”、“d”、“w”、“s”来控制飞机的移动,使用空格键来发射子弹,避免被敌机撞击。游戏中,敌机会不断地从屏幕上方飞过来,玩家需要及时发射子弹来击中敌机,得分才能不断增加。若敌机撞到飞机,游戏结束,显示得分。
相关推荐
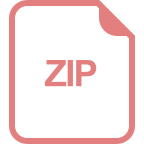
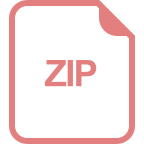
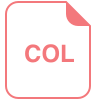
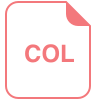
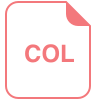
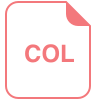
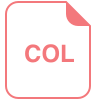









