写一个python程序。利用pycrfsuite库。训练crf模型,给一个中文的bio序列贴上另一个bio序列的标签,然后利用此训练好的模型,给新的bio序列贴上新的bio标签
时间: 2024-02-01 22:13:06 浏览: 69
以下是一个简单的示例程序,用于训练和测试CRF模型。在这里,我们使用了pycrfsuite库和中文的分词数据集MSRA。
```python
import pycrfsuite
import codecs
# 加载数据
def load_data(filename):
sents = []
with codecs.open(filename, 'r', encoding='utf8') as f:
sent = []
for line in f:
line = line.strip()
if len(line) == 0:
if len(sent) > 0:
sents.append(sent)
sent = []
else:
word, label = line.split()
sent.append((word, label))
if len(sent) > 0:
sents.append(sent)
return sents
# 特征提取函数
def word2features(sent, i):
word = sent[i][0]
features = [
'bias',
'word.lower=' + word.lower(),
'word[-3:]=' + word[-3:],
'word[-2:]=' + word[-2:],
'word.isnumeric=%s' % word.isnumeric(),
'word.isdigit=%s' % word.isdigit(),
]
if i > 0:
word1 = sent[i-1][0]
features.extend([
'-1:word.lower=' + word1.lower(),
'-1:word[-3:]=' + word1[-3:],
'-1:word[-2:]=' + word1[-2:],
'-1:word.isnumeric=%s' % word1.isnumeric(),
'-1:word.isdigit=%s' % word1.isdigit(),
])
else:
features.append('BOS')
if i < len(sent)-1:
word1 = sent[i+1][0]
features.extend([
'+1:word.lower=' + word1.lower(),
'+1:word[-3:]=' + word1[-3:],
'+1:word[-2:]=' + word1[-2:],
'+1:word.isnumeric=%s' % word1.isnumeric(),
'+1:word.isdigit=%s' % word1.isdigit(),
])
else:
features.append('EOS')
return features
# 特征提取函数
def sent2features(sent):
return [word2features(sent, i) for i in range(len(sent))]
# 标签提取函数
def sent2labels(sent):
return [label for _, label in sent]
# 序列提取函数
def sent2seq(sent):
return [word for word, _ in sent]
# 训练模型
def train_model(train_file, model_file):
# 加载训练数据
train_sents = load_data(train_file)
# 创建Trainer
trainer = pycrfsuite.Trainer(verbose=False)
# 加载训练数据
for sent in train_sents:
features = sent2features(sent)
labels = sent2labels(sent)
trainer.append(features, labels)
# 设置参数
trainer.set_params({
'c1': 1.0, # L1正则化系数
'c2': 1e-3, # L2正则化系数
'max_iterations': 100, # 最大迭代次数
'feature.possible_transitions': True # 允许所有转移
})
# 训练模型
trainer.train(model_file)
# 测试模型
def test_model(model_file, test_file, result_file):
# 加载测试数据
test_sents = load_data(test_file)
# 创建Tagger
tagger = pycrfsuite.Tagger()
tagger.open(model_file)
# 预测标签
with codecs.open(result_file, 'w', encoding='utf8') as f:
for sent in test_sents:
features = sent2features(sent)
labels = tagger.tag(features)
words = sent2seq(sent)
for word, label in zip(words, labels):
f.write(word + ' ' + label + '\n')
f.write('\n')
# 训练模型
train_file = 'msr_training_bio.txt'
model_file = 'crf_model.bin'
train_model(train_file, model_file)
# 测试模型
test_file = 'msr_test_bio.txt'
result_file = 'result.txt'
test_model(model_file, test_file, result_file)
```
在这个例子中,我们使用了MSRA数据集,其中包含了中文的分词数据。我们首先通过load_data函数将数据加载到内存中,并使用sent2features、sent2labels和sent2seq函数将数据转换为特征、标签和序列。然后,我们使用Trainer类来训练CRF模型,并使用Tagger类来预测新的序列标签。最后,我们将预测结果写入文件中。
注意,这只是一个简单的示例程序,实际中还需要进行更多的特征工程和模型调参。
阅读全文
相关推荐
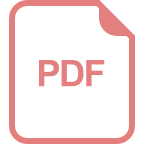


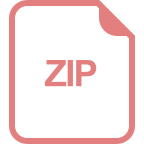
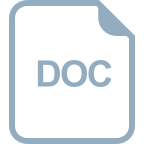
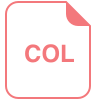
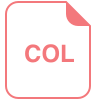
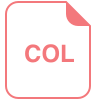
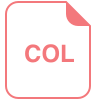
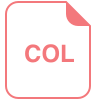
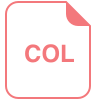


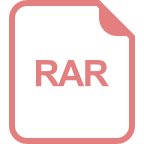