写一篇2000字Python语言语法的文献综述
时间: 2023-08-27 21:35:23 浏览: 126
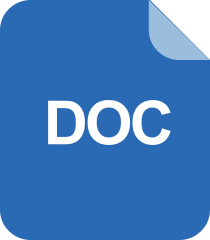
文献综述的写法.doc
Python是一种高级编程语言,已经成为了数据科学和人工智能领域的标准语言。Python的语法是非常简单易懂的,使得初学者也能够很快上手。本文将介绍Python的语法,包括语句、模块、函数、变量和数据类型、控制流语句、面向对象编程、异常处理、文件操作和正则表达式。
一、语句
Python中的语句是由一行或多行代码组成的。Python中的语句不需要使用分号(;)来分隔,而是使用换行符。例如:
```
print("Hello, world!")
```
Python中的注释使用#号。例如:
```
# This is a comment
```
二、模块
Python中的模块是一个包含函数、类和变量的文件。可以使用import关键字导入模块。例如:
```
import math
print(math.sqrt(4))
```
可以使用from...import语句导入模块中的函数、类和变量。例如:
```
from math import sqrt
print(sqrt(4))
```
三、函数
Python中的函数使用def关键字定义。例如:
```
def add(a, b):
return a + b
```
Python中的函数可以有默认参数值和不定长参数。例如:
```
def say_hello(name="world"):
print("Hello, " + name + "!")
say_hello()
say_hello("Alice")
def add(*args):
return sum(args)
print(add(1, 2, 3))
```
四、变量和数据类型
Python是一种动态类型语言,也就是说,变量的数据类型不需要预先声明。在Python中,变量名就是变量的声明。例如:x = 5。Python支持多种数据类型,包括整数、浮点数、字符串、布尔值、列表、元组和字典。
五、控制流语句
Python中的控制流语句包括条件语句和循环语句。条件语句使用if和else关键字。例如:
```
if x > 0:
print("x is positive")
else:
print("x is not positive")
```
循环语句包括while和for循环。例如:
```
while x < 10:
x += 1
print(x)
for i in range(10):
print(i)
```
六、面向对象编程
Python是一种面向对象的语言。使用class关键字定义类,使用self关键字引用当前实例。例如:
```
class Person:
def __init__(self, name):
self.name = name
def say_hello(self):
print("Hello, my name is " + self.name)
person = Person("Alice")
person.say_hello()
```
七、异常处理
Python中的异常处理使用try和except关键字。例如:
```
try:
x = int(input("Enter a number: "))
print("You entered " + str(x))
except ValueError:
print("That's not a number!")
```
八、文件操作
Python中的文件操作使用open函数打开文件,使用read和write方法读写文件。例如:
```
file = open("example.txt", "w")
file.write("Hello, world!")
file.close()
file = open("example.txt", "r")
print(file.read())
file.close()
```
九、正则表达式
Python中的正则表达式使用re模块。例如:
```
import re
pattern = r"([a-zA-Z]+) (\d+)"
text = "June 24, August 9, Dec 12"
match = re.search(pattern, text)
if match:
print(match.group(0))
print(match.group(1))
print(match.group(2))
```
结论
本文介绍了Python的语法,包括语句、模块、函数、变量和数据类型、控制流语句、面向对象编程、异常处理、文件操作和正则表达式。这些语法是Python编程的基础,掌握它们对于编写高质量的Python代码至关重要。
阅读全文
相关推荐
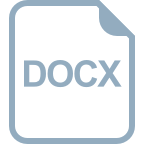
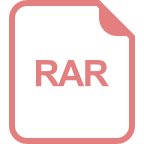
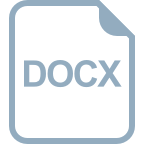
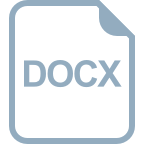
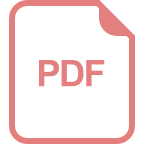
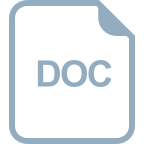
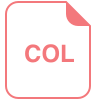
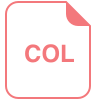
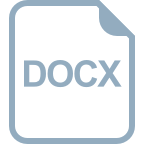
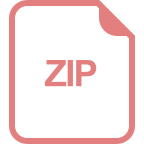
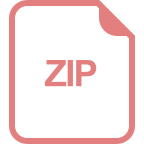
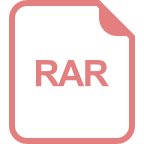
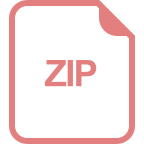
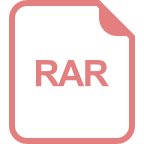