如何用C语言统计数组中出现次数最多的数字,需要使用二分法
时间: 2023-05-29 11:04:14 浏览: 373
使用二分法统计数组中出现次数最多的数字可以按照以下步骤:
1. 对数组进行排序,以便使用二分法查找数字。
2. 遍历数组,用一个计数器记录每个数字出现的次数。
3. 用二分法查找数组中出现次数最多的数字。
4. 返回出现次数最多的数字。
下面是具体的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
int binary_search(int arr[], int n, int x) {
int left = 0, right = n - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == x) {
return mid;
} else if (arr[mid] < x) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
int main() {
int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 9, 9, 9};
int n = sizeof(arr) / sizeof(arr[0]);
int count[100] = {0}; // 数字最大值为100
int max_count = 0, max_num;
// 统计每个数字的出现次数
for (int i = 0; i < n; i++) {
count[arr[i]]++;
if (count[arr[i]] > max_count) {
max_count = count[arr[i]];
max_num = arr[i];
}
}
// 用二分法查找出现次数最多的数字
int idx = binary_search(arr, n, max_num);
printf("数字 %d 出现次数最多,共出现 %d 次,第一次出现的位置为 %d\n", max_num, max_count, idx);
return 0;
}
```
在上面的代码中,我们首先创建了一个计数器数组 `count`,用来记录每个数字出现的次数。然后遍历原数组 `arr`,将每个数字的出现次数加一,并同时记录出现次数最多的数字和它的出现次数。最后,我们使用二分法查找出现次数最多的数字在原数组中的位置,并输出相关信息。
相关推荐
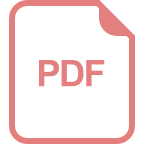
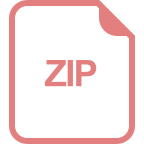














