使用for循环尾插法建立一个单链表
时间: 2023-09-18 07:08:39 浏览: 165
好的,以下是使用for循环尾插法建立一个单链表的示例代码:
```C++
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 尾插法建立单链表,返回链表头节点指针
ListNode* createList(int n) {
// 定义头节点,其val值为0,next指向NULL
ListNode* head = new ListNode(0);
// 定义尾节点指针tail,初始值指向头节点
ListNode* tail = head;
// 依次插入n个节点
for (int i = 1; i <= n; i++) {
// 读入节点值
int x;
cin >> x;
// 创建新节点
ListNode* newNode = new ListNode(x);
// 将新节点插入到尾部
tail->next = newNode;
// 更新尾节点指针
tail = newNode;
}
// 返回链表头节点指针
return head->next;
}
// 打印链表
void printList(ListNode* head) {
ListNode* p = head;
while (p != NULL) {
cout << p->val << " ";
p = p->next;
}
cout << endl;
}
int main() {
// 读入链表长度
int n;
cin >> n;
// 建立链表
ListNode* head = createList(n);
// 打印链表
printList(head);
return 0;
}
```
以上代码中,我们通过定义链表节点结构体 `ListNode` 和使用for循环尾插法来建立单链表。同时,我们也提供了打印链表的函数 `printList`。
阅读全文
相关推荐
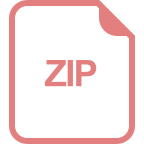
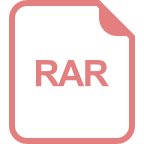
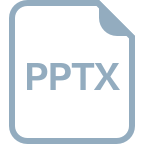















