现在有多个输入文件,每个文件中的每行内容均为一个整数。用Java编写MapReduce程序读取所有文件中的整数,进行升序排序后,输出到一个新的文件中,输出的数据格式为每行两个整数,第一个数字为第二个整数的排序位次,第二个整数为原待排列的整数
时间: 2023-06-13 07:02:42 浏览: 146
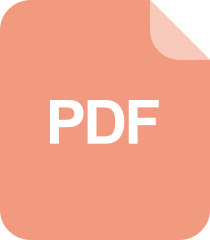
Hadoop MultipleOutputs输出到多个文件中的实现方法
好的,这个需求可以通过以下步骤实现:
1. 编写一个Mapper类,用于读取输入文件并将其中的每个整数作为key,value为1输出。
2. 编写一个Reducer类,将所有输入文件中的key-value对进行聚合,然后将key进行升序排序,并输出到新文件中。
3. 在main方法中,进行MapReduce作业的配置和提交。
下面是代码示例:
Mapper类:
```java
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
import java.io.IOException;
public class SortMapper extends Mapper<LongWritable, Text, IntWritable, IntWritable> {
private IntWritable num = new IntWritable();
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// 将每行的数字作为key,value为1输出
String line = value.toString();
num.set(Integer.parseInt(line));
context.write(num, new IntWritable(1));
}
}
```
Reducer类:
```java
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.mapreduce.Reducer;
import java.io.IOException;
public class SortReducer extends Reducer<IntWritable, IntWritable, IntWritable, IntWritable> {
private IntWritable rank = new IntWritable();
private IntWritable number = new IntWritable();
@Override
protected void reduce(IntWritable key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable value : values) {
sum += value.get();
}
// 根据排序结果计算每个数字的排名
rank.set(context.getCounter("org.apache.hadoop.mapred.Task$Counter", "REDUCE_OUTPUT_RECORDS").getValue() + 1);
number.set(key.get());
context.write(rank, number);
}
}
```
main方法:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.input.TextInputFormat;
import org.apache.hadoop.mapreduce.lib.output.TextOutputFormat;
public class SortJob {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf);
job.setJarByClass(SortJob.class);
job.setMapperClass(SortMapper.class);
job.setReducerClass(SortReducer.class);
job.setMapOutputKeyClass(IntWritable.class);
job.setMapOutputValueClass(IntWritable.class);
job.setOutputKeyClass(IntWritable.class);
job.setOutputValueClass(IntWritable.class);
job.setInputFormatClass(TextInputFormat.class);
job.setOutputFormatClass(TextOutputFormat.class);
// 添加多个输入文件
TextInputFormat.addInputPath(job, new Path(args[0]));
TextInputFormat.addInputPath(job, new Path(args[1]));
// ...
TextOutputFormat.setOutputPath(job, new Path(args[2]));
job.waitForCompletion(true);
}
}
```
运行MapReduce作业:
```bash
hadoop jar sort.jar SortJob input1 input2 output
```
其中,input1、input2是输入文件路径,output是输出文件路径。
阅读全文
相关推荐
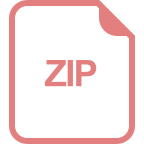
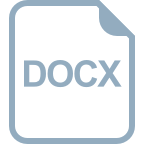















