C语言 实现视角的移动
时间: 2023-05-11 17:04:43 浏览: 101
可以使用OpenGL库来实现视角的移动,具体实现方法可以参考以下代码:
#include <GL/glut.h>
// 定义视角的位置和方向
GLfloat position[] = {0.0, 0.0, 5.0, 1.0};
GLfloat direction[] = {0.0, 0.0, -1.0, 0.0};
void display() {
// 清空屏幕
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// 设置视角
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
gluLookAt(position[0], position[1], position[2],
position[0] + direction[0], position[1] + direction[1], position[2] + direction[2],
0.0, 1.0, 0.0);
// 绘制场景
// ...
// 刷新屏幕
glutSwapBuffers();
}
void keyboard(unsigned char key, int x, int y) {
switch (key) {
case 'w':
// 向前移动
position[0] += direction[0] * 0.1;
position[1] += direction[1] * 0.1;
position[2] += direction[2] * 0.1;
break;
case 's':
// 向后移动
position[0] -= direction[0] * 0.1;
position[1] -= direction[1] * 0.1;
position[2] -= direction[2] * 0.1;
break;
case 'a':
// 向左移动
position[0] += direction[2] * 0.1;
position[2] -= direction[0] * 0.1;
break;
case 'd':
// 向右移动
position[0] -= direction[2] * 0.1;
position[2] += direction[0] * 0.1;
break;
}
glutPostRedisplay();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(640, 480);
glutCreateWindow("视角移动");
glutDisplayFunc(display);
glutKeyboardFunc(keyboard);
glEnable(GL_DEPTH_TEST);
glutMainLoop();
return 0;
}
这段代码使用了OpenGL库中的gluLookAt函数来设置视角的位置和方向,然后通过键盘事件来移动视角的位置。
阅读全文
相关推荐
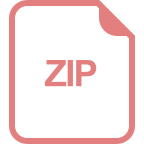
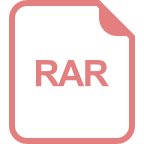
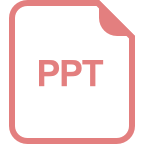










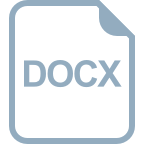
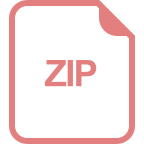
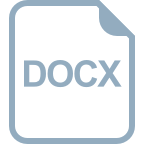
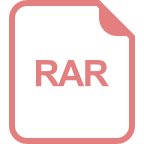
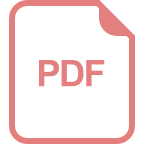
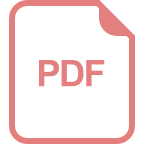