package util; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Util { private static final String URL = "jdbc:mysql://localhost:3306/_library"; private static final String USERNAME = "root"; private static final String PASSWORD = "root"; public static Connection getConnection() { try { return DriverManager.getConnection(URL, USERNAME, PASSWORD); } catch (SQLException e) { System.out.println("无法建立数据库连接:" + e.getMessage()); return null; } } }
时间: 2024-01-29 13:02:32 浏览: 30
看起来你的代码片段是 Java 语言编写的,用于获取数据库连接。这个错误通常是由于找不到特定的类而引起的。具体来说,这个错误可能是由于以下原因之一导致的:
1. 缺少依赖库:你需要确保你的项目中包含了正确的依赖库,比如 MySQL 的 JDBC 驱动程序。如果你使用的是 Maven 或 Gradle 等构建工具,则需要在你的项目配置文件中添加相应的依赖项。
2. 类名错误:你需要确保你的类名和包名都正确。在你的代码片段中,包名是 `util`,类名是 `Util`。如果你在其他地方使用了这个类,需要确保你的引用是正确的。
3. 编译错误:你需要确保你的代码能够成功编译。如果你在编译时遇到了错误,则需要解决这些错误。
如果你可以提供更多的上下文信息,比如完整的错误信息和调用堆栈跟踪,我将能够更好地帮助你解决这个问题。
相关问题
package connectmysql; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; import java.util.Scanner; import java.sql.SQLException; import java.sql.Statement; public class text { private final static String url ="jdbc:mysql://localhost:3306/mytextsql?severTimezone=GMT"; private final static String username="root"; private final static String password="yxh9497"; public static void main(String args[]) { try { Class.forName("com.mysql.cj.jdbc.Driver"); }catch(Exception e) { e.printStackTrace(); } try { Connection connect=DriverManager.getConnection(url,username,password); Statement stmt=connect.createStatement(); Scanner a=new Scanner(System.in); System.out.print("删除的是:"+'\n'); String a4=a.next(); String sql="delete from user where name="+a4; int i=stmt.executeUpdate(sql); if(i > 0){ System.out.println("删除成功"); } }catch(Exception e) { e.printStackTrace(); } } }
看起来您的代码是在使用Java连接MySQL数据库,并尝试删除一个名为`user`的表中的行。但是,这段代码存在一些潜在的问题。
首先,您缺少`import java.sql.SQLException`语句,因此编译器可能会报告找不到该类的错误。因此,您需要在代码顶部添加此导入语句。
其次,您在SQL语句中使用了字符串变量`a4`,但没有将其用引号括起来。这可能导致SQL语句无法正确解析,从而导致删除操作失败。因此,您需要将`a4`变量用引号括起来,以确保SQL语句正确。
最后,您使用了两个`Statement`对象,这应该会导致编译错误。您需要删除其中一个`import java.sql.Statement`语句,并将`stmt`对象的声明放置在`try`块中。
下面是修改后的代码:
```
package connectmysql;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.Scanner;
import java.sql.SQLException;
public class text {
private final static String url ="jdbc:mysql://localhost:3306/mytextsql?severTimezone=GMT";
private final static String username="root";
private final static String password="yxh9497";
public static void main(String args[]) {
try {
Class.forName("com.mysql.cj.jdbc.Driver");
}catch(Exception e) {
e.printStackTrace();
}
try {
Connection connect=DriverManager.getConnection(url,username,password);
Statement stmt=connect.createStatement();
Scanner a=new Scanner(System.in);
System.out.print("删除的是:"+'\n');
String a4=a.next();
String sql="delete from user where name='"+a4+"'";
int i=stmt.executeUpdate(sql);
if(i > 0){
System.out.println("删除成功");
}
}catch(SQLException e) {
e.printStackTrace();
}
}
}
```
希望这可以帮助您解决问题。
package jar; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class DBUtil { public static Connection getConnection() { Connection conn=null; try { Class.forName("com.mysql.cj.jdbc.Driver"); String url="jdbc:mysql://localhost:3306/studenttest"; conn=DriverManager.getConnection(url, "root", "root"); } catch (ClassNotFoundException e) { System.out.println("驱动找不到"); e.printStackTrace(); } catch (SQLException e) { System.out.println("数据库连接不成功"); e.printStackTrace(); } System.out.println("数据库连接成功!"); return conn; } public static void main(String[] args) { DBUtil db = new DBUtil(); db.getConnection(); } }哪里需要改进
它。在接收到数据时,它将其附加到接收文本框中。在发送数据时,它将份代码看起来没什么问题,但是有以下几点可以改进:
1. getConnection()方法可以设置为静态方法,这样就不需要每次都实例化一个对象来调用getConnection()方法了。
2. getConnection()方法中的数据库连接信息可以通过配置文件读取,这样就可以在不同环境下方便地修改数据库连接信息。
3. getConnection()方法中的异常处理可以更加具体,比如可以区分ClassNotFoundException和SQLException,以便更好地定位问题。
4. getConnection()方法中可以加入连接池的支持,以提高程序的性能和稳定性。
5. getConnection()方法中可以加入日志输出,以便更好地追踪和排查问题。
6. getConnection()方法中可以加入异常捕获,以确保资源的正确释放,避免资源泄露或者异常退出的情况。
综上所述,可以对代码进行如下改进:
```
package jar;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.Properties;
public class DBUtil {
private static String url;
private static String user;
private static String password;
private static String driver;
static {
try {
// 加载配置文件
InputStream in = DBUtil.class.getClassLoader().getResourceAsStream("db.properties");
Properties prop = new Properties();
prop.load(in);
// 读取配置信息
url = prop.getProperty("url");
user = prop.getProperty("user");
password = prop.getProperty("password");
driver = prop.getProperty("driver");
// 加载驱动
Class.forName(driver);
} catch (IOException | ClassNotFoundException e) {
// 日志输出
e.printStackTrace();
throw new RuntimeException("加载数据库配置信息失败!");
}
}
public static Connection getConnection() {
Connection conn = null;
try {
conn = DriverManager.getConnection(url, user, password);
} catch (SQLException e) {
// 日志输出
e.printStackTrace();
throw new RuntimeException("获取数据库连接失败!");
}
return conn;
}
public static void close(Connection conn) {
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
// 日志输出
e.printStackTrace();
}
}
}
public static void main(String[] args) {
Connection conn = DBUtil.getConnection();
System.out.println("数据库连接成功!");
DBUtil.close(conn);
}
}
```
在改进后的代码中,getConnection()方法中的数据库连接信息通过配置文件读取,同时加载驱动和异常处理等操作也都进行了改进。此外,还新增了close()方法来释放资源。
相关推荐
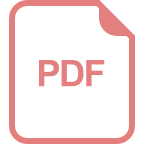
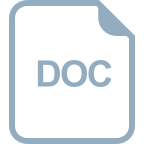
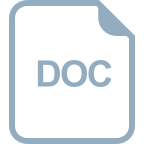












