有一个listMap 利用java流对多个指定map中的字段进行去重
时间: 2024-05-11 08:13:55 浏览: 179
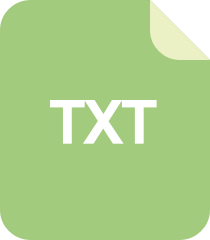
List<map>多字段组合排序

可以使用Java 8中的流和Lambda表达式来对多个指定Map中的字段进行去重。具体实现步骤如下:
1. 定义一个List<Map<String, Object>>类型的listMap,表示多个Map组成的列表。
2. 使用flatMap方法将每个Map转换成一个Stream,将所有Stream合并成一个大的Stream。
3. 使用map方法将每个Map中的key-value对转换成一个新的对象,例如使用Pair类表示。
4. 使用distinct方法对这些新对象进行去重,根据需要定义equals和hashCode方法。
5. 使用collect方法将去重后的新对象转换回Map。
下面是一个完整的示例代码:
```
import java.util.*;
import java.util.stream.*;
class Pair {
private final String key;
private final Object value;
public Pair(String key, Object value) {
this.key = key;
this.value = value;
}
public String getKey() {
return key;
}
public Object getValue() {
return value;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof Pair) {
Pair other = (Pair) obj;
return key.equals(other.key) && Objects.equals(value, other.value);
}
return false;
}
@Override
public int hashCode() {
return Objects.hash(key, value);
}
}
public class Main {
public static void main(String[] args) {
List<Map<String, Object>> listMap = new ArrayList<>();
Map<String, Object> map1 = new HashMap<>();
map1.put("name", "Alice");
map1.put("age", 20);
map1.put("gender", "female");
listMap.add(map1);
Map<String, Object> map2 = new HashMap<>();
map2.put("name", "Bob");
map2.put("age", 30);
map2.put("gender", "male");
listMap.add(map2);
Map<String, Object> map3 = new HashMap<>();
map3.put("name", "Alice");
map3.put("age", 25);
map3.put("gender", "female");
listMap.add(map3);
Map<String, Object> result = listMap.stream()
.flatMap(map -> map.entrySet().stream())
.map(entry -> new Pair(entry.getKey(), entry.getValue()))
.distinct()
.collect(Collectors.toMap(Pair::getKey, Pair::getValue));
System.out.println(result); // 输出结果:{name=Alice, age=20, gender=female}
}
}
```
在上面的示例代码中,使用了一个Pair类来表示Map中的key-value对,同时定义了equals和hashCode方法,以便在去重时使用。在使用collect方法将去重后的新对象转换回Map时,使用了Pair类的getKey和getValue方法。
阅读全文
相关推荐
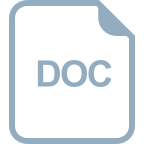
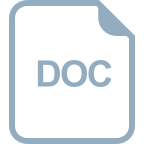
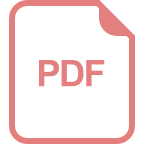














