ffmpeg RTP拉取PCM音频流带啊
时间: 2023-07-06 07:44:04 浏览: 60
可以使用ffmpeg命令行工具拉取RTP传输的PCM音频流,例如:
```
ffmpeg -protocol_whitelist "file,rtp,udp" -i "rtp://ip_address:port" -acodec pcm_s16le output.wav
```
其中,`ip_address` 和 `port` 分别为RTP音频流的IP地址和端口号,`-acodec pcm_s16le` 指定输出音频流的编码格式为PCM,`output.wav` 为输出文件名。可以根据需要修改输出文件名和编码格式参数。
相关问题
ffmpeg RTP拉取PCM音频流C代码
可以使用FFmpeg的C API来实现RTP拉取PCM音频流的功能。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <unistd.h>
#include <pthread.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <libavutil/avutil.h>
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
#define RTP_HEADER_SIZE 12
typedef struct {
AVFormatContext *fmt_ctx;
AVCodecContext *codec_ctx;
AVPacket *pkt;
int audio_stream_index;
int sockfd;
struct sockaddr_in addr;
} RTPContext;
static void *recv_rtp_packets(void *arg)
{
RTPContext *ctx = (RTPContext *)arg;
uint8_t buffer[4096];
int n;
while (1) {
n = recvfrom(ctx->sockfd, buffer, sizeof(buffer), 0, NULL, NULL);
if (n <= 0) {
break;
}
av_packet_from_data(ctx->pkt, buffer + RTP_HEADER_SIZE, n - RTP_HEADER_SIZE);
avcodec_send_packet(ctx->codec_ctx, ctx->pkt);
while (avcodec_receive_frame(ctx->codec_ctx, ctx->fmt_ctx->streams[ctx->audio_stream_index]->codecpar) == 0) {
// 处理音频帧
}
}
return NULL;
}
int main(int argc, char *argv[])
{
if (argc < 3) {
fprintf(stderr, "Usage: %s <ip_address> <port>\n", argv[0]);
exit(1);
}
av_register_all();
avformat_network_init();
RTPContext ctx = {0};
int ret;
// 打开RTP流
avformat_open_input(&ctx.fmt_ctx, "rtp://", NULL, NULL);
av_dict_set(&ctx.fmt_ctx->metadata, "rtpflags", "listen", 0);
av_dict_set(&ctx.fmt_ctx->metadata, "protocol_whitelist", "udp,rtp", 0);
av_dict_set(&ctx.fmt_ctx->metadata, "listen_timeout", "0", 0);
// 添加音频流
AVStream *audio_stream = avformat_new_stream(ctx.fmt_ctx, NULL);
audio_stream->codecpar->codec_type = AVMEDIA_TYPE_AUDIO;
audio_stream->codecpar->codec_id = AV_CODEC_ID_PCM_S16LE;
audio_stream->codecpar->channels = 1;
audio_stream->codecpar->sample_rate = 8000;
avformat_write_header(ctx.fmt_ctx, NULL);
// 打开解码器
ctx.codec_ctx = avcodec_alloc_context3(NULL);
avcodec_parameters_to_context(ctx.codec_ctx, audio_stream->codecpar);
avcodec_open2(ctx.codec_ctx, avcodec_find_decoder(AV_CODEC_ID_PCM_S16LE), NULL);
// 创建RTP套接字
ctx.sockfd = socket(AF_INET, SOCK_DGRAM, 0);
ctx.addr.sin_family = AF_INET;
ctx.addr.sin_addr.s_addr = inet_addr(argv[1]);
ctx.addr.sin_port = htons(atoi(argv[2]));
bind(ctx.sockfd, (struct sockaddr *)&ctx.addr, sizeof(ctx.addr));
// 创建AVPacket
ctx.pkt = av_packet_alloc();
// 启动接收线程
pthread_t recv_thread;
pthread_create(&recv_thread, NULL, recv_rtp_packets, &ctx);
// 等待接收线程结束
pthread_join(recv_thread, NULL);
// 清理资源
av_packet_free(&ctx.pkt);
avcodec_free_context(&ctx.codec_ctx);
avformat_close_input(&ctx.fmt_ctx);
avformat_network_deinit();
return 0;
}
```
代码中使用了FFmpeg的C API来实现RTP流的接收和音频解码,需要注意的是,音频帧的处理需要在解码器解码后进行。在代码中使用了一个线程来接收RTP数据包,当收到数据包后,将其存入一个AVPacket中,然后送给解码器进行解码。解码器每次解码出一帧音频数据后,将其存储在AVCodecParameters结构体中,可以通过该结构体的成员变量获取音频数据的信息。可以根据需要修改音频流的参数和输出方式。
ffmpeg rtp接收pcm音频流代码
以下是使用FFmpeg接收RTP传输的PCM音频流的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/avutil.h>
#include <libavutil/opt.h>
#include <libavutil/samplefmt.h>
#include <libswresample/swresample.h>
