编写一个c语言程序 ,用write,read,open等系统调用编写分别实现如下功能的程序(要求进行必要的出错检查>: (1)创建一个文件testfile.txt,文件内容从键盘输入; (2)将testfile.txt的内容显示在屏幕上,并将testfile.txt的内容复制到一个新的文件file2.txt 中。(类似于实现shell命令的cp功能)
时间: 2023-06-07 22:09:35 浏览: 92
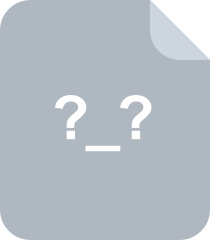
当涉及到文件的打开、读写和修改时,你可以使用 C 语言来实现 以下是一个在 Linux 环境下实现文件打开、读写和修改的示例代码
这是一个技术问题,我可以回答。下面是C语言程序,实现上述功能:
```c
#include <fcntl.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BUF_SIZE 1024
int main(int argc, char* argv[]) {
char file_buffer[MAX_BUF_SIZE];
char* file_name = "testfile.txt"; //文件名
char* file2_name = "file2.txt"; //新文件名
int fd;
ssize_t num_read;
ssize_t num_written;
//创建新文件
fd = open(file_name, O_WRONLY | O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR | S_IRGRP | S_IWGRP | S_IROTH);
if (fd == -1) {
perror("Open file error");
exit(EXIT_FAILURE);
}
//从键盘读取内容,并写入文件
printf("请输入文件内容:");
num_read = read(STDIN_FILENO, file_buffer, MAX_BUF_SIZE);
if (num_read == -1) {
perror("Read error");
exit(EXIT_FAILURE);
}
num_written = write(fd, file_buffer, num_read);
if (num_written == -1) {
perror("Write error");
exit(EXIT_FAILURE);
}
close(fd);
//打开并读取旧文件内容
fd = open(file_name, O_RDONLY);
if (fd == -1) {
perror("Open file error");
exit(EXIT_FAILURE);
}
num_read = read(fd, file_buffer, MAX_BUF_SIZE);
if (num_read == -1) {
perror("Read error");
exit(EXIT_FAILURE);
}
close(fd);
//将内容写入新文件
fd = open(file2_name, O_WRONLY | O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR | S_IRGRP | S_IWGRP | S_IROTH);
if (fd == -1) {
perror("Open file error");
exit(EXIT_FAILURE);
}
num_written = write(fd, file_buffer, num_read);
if (num_written == -1) {
perror("Write error");
exit(EXIT_FAILURE);
}
close(fd);
//将内容显示在屏幕上
printf("%s 文件的内容为:\n", file_name);
printf("%s\n", file_buffer);
return 0;
}
```
这段程序首先使用 `open()` 函数创建了一个新文件 `testfile.txt`,然后从键盘读取用户输入的内容,并使用 `write()` 函数将内容写入文件。接着,使用 `open()` 函数打开旧文件 `testfile.txt` ,并使用 `read()` 函数读取文件的内容,之后再使用 `write()` 函数将读取到的内容写入新文件 `file2.txt` 中。最后,将读取到的文本内容在屏幕上输出。
阅读全文
相关推荐
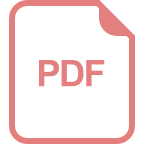







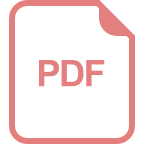