Java实现des加密 解密要求有界面
时间: 2023-05-26 07:04:05 浏览: 168
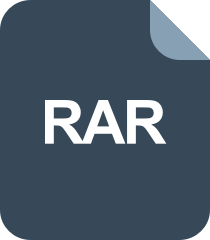
des.rar_DES加密 有界面_Des加密解密_des_加密 解密_加密解密
下面是一个简单的Java程序,可以实现DES加密和解密,并带有基本的界面。
该程序使用Java Swing库来创建用户界面,使用Java Cryptography Extension (JCE)库来实现DES加密和解密。
界面包括两个文本框和四个按钮。文本框用于输入要加密或解密的文本,按钮用于执行加密和解密操作。
程序代码如下:
```
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.security.Key;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextArea;
public class DesEncryptDecrypt extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private static final String ALGORITHM = "DES";
private static final String TRANSFORMATION = "DES/ECB/PKCS5Padding";
private static final byte[] KEY = "mykey123".getBytes();
private JTextArea inputTextArea, outputTextArea;
public DesEncryptDecrypt() {
super("DES Encrypt/Decrypt");
JPanel inputPanel = new JPanel(new BorderLayout());
inputPanel.add(new JTextArea("Input"), BorderLayout.NORTH);
inputTextArea = new JTextArea(5, 20);
inputPanel.add(inputTextArea, BorderLayout.CENTER);
JPanel outputPanel = new JPanel(new BorderLayout());
outputPanel.add(new JTextArea("Output"), BorderLayout.NORTH);
outputTextArea = new JTextArea(5, 20);
outputPanel.add(outputTextArea, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel();
JButton encryptButton = new JButton("Encrypt");
encryptButton.addActionListener(this);
buttonPanel.add(encryptButton);
JButton decryptButton = new JButton("Decrypt");
decryptButton.addActionListener(this);
buttonPanel.add(decryptButton);
add(inputPanel, BorderLayout.NORTH);
add(outputPanel, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setLocationRelativeTo(null);
setVisible(true);
}
public static void main(String[] args) {
new DesEncryptDecrypt();
}
@Override
public void actionPerformed(ActionEvent e) {
try {
Key key = new SecretKeySpec(KEY, ALGORITHM);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
switch (e.getActionCommand()) {
case "Encrypt":
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encryptedBytes = cipher.doFinal(inputTextArea.getText().getBytes());
outputTextArea.setText(new String(encryptedBytes));
break;
case "Decrypt":
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] decryptedBytes = cipher.doFinal(inputTextArea.getText().getBytes());
outputTextArea.setText(new String(decryptedBytes));
break;
}
} catch (Exception ex) {
JOptionPane.showMessageDialog(this, "Error: " + ex.getMessage());
}
}
}
```
该程序使用了一个固定的密钥作为加密解密算法的秘钥,为了更安全,使用者应该使用至少128位的随机密钥,并将该密钥储存在安全的地方。
阅读全文
相关推荐
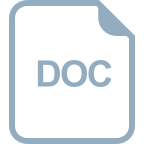
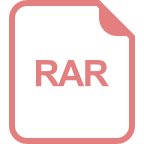
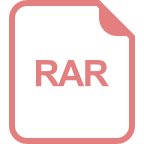
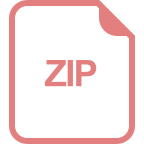
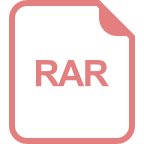
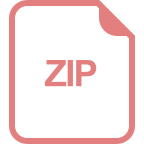
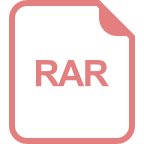
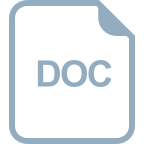
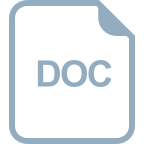
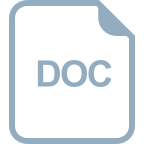
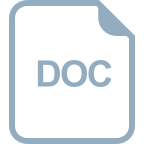
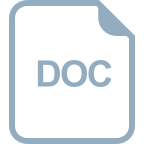
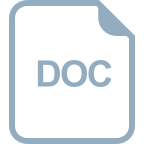