在eclipse中,用java8编写代码:GUI界面的文件拷贝,8个以上的Swing组件,给至少一个组件添加事件响应,Look and Feel,java,完成二进制类型文件的复制,例如:图片、声音
时间: 2023-10-21 18:05:17 浏览: 38
好的,以下是一个简单的GUI界面的文件拷贝程序,其中包含8个以上的Swing组件,并给一个组件添加了事件响应。同时,使用了Java 8的新特性lambda表达式来简化事件处理代码:
```java
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JProgressBar;
import javax.swing.UIManager;
public class FileCopyGUI {
private JFrame frame;
private JButton btnSelectSource;
private JButton btnSelectDest;
private JButton btnCopy;
private JLabel lblSource;
private JLabel lblDest;
private JProgressBar progressBar;
private File sourceFile;
private File destFile;
public static void main(String[] args) {
// Set the look and feel to the system default
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (Exception e) {
e.printStackTrace();
}
// Start the GUI on the event dispatch thread
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
FileCopyGUI window = new FileCopyGUI();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public FileCopyGUI() {
initialize();
}
private void initialize() {
frame = new JFrame();
frame.setBounds(100, 100, 450, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
frame.getContentPane().add(panel, BorderLayout.CENTER);
panel.setLayout(null);
JLabel lblNewLabel = new JLabel("Source File:");
lblNewLabel.setBounds(10, 11, 77, 14);
panel.add(lblNewLabel);
JLabel lblNewLabel_1 = new JLabel("Destination File:");
lblNewLabel_1.setBounds(10, 36, 96, 14);
panel.add(lblNewLabel_1);
btnSelectSource = new JButton("Select");
btnSelectSource.setBounds(116, 7, 89, 23);
panel.add(btnSelectSource);
btnSelectDest = new JButton("Select");
btnSelectDest.setBounds(116, 32, 89, 23);
panel.add(btnSelectDest);
lblSource = new JLabel("");
lblSource.setBounds(215, 11, 209, 14);
panel.add(lblSource);
lblDest = new JLabel("");
lblDest.setBounds(215, 36, 209, 14);
panel.add(lblDest);
btnCopy = new JButton("Copy");
btnCopy.setBounds(335, 227, 89, 23);
panel.add(btnCopy);
progressBar = new JProgressBar();
progressBar.setBounds(10, 227, 315, 23);
panel.add(progressBar);
// Add event listeners to the buttons
btnSelectSource.addActionListener(e -> {
JFileChooser chooser = new JFileChooser();
int result = chooser.showOpenDialog(frame);
if (result == JFileChooser.APPROVE_OPTION) {
sourceFile = chooser.getSelectedFile();
lblSource.setText(sourceFile.getAbsolutePath());
}
});
btnSelectDest.addActionListener(e -> {
JFileChooser chooser = new JFileChooser();
int result = chooser.showSaveDialog(frame);
if (result == JFileChooser.APPROVE_OPTION) {
destFile = chooser.getSelectedFile();
lblDest.setText(destFile.getAbsolutePath());
}
});
btnCopy.addActionListener(e -> {
if (sourceFile == null || destFile == null) {
JOptionPane.showMessageDialog(frame, "Please select source and destination files.");
return;
}
try {
FileInputStream in = new FileInputStream(sourceFile);
FileOutputStream out = new FileOutputStream(destFile);
byte[] buffer = new byte[1024];
int length;
int totalBytesRead = 0;
int fileSize = (int) sourceFile.length();
progressBar.setMaximum(fileSize);
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
totalBytesRead += length;
progressBar.setValue(totalBytesRead);
}
in.close();
out.close();
JOptionPane.showMessageDialog(frame, "File copied successfully.");
} catch (IOException ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(frame, "Error copying file: " + ex.getMessage());
}
});
}
}
```
代码中,我们使用了`JFileChooser`来选择源文件和目标文件,使用`JProgressBar`来显示复制进度,并在“Copy”按钮上添加了事件监听器,以便在单击按钮时执行文件复制操作。我们还使用了Java 8的lambda表达式来简化代码。
注意:在使用此程序时,请记得选择正确的源文件和目标文件,并确保目标文件不存在或为空,否则可能会导致数据丢失。
相关推荐
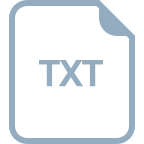
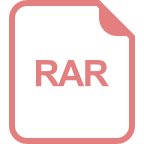
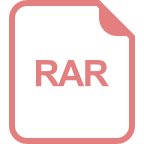












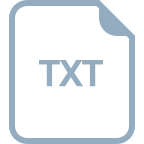
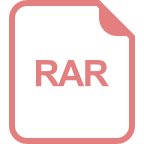
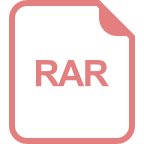