将datagridview里的一条数据显示在另一个窗体里的多个textbox里,修改多个textbox内容更新到数据库里
时间: 2024-03-02 11:51:41 浏览: 31
好的,针对您的问题,我可以给出以下解决方案:
1. 在主窗体中的datagridview的CellDoubleClick事件中,获取当前选中的行的数据,并将数据传递给另一个窗体。
2. 在另一个窗体中,将传递过来的数据显示在对应的textbox中。
3. 在textbox的TextChanged事件中,将修改后的数据保存到内存中。
4. 在保存按钮的Click事件中,将修改后的数据更新到数据库中。
具体实现过程如下:
1. 在主窗体中的datagridview的CellDoubleClick事件中,获取当前选中的行的数据,并将数据传递给另一个窗体。
```csharp
private void dataGridView1_CellDoubleClick(object sender, DataGridViewCellEventArgs e)
{
if (e.RowIndex >= 0)
{
DataGridViewRow row = dataGridView1.Rows[e.RowIndex];
// 创建一个新的窗体,并传递数据
DetailForm detailForm = new DetailForm();
detailForm.SetData(row.Cells["Name"].Value.ToString(),
row.Cells["Age"].Value.ToString(),
row.Cells["Address"].Value.ToString());
detailForm.ShowDialog();
}
}
```
2. 在另一个窗体中,将传递过来的数据显示在对应的textbox中。
```csharp
public partial class DetailForm : Form
{
// 保存传递过来的数据
private string name;
private string age;
private string address;
public DetailForm()
{
InitializeComponent();
}
// 设置数据的方法
public void SetData(string name, string age, string address)
{
this.name = name;
this.age = age;
this.address = address;
}
private void DetailForm_Load(object sender, EventArgs e)
{
txtName.Text = name;
txtAge.Text = age;
txtAddress.Text = address;
}
}
```
3. 在textbox的TextChanged事件中,将修改后的数据保存到内存中。
```csharp
private void txtName_TextChanged(object sender, EventArgs e)
{
this.name = txtName.Text;
}
private void txtAge_TextChanged(object sender, EventArgs e)
{
this.age = txtAge.Text;
}
private void txtAddress_TextChanged(object sender, EventArgs e)
{
this.address = txtAddress.Text;
}
```
4. 在保存按钮的Click事件中,将修改后的数据更新到数据库中。
```csharp
private void btnSave_Click(object sender, EventArgs e)
{
// 更新到数据库中
UpdateDataToDB(name, age, address);
MessageBox.Show("数据保存成功!");
}
```
以上就是将datagridview里的一条数据显示在另一个窗体里的多个textbox里,修改多个textbox内容更新到数据库里的解决方案。希望能对您有所帮助!
相关推荐
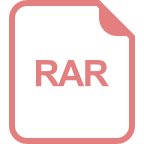
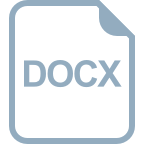
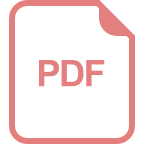














