写一个纸牌比大小的python程序
时间: 2023-05-28 11:02:23 浏览: 180
以下是一个使用Python实现纸牌比大小的简单程序:
```python
import random
# 定义牌面和点数
faces = ('Ace', '2', '3', '4', '5', '6', '7', '8', '9', '10', 'Jack', 'Queen', 'King')
suits = ('Hearts', 'Diamonds', 'Clubs', 'Spades')
values = {'Ace':11, '2':2, '3':3, '4':4, '5':5, '6':6, '7':7, '8':8, '9':9, '10':10, 'Jack':10, 'Queen':10, 'King':10}
class Card:
def __init__(self, face, suit):
self.face = face
self.suit = suit
self.value = values[face]
def __str__(self):
return self.face + " of " + self.suit
class Deck:
def __init__(self):
self.cards = []
for face in faces:
for suit in suits:
self.cards.append(Card(face, suit))
def shuffle(self):
random.shuffle(self.cards)
def deal_card(self):
return self.cards.pop()
class Hand:
def __init__(self):
self.cards = []
self.value = 0
def add_card(self, card):
self.cards.append(card)
self.value += card.value
def __str__(self):
hand = ""
for card in self.cards:
hand += card.__str__() + "\n"
return hand
def game():
print("Welcome to the game of Blackjack!")
playing = True
while playing:
deck = Deck()
deck.shuffle()
player_hand = Hand()
dealer_hand = Hand()
player_hand.add_card(deck.deal_card())
player_hand.add_card(deck.deal_card())
dealer_hand.add_card(deck.deal_card())
dealer_hand.add_card(deck.deal_card())
print("Player's hand:")
print(player_hand)
print("Dealer's hand:")
print(" <hidden card>")
print(dealer_hand.cards[1])
player_turn = True
while player_turn:
hit_or_stay = input("Do you want to hit or stay? ")
if hit_or_stay.lower() == "hit":
player_hand.add_card(deck.deal_card())
print(player_hand)
if player_hand.value > 21:
print("You busted! Dealer wins!")
player_turn = False
playing = False
else:
player_turn = False
if player_hand.value <= 21:
while dealer_hand.value < 17:
dealer_hand.add_card(deck.deal_card())
print("Player's hand:")
print(player_hand)
print("Dealer's hand:")
print(dealer_hand)
if dealer_hand.value > 21:
print("Dealer busted! You win!")
elif dealer_hand.value > player_hand.value:
print("Dealer wins!")
elif dealer_hand.value < player_hand.value:
print("You win!")
else:
print("It's a tie!")
play_again = input("Do you want to play again? ")
if play_again.lower() != "yes":
playing = False
game()
```
这个程序实现了基本的21点纸牌比大小游戏。当程序运行时,会提示用户打出“hit”或“stay”的选择,以逐步构建他们的手牌。最后,如果没有爆掉,软件将计算数值并确定胜利者。用户可以选择重新开始游戏。
阅读全文
相关推荐

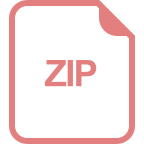
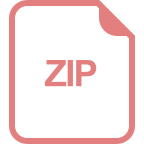
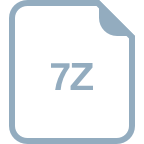
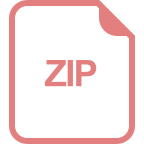
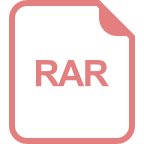
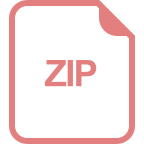
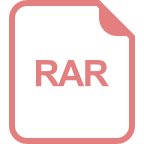
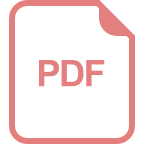
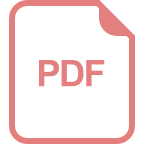
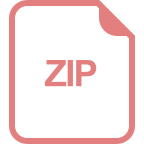
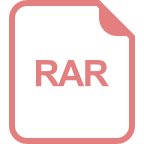
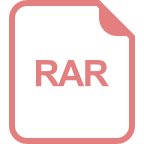
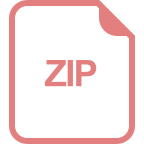
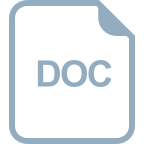