#define AUDIO_BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Usage: %s [RTP URL]\n", argv[0]);
return 1;
}
av_register_all();
avcodec_register_all();
AVFormatContext *formatCtx = NULL;
AVCodecContext *codecCtx = NULL;
AVCodec *codec = NULL;
AVPacket packet;
AVFrame *frame = NULL;
int streamIndex = -1;
uint8_t inBuffer[AUDIO_BUFFER_SIZE + AV_INPUT_BUFFER_PADDING_SIZE];
int inBufferLen;
uint8_t *data = NULL;
int dataSize = 0;
int ret;
if ((ret = avformat_open_input(&formatCtx, argv[1], NULL, NULL)) < 0) {
printf("Failed to open input: %s\n", av_err2str(ret));
return 1;
}
if ((ret = avformat_find_stream_info(formatCtx, NULL)) < 0) {
printf("Failed to find stream info: %s\n", av_err2str(ret));
return 1;
}
for (int i = 0; i < formatCtx->nb_streams; i++) {
if (formatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_AUDIO) {
streamIndex = i;
break;
}
}
if (streamIndex == -1) {
printf("Failed to find audio stream\n");
return 1;
}
codec = avcodec_find_decoder(formatCtx->streams[streamIndex]->codecpar->codec_id);
if (codec == NULL) {
printf("Failed to find codec\n");
return 1;
}
codecCtx = avcodec_alloc_context3(codec);
if (codecCtx == NULL) {
printf("Failed to allocate codec context\n");
return 1;
}
if ((ret = avcodec_parameters_to_context(codecCtx, formatCtx->streams[streamIndex]->codecpar)) < 0) {
printf("Failed to copy codec parameters to context: %s\n", av_err2str(ret));
return 1;
}
if ((ret = avcodec_open2(codecCtx, codec, NULL)) < 0) {
printf("Failed to open codec: %s\n", av_err2str(ret));
return 1;
}
frame = av_frame_alloc();
if (frame == NULL) {
printf("Failed to allocate frame\n");
return 1;
}
av_init_packet(&packet);
AVIOContext *avioCtx = NULL;
struct sockaddr_in servAddr;
int sock = socket(AF_INET, SOCK_DGRAM, 0);
if (sock < 0) {
printf("Failed to create socket\n");
return 1;
}
memset(&servAddr, 0, sizeof(servAddr));
servAddr.sin_family = AF_INET;
servAddr.sin_addr.s_addr = INADDR_ANY;
servAddr.sin_port = htons(1234); // replace with your RTP port
if (bind(sock, (struct sockaddr *)&servAddr, sizeof(servAddr)) < 0) {
printf("Failed to bind socket\n");
return 1;
}
avioCtx = avio_alloc_context(inBuffer, sizeof(inBuffer), 0, sock, NULL, NULL, NULL);
avioCtx->seekable = 0;
formatCtx->pb = avioCtx;
while (av_read_frame(formatCtx, &packet) >= 0) {
if (packet.stream_index == streamIndex) {
ret = avcodec_send_packet(codecCtx, &packet);
if (ret < 0) {
printf("Failed to send packet to codec: %s\n", av_err2str(ret));
break;
}
while (ret >= 0) {
ret = avcodec_receive_frame(codecCtx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
printf("Failed to receive frame from codec: %s\n", av_err2str(ret));
goto end;
}
dataSize = av_samples_get_buffer_size(NULL, codecCtx->channels, frame->nb_samples, codecCtx->sample_fmt, 1);
data = frame->data[0];
// TODO: Process audio data here
av_frame_unref(frame);
}
}
av_packet_unref(&packet);
}
end:
avcodec_free_context(&codecCtx);
avformat_close_input(&formatCtx);
av_frame_free(&frame);
avio_context_free(&avioCtx);
close(sock);
return 0;
}
```
这是一个简单的示例,它假定您已经了解如何使用FFmpeg解码音频流并处理音频数据。您需要将代码中的RTP端口号替换为您要使用的端口号,并且需要编写自己的音频处理代码,以便将音频数据保存到文件或以其他方式处理它。
相关推荐
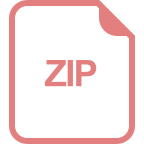
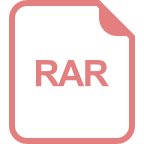
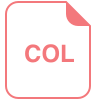











