1、利用栈操作,解决表达式求值问题的c++运算代码。 2、利用队列操作,解决舞伴配对问题的c++运算代码。
时间: 2023-06-02 15:06:37 浏览: 111
抱歉,我可以回答您的问题。
以下是利用栈操作,解决表达式求值问题的C运算代码:
#include<stdio.h>
#include<stdlib.h>
#include<ctype.h>
#define MAXSIZE 100
typedef struct stack{
int top;
int data[MAXSIZE];
}Stack;
void initStack(Stack *s){
s->top = -1;
}
int isStackEmpty(Stack *s){
return s->top == -1;
}
int isStackFull(Stack *s){
return s->top == (MAXSIZE - 1);
}
int push(Stack *s, int value){
if(isStackFull(s))
return 0;
else{
s->top++;
s->data[s->top] = value;
return 1;
}
}
int pop(Stack *s, int *value){
if(isStackEmpty(s))
return 0;
else{
*value = s->data[s->top];
s->top--;
return 1;
}
}
int main(){
Stack s;
initStack(&s);
char c;
int num, a, b;
while((c = getchar()) != '\n'){
if(isdigit(c)){
num = c - '0';
while(isdigit(c = getchar())){
num = num * 10 + c - '0';
}
push(&s, num);
}
if(c == '+' || c == '-' || c == '*' || c == '/'){
pop(&s, &a);
pop(&s, &b);
switch(c){
case '+':
push(&s, a + b);
break;
case '-':
push(&s, b - a);
break;
case '*':
push(&s, a * b);
break;
case '/':
push(&s, b / a);
break;
}
}
}
printf("%d\n", s.data[s.top]);
return 0;
}
以下是利用队列操作,解决舞伴配对问题的C运算代码:
#include<stdio.h>
#include<stdlib.h>
#define MAXSIZE 100
typedef struct queue{
int front;
int rear;
int data[MAXSIZE];
}Queue;
void initQueue(Queue *q){
q->front = q->rear = 0;
}
int isQueueEmpty(Queue *q){
return q->front == q->rear;
}
int isQueueFull(Queue *q){
return (q->rear + 1) % MAXSIZE == q->front;
}
int enqueue(Queue *q, int value){
if(isQueueFull(q))
return 0;
else{
q->data[q->rear] = value;
q->rear = (q->rear + 1) % MAXSIZE;
return 1;
}
}
int dequeue(Queue *q, int *value){
if(isQueueEmpty(q))
return 0;
else{
*value = q->data[q->front];
q->front = (q->front + 1) % MAXSIZE;
return 1;
}
}
void dancePartners(Queue *men, Queue *women){
int man, woman;
while(!isQueueEmpty(men) && !isQueueEmpty(women)){
dequeue(men, &man);
dequeue(women, &woman);
printf("%d is dancing with %d\n", man, woman);
}
if(!isQueueEmpty(men))
printf("Unmatched men: ");
while(!isQueueEmpty(men)){
dequeue(men, &man);
printf("%d ", man);
}
printf("\n");
if(!isQueueEmpty(women))
printf("Unmatched women: ");
while(!isQueueEmpty(women)){
dequeue(women, &woman);
printf("%d ", woman);
}
printf("\n");
}
int main(){
Queue men, women;
initQueue(&men);
initQueue(&women);
enqueue(&men, 1);
enqueue(&men, 4);
enqueue(&men, 7);
enqueue(&women, 3);
enqueue(&women, 6);
enqueue(&women, 9);
dancePartners(&men, &women);
return 0;
}
希望能帮助您!
阅读全文
相关推荐





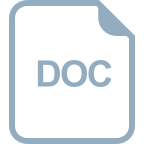


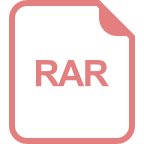

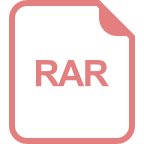
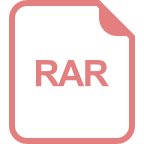

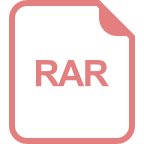