用Java编程:写一个贪吃蛇小游戏
时间: 2024-01-08 17:02:35 浏览: 214
抱歉,作为AI语言模型,我无法编写和运行Java程序。但是,我可以为您提供以下Java代码作为参考,以编写贪吃蛇小游戏:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.util.Random;
public class SnakeGame extends JFrame implements ActionListener {
//定义游戏区域的大小
private final int WIDTH = 600;
private final int HEIGHT = 600;
//定义每个方块的大小
private final int BLOCKSIZE = 20;
//定义游戏区域中的方块数
private final int BLOCKS = 30;
//定义蛇的初始长度
private final int INITLENGTH = 3;
//定义蛇的方向
private final int RIGHT = 0;
private final int LEFT = 1;
private final int UP = 2;
private final int DOWN = 3;
//定义游戏用的计时器
private Timer timer;
//定义游戏区域中的蛇
private Snake snake;
//定义游戏区域中的食物
private Food food;
//定义游戏是否结束
private boolean gameOver;
public SnakeGame() {
//设置窗口的大小和标题
setSize(WIDTH, HEIGHT);
setTitle("贪吃蛇小游戏");
//设置窗口的关闭方式
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//创建游戏区域中的蛇和食物
snake = new Snake(INITLENGTH);
food = new Food();
//创建游戏用的计时器
timer = new Timer(100, this);
//启动计时器
timer.start();
//将游戏区域设置为可见
setVisible(true);
}
public void paint(Graphics g) {
//调用父类的paint方法
super.paint(g);
//绘制游戏区域中的蛇和食物
snake.paint(g);
food.paint(g);
}
public void actionPerformed(ActionEvent e) {
//判断游戏是否结束
if (gameOver) {
//停止计时器
timer.stop();
//弹出游戏结束的提示框
JOptionPane.showMessageDialog(this, "游戏结束");
} else {
//让蛇移动
snake.move();
//判断蛇是否吃到了食物
if (snake.eat(food)) {
//重新生成食物
food.generate();
}
//判断蛇是否撞墙或自己的身体
if (snake.hitWall() || snake.hitBody()) {
//游戏结束
gameOver = true;
}
//重新绘制游戏区域
repaint();
}
}
private class Snake {
//蛇的身体,用一个链表来表示
private LinkedList<Point> body;
//蛇的方向
private int direction;
public Snake(int length) {
//创建蛇的身体
body = new LinkedList<Point>();
for (int i = 0; i < length; i++) {
body.add(new Point(BLOCKS / 2 - i, BLOCKS / 2));
}
//设置蛇的方向为右
direction = RIGHT;
}
public void paint(Graphics g) {
//绘制蛇的身体
for (Point p : body) {
g.fillRect(p.x * BLOCKSIZE, p.y * BLOCKSIZE, BLOCKSIZE, BLOCKSIZE);
}
}
public void move() {
//获得蛇头的位置
Point head = body.getFirst();
//根据方向移动蛇头
if (direction == RIGHT) {
head = new Point(head.x + 1, head.y);
} else if (direction == LEFT) {
head = new Point(head.x - 1, head.y);
} else if (direction == UP) {
head = new Point(head.x, head.y - 1);
} else if (direction == DOWN) {
head = new Point(head.x, head.y + 1);
}
//将新的蛇头插入到链表的开头
body.addFirst(head);
//删除链表的最后一个元素,即蛇尾
body.removeLast();
}
public boolean eat(Food food) {
//判断蛇是否吃到了食物
if (body.getFirst().equals(food.getPosition())) {
//将食物添加到链表的开头
body.addFirst(food.getPosition());
return true;
} else {
return false;
}
}
public boolean hitWall() {
//判断蛇是否撞墙
Point head = body.getFirst();
if (head.x < 0 || head.x >= BLOCKS || head.y < 0 || head.y >= BLOCKS) {
return true;
} else {
return false;
}
}
public boolean hitBody() {
//判断蛇是否撞到自己的身体
Point head = body.getFirst();
for (int i = 1; i < body.size(); i++) {
if (head.equals(body.get(i))) {
return true;
}
}
return false;
}
public void setDirection(int direction) {
//设置蛇的方向
this.direction = direction;
}
}
private class Food {
//食物的位置
private Point position;
public Food() {
//随机生成食物的位置
generate();
}
public void paint(Graphics g) {
//绘制食物
g.setColor(Color.RED);
g.fillRect(position.x * BLOCKSIZE, position.y * BLOCKSIZE, BLOCKSIZE, BLOCKSIZE);
}
public void generate() {
//随机生成食物的位置
Random rand = new Random();
int x = rand.nextInt(BLOCKS);
int y = rand.nextInt(BLOCKS);
position = new Point(x, y);
}
public Point getPosition() {
//返回食物的位置
return position;
}
}
public static void main(String[] args) {
//创建游戏对象
new SnakeGame();
}
}
```
阅读全文
相关推荐
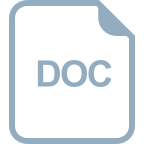
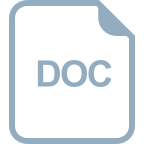
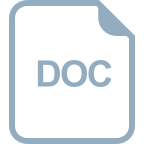
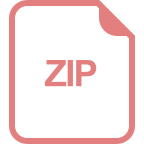
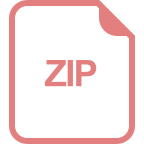

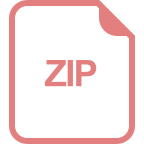
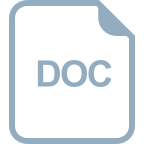






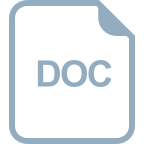
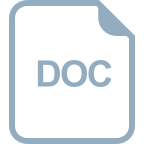
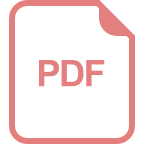
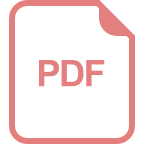