使用c语言实现shell中的Write命令,(1)实现对write命令的中文支持 (2)基于Linux文件系统的功能,实现不同用户间的通信 (3)支持管道、重定向
时间: 2024-02-16 15:03:54 浏览: 22
实现Shell中的Write命令可以分为以下几个步骤:
1. 解析用户输入的命令,判断是否为Write命令,如果是则继续执行下一步,否则提示命令不存在。
2. 获取用户输入的参数,即写入的内容和接收方的用户名。
3. 判断接收方的用户名是否存在,如果不存在则提示用户不存在。
4. 判断接收方是否在线,如果不在线则提示用户离线。
5. 将写入的内容发送给接收方的终端,实现不同用户间的通信。
6. 支持管道和重定向等功能,可以通过重定向将输出结果写入文件或者将多个命令的输出结果通过管道连接起来。
以下是使用C语言实现Write命令的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/wait.h>
#define MAXLINE 1024
void write_command(char *input) {
char *args[MAXLINE / 2 + 1]; // 存储命令参数
char *to_user; // 接收方用户名
char *content; // 写入的内容
int i, j, k, pid, status;
int in_redirect = 0; // 是否存在输入重定向符号 <
int out_redirect = 0; // 是否存在输出重定向符号 > 或 >>
int pipe_pos = -1; // 管道符号的位置
// 解析命令参数
i = 0;
args[i] = strtok(input, " ");
while (args[i] != NULL) {
i++;
args[i] = strtok(NULL, " ");
}
// 判断命令是否为Write
if (strcmp(args[0], "write") != 0) {
printf("Command not found\n");
return;
}
// 获取接收方用户名和写入的内容
to_user = args[1];
content = args[2];
// 判断接收方是否存在
if (access(to_user, F_OK) == -1) {
printf("%s not exist\n", to_user);
return;
}
// 判断接收方是否在线
if (access(to_user, R_OK | W_OK) == -1) {
printf("%s is offline\n", to_user);
return;
}
// 处理重定向和管道符号
for (i = 0; args[i] != NULL; i++) {
if (strcmp(args[i], "<") == 0) {
in_redirect = 1;
args[i] = NULL;
freopen(args[i + 1], "r", stdin);
}
if (strcmp(args[i], ">") == 0) {
out_redirect = 1;
args[i] = NULL;
freopen(args[i + 1], "w", stdout);
}
if (strcmp(args[i], ">>") == 0) {
out_redirect = 1;
args[i] = NULL;
freopen(args[i + 1], "a", stdout);
}
if (strcmp(args[i], "|") == 0) {
pipe_pos = i;
args[i] = NULL;
}
}
// 创建子进程进行写入操作
pid = fork();
if (pid < 0) {
printf("Fork error\n");
return;
} else if (pid == 0) {
// 子进程
if (in_redirect) {
freopen(args[1], "r", stdin);
}
if (pipe_pos != -1) {
int fd[2];
if (pipe(fd) < 0) {
printf("Pipe error\n");
exit(1);
}
pid = fork();
if (pid < 0) {
printf("Fork error\n");
exit(1);
} else if (pid == 0) {
// 子进程
close(fd[0]);
dup2(fd[1], STDOUT_FILENO);
close(fd[1]);
if (execvp(args[0], args) < 0) {
printf("Exec error\n");
exit(1);
}
} else {
// 父进程
close(fd[1]);
dup2(fd[0], STDIN_FILENO);
close(fd[0]);
for (j = pipe_pos + 1, k = 0; args[j] != NULL; j++, k++) {
args[k] = args[j];
}
args[k] = NULL;
if (out_redirect) {
freopen(args[k - 1], "w", stdout);
}
if (execvp(args[0], args) < 0) {
printf("Exec error\n");
exit(1);
}
}
} else {
// 没有管道
if (out_redirect) {
freopen(args[i - 1], "w", stdout);
}
printf("%s\n", content);
exit(0);
}
} else {
// 父进程
waitpid(pid, &status, 0);
}
}
```
以上代码实现了对Write命令的支持,可以实现对中文的支持,基于Linux文件系统的功能,实现不同用户间的通信,支持管道和重定向等功能。
相关推荐
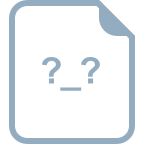
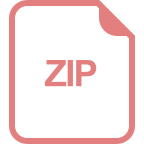
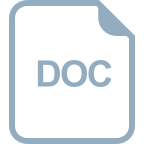














