resttemplate form表单提交
时间: 2023-04-27 21:03:33 浏览: 971
RestTemplate是Spring框架中的一个HTTP客户端工具,可以用于发送HTTP请求。其中,form表单提交是一种常见的HTTP请求方式,可以使用RestTemplate来实现。
具体实现步骤如下:
1. 创建RestTemplate对象。
2. 创建MultiValueMap对象,用于存储表单数据。
3. 使用RestTemplate的postForObject方法发送POST请求,其中第一个参数为请求URL,第二个参数为表单数据,第三个参数为返回类型。
示例代码如下:
```
RestTemplate restTemplate = new RestTemplate();
MultiValueMap<String, String> formData = new LinkedMultiValueMap<>();
formData.add("username", "test");
formData.add("password", "123456");
String result = restTemplate.postForObject("http://example.com/login", formData, String.class);
```
以上代码实现了向http://example.com/login发送一个POST请求,请求参数为username=test&password=123456的表单数据,并返回响应结果。
相关问题
jmeter form表单提交
JMeter是一个功能强大的性能测试工具,可以用于模拟多种场景下的负载测试。在JMeter中,可以使用HTTP请求来模拟提交表单的操作。
要使用JMeter进行form表单提交,可以按照以下步骤进行操作:
1. 添加线程组:在测试计划中添加一个线程组,用于定义并发用户数、循环次数等参数。
2. 添加HTTP请求:在线程组下添加一个HTTP请求,默认使用HTTP请求默认值配置元件。
3. 配置HTTP请求:在HTTP请求中,设置服务器名称或IP地址、端口号、路径等信息。选择请求方法为POST,并勾选"Use multipart/form-data for POST"选项。
4. 添加参数:在HTTP请求中添加需要提交的参数。可以使用HTTP请求默认值配置元件来设置公共参数,也可以在HTTP请求中直接添加参数。
5. 添加监听器:为了查看测试结果,可以添加一个监听器,如查看结果树、聚合报告等。
6. 运行测试计划:保存并运行测试计划,JMeter会模拟多个用户同时提交表单,并记录响应时间、吞吐量等性能指标。
需要注意的是,具体的form表单提交方式可能因网站的实现方式而有所不同。有些网站可能使用了特殊的加密方式或者需要额外的认证信息,这时需要根据具体情况进行配置。
elementui form 表单提交
elementui form表单提交可以通过以下步骤实现:
1. 在HTML文件中,使用`<el-form>`标签包裹需要提交的表单元素。
2. 在`<el-form>`标签中,使用`ref`属性给表单设置一个唯一的引用名称,例如`ref="formName"`。
3. 在表单元素中,使用`v-model`指令绑定表单输入的值到一个Vue实例的数据属性上。
4. 在`<el-form>`标签中,使用`@submit`事件监听表单的提交动作。
5. 在Vue实例的方法中,通过表单引用名称获取表单实例,然后调用`validate()`方法进行表单验证。
6. 在`validate()`方法的回调函数中,判断表单验证结果是否通过。
7. 如果验证通过,可以在回调函数中执行表单提交的操作。
相关推荐
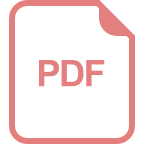
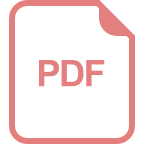












