android 加密源文件示例
时间: 2023-10-31 11:59:17 浏览: 35
以下是一个简单的 Android 加密和解密文件的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
public class FileEncryptor {
private static final String ALGORITHM = "AES/CBC/PKCS5Padding";
private static final String SECRET_KEY = "mySecretKey12345";
private static final String IV_PARAMETER = "myIVParameter123";
public static void encrypt(File inputFile, File outputFile) throws Exception {
SecretKey secretKey = new SecretKeySpec(SECRET_KEY.getBytes(), "AES");
IvParameterSpec ivParameterSpec = new IvParameterSpec(IV_PARAMETER.getBytes());
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey, ivParameterSpec);
FileInputStream inputStream = new FileInputStream(inputFile);
FileOutputStream outputStream = new FileOutputStream(outputFile);
byte[] inputBytes = new byte[(int) inputFile.length()];
inputStream.read(inputBytes);
byte[] outputBytes = cipher.doFinal(inputBytes);
outputStream.write(outputBytes);
inputStream.close();
outputStream.close();
}
public static void decrypt(File inputFile, File outputFile) throws Exception {
SecretKey secretKey = new SecretKeySpec(SECRET_KEY.getBytes(), "AES");
IvParameterSpec ivParameterSpec = new IvParameterSpec(IV_PARAMETER.getBytes());
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKey, ivParameterSpec);
FileInputStream inputStream = new FileInputStream(inputFile);
FileOutputStream outputStream = new FileOutputStream(outputFile);
byte[] inputBytes = new byte[(int) inputFile.length()];
inputStream.read(inputBytes);
byte[] outputBytes = cipher.doFinal(inputBytes);
outputStream.write(outputBytes);
inputStream.close();
outputStream.close();
}
}
```
在此示例中,我们使用 AES 算法进行加密和解密,并使用 CBC 模式和 PKCS5Padding 填充。我们使用相同的密钥和 IV 参数来加密和解密文件。
要使用此类对文件进行加密和解密,可以按照以下步骤操作:
```java
File inputFile = new File("/path/to/input/file");
File encryptedFile = new File("/path/to/encrypted/file");
File decryptedFile = new File("/path/to/decrypted/file");
// 加密文件
FileEncryptor.encrypt(inputFile, encryptedFile);
// 解密文件
FileEncryptor.decrypt(encryptedFile, decryptedFile);
```
请注意,此示例仅适用于小文件。如果要加密或解密大型文件,请使用流来处理文件内容。
相关推荐
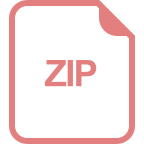
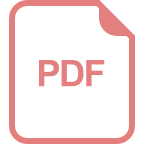














